Use APIs in conjunction with promotions and discounts
Describes the required steps to apply overrides to accomplish promotion-related scenarios in Optimizely Configured Commerce.
This article provides the required steps to apply overrides to accomplish two promotion-related scenarios:
- Retrieve the promotions that are applied to a given line item in the order.
- Provide information about which promotions were applied to the order AND also report which promotions were order level vs. order line level.
The following table shows the default behavior of the APIs related to promotions:
GET | /api/v1/carts/{cartId}/promotions | Return a collection of promotions applied to a specific shopping cart |
POST | /api/v1/carts/{cartId}/promotions | Apply promotions to a specific shopping cart |
GET | /api/v1/carts/{cartId}/promotions/{promotionId} | Return a single promotion for a specific shopping cart |
Retrieving the Cart Line Level Data
- Create a custom mapper class that inherits from the GetCartLineMapper base class.
- Override the MapResult method. Be sure to call the base method before doing any work.
- The GetCartLineResult object is passed into the MapResult method. This object contains the CartLine object as a property, which contains a PromotionResult property and a Promotion property. Add any additional information you need to the Properties collection of the GetCartLineModel object.
The following mapper adds the Promotion "Name" to the Properties collection.
public class CustomGetCartLineMapper : GetCartLineMapper
{
public CustomGetCartLineMapper(ICurrencyFormatProvider currencyFormatProvider, IObjectToObjectMapper objectToObjectMapper, IUrlHelper urlHelper, IRouteDataProvider routeDataProvider)
: base(currencyFormatProvider, objectToObjectMapper, urlHelper, routeDataProvider)
{
}
public override CartLineModel MapResult(GetCartLineResult serviceResult, HttpRequestMessage request)
{
var result = base.MapResult(serviceResult, request);
result.Properties.Add("promotionName", serviceResult.CartLine.PromotionResult?.Promotion.Name);
return result;
}
}
```
The image below shows the new, updated response with the "promotionName" custom property.
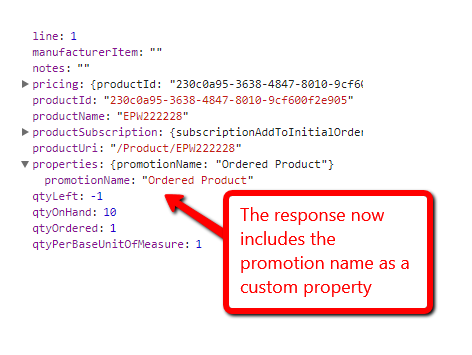
### Using the Promotions API
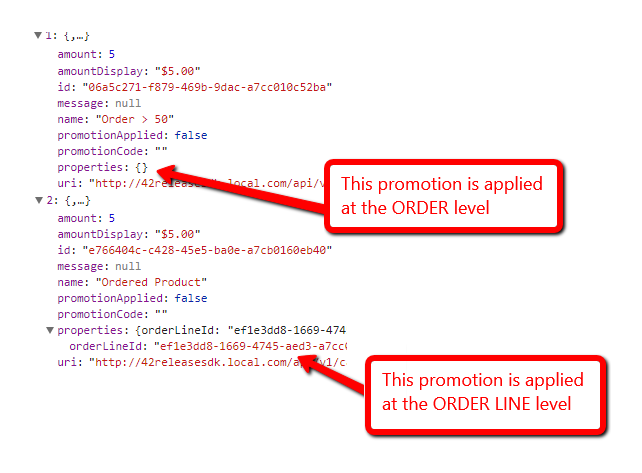
The image below shows the new, updated response with the "promotionName" custom property.
This solution is very similar to the solution used in <<product-name>> 4.3 to indicate promotions at the order line or order level.
```prettyprint
public class CustomGetPromotionMapper : GetPromotionMapper
{
protected readonly ICartService CartService;
public CustomGetPromotionMapper(IObjectToObjectMapper objectToObjectMapper, IUrlHelper urlHelper, ICartService cartService)
: base(objectToObjectMapper, urlHelper)
{
this.CartService = cartService;
}
public override PromotionModel MapResult(GetPromotionResult serviceResult, HttpRequestMessage request)
{
var result = base.MapResult(serviceResult, request);
var cartId = request.GetRouteData().Values["cartid"].ToString();
var cart = this.CartService.GetCart(new GetCartParameter
{
CartId = cartId.ToCurrentOrGuid(),
GetCartLineResults = true
});
foreach (var cartLine in cart.CartLineResults.Select(o => o.CartLine))
{
if (cartLine.PromotionResult?.Promotion.Id == serviceResult.Promotion.Id)
{
result.Properties.Add("orderLineId", cartLine.Id.ToString());
}
}
return result;
}
}
```
The following custom mapper adds the matching "orderLineId" to the promotion response. This indicates that the promotion applies to the cart line level. If this custom property does not exist in the response, this indicates that the promotion applies to the cart level.
1. Create a custom mapper class, but this time inherit from the **GetPromotionMapper** base class.
2. Again, override the **MapResult** method and be sure to call the base method before doing any work.
3. In this method, you can access the **cartid** from the incoming request.
```prettyprint
var cartId = request.GetRouteData().Values["cartid"].ToString(); ```
4. Using the **cartid** you can access the current cart.
5. Then, just like before, you can add any additional information you need to the **Properties** collection of the **PromotionModel**.
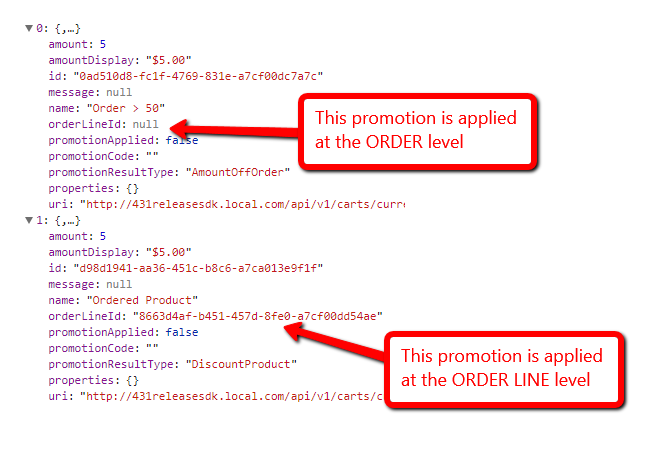
The image below shows a response with the both a cart and order line level promotion applied. The first promotion object contains an "orderLineId" property that has a null value. This indicates that the promotion was applied at the order level. In the second promotion object, the "orderLineId" property contains the id of the matching order line to which the promotion was applied.
The endpoint `/api/v1/carts/current` returns order line data alongside the promotion data. This can help determine which promotions are applied at the order or order line level.
Updated about 1 year ago