IBuildEmailValues
Describes how the build email values object is responsible for building a list of recipients for various emails.
Methods
List<string> BuildOrderConfirmationEmailToList(Guid customerOrderId);
Builds a list of email recipients for an order confirmation email.
customerOrderId
– The id of the customer order for which the order confirmation email will be sent.
List<string> BuildQuoteEmailToList(Guid customerOrderId);
Builds a list of email recipients for an email sent during the RFQ process. When are emails sent and to whom?
customerOrderId
– The id of the customer order for which the order confirmation email will be sent.
List<string> BuildShipmentEmailToList(Guid shipmentId);
Builds a list of recipients for an email sent when a shipment has been shipped.
customerOrderId
– The id of the customer order for which the order confirmation email will be sent.
Remarks
Configured Commerce provides a single implementation. This implementation uses settings when choosing some of the recipients for the emails. When you provide a new implementation, your new implementation will be used instead of the default implementation.
Order confirmation email
Recipients for the order confirmation email include the email addresses configured in the Admin Console using the Site Configurations > Emails > Order Notification Email Address setting.
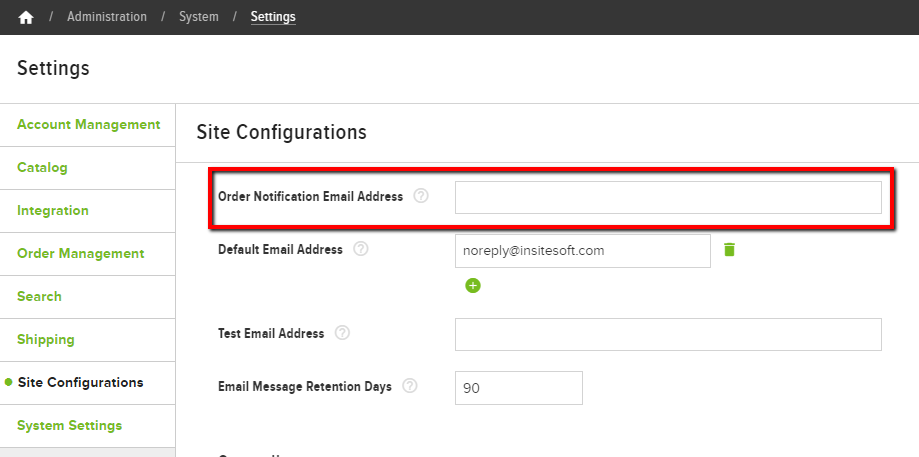
The user who placed the order and the bill-to and ship-to customers for whom the order was placed can also be included as recipients. These recipients can be configured in the Admin Console using the Order Management > Checkout > Send Order Confirmation To setting.
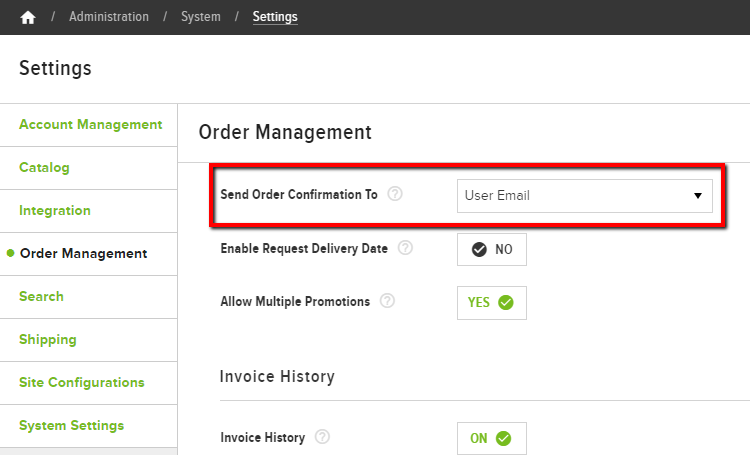
Quote emails
Recipients for the quote emails include the bill-to and ship-to customer for whom the order will be placed. You can specify additional recipients for these emails in the Admin Console using the Site Configurations > Emails > Order Notification Email Address setting.
Shipment emails
Recipients for the shipment email include the user who placed the order, the bill-to and ship-to customers for whom the order was placed and the salesperson associated with the order that is being shipped. You can specify additional recipients for these emails in the Admin Console using the Site Configurations > Emails > Customer Service Email Address setting.
Example: Build list of recipients for order confirmation email
The code sample below shows the handler that uses the IBuildEmailValues dependency to build a list of recipients and send an order confirmation email to those recipients.
[DependencyName(nameof(SendConfirmationEmail))]
public sealed class SendConfirmationEmail : HandlerBase<UpdateCartParameter, UpdateCartResult>
{
public override int Order => 3200;
private readonly Lazy<IBuildEmailValues> buildEmailValues;
private readonly Lazy<IEmailService> emailService;
public SendConfirmationEmail(Lazy<IBuildEmailValues> buildEmailValues, Lazy<IEmailService> emailService)
{
this.buildEmailValues = buildEmailValues;
this.emailService = emailService;
}
public override UpdateCartResult Execute(IUnitOfWork unitOfWork, UpdateCartParameter parameter, UpdateCartResult result)
{
if (!parameter.Status.EqualsIgnoreCase(CustomerOrder.StatusType.Submitted))
{
return this.NextHandler.Execute(unitOfWork, parameter, result);
}
var emailList = unitOfWork.GetTypedRepository<IEmailListRepository>().GetOrCreateByName("OrderConfirmation", "Order Confirmation");
var toAddresses = this.buildEmailValues.Value.BuildOrderConfirmationEmailToList(result.GetCartResult.Cart.Id);
this.emailService.Value.SendEmailList(emailList.Id, toAddresses, result.ConfirmationEmailModel, null, unitOfWork, SiteContext.Current.WebsiteDto?.Id);
return this.NextHandler.Execute(unitOfWork, parameter, result);
}
}
Updated over 1 year ago