Property attributes
Describes attributes available for properties in Optimizely Content Management System (CMS).
Properties are the fields in content, such as pages and blocks, where editors enter information. You can apply different attributes to properties, to describe their purpose and how they should behave.
Consider the following when working with properties:
- Use an intuitive naming of properties in code, and set a "friendly" display name and description, to help editors understand the purpose of a property (attributes
Name
,Description
). - Organize related properties under the same tab in edit view, and in logical order with the most frequently used ones at the top (attributes
GroupName
andOrder
). - Consider adding field value validation, default values, or required fields, where applicable.
Available attributes
The following attributes are located in the EPiServer.DataAnnotations
namespace.
BackingType(Type)
– Defines thePropertyData
type used to store values for this property. Must be a type inheriting fromPropertyData
. The backing type is auto-determined. See Default Backing Types.CultureSpecific(bool)
– Defines if this property should have a different value for each language. Properties will not be culture-specific.Searchable(bool)
– Defines if the property value should be searchable. String properties are searchable, other property types are not.Ignore
– CMS ignores property and will not be backed by aPropertyData
type. (When CMS ignores the property, it is technically possible to assign the property even though the content is read-only. To avoid that, implement a setter that checks theIsReadOnly
property before assigning fields. Content is stored as read-only singletons in the cache so it is impossible to use ignored properties for assigning state related to a single request, as that would cause serious concurrency issues.) Property is not ignored.AllowedTypes
– Allows or restricts certain items to be added to the property. This attribute only works withContentArea
,ContentReference
, andContentReferenceList
type properties. See Restricting content types in properties. By default, items can be added to the property.
The following attributes are located in the System.ComponentModel.DataAnnotations
namespace.
Required
– Defines if a value for this property must be set before being able to save a page of the parent type. Property value is not required.ScaffoldColumn(bool)
– Defines if this property is visible in edit view. The property is visible in edit view.Display(Name=...
,Description=...
,GroupName=...
,Order=...
,Prompt=...)
– TheName
,Description
,GroupName
,Order
, andPrompt
properties are used to set theEditCaption
,HelpText
,Tab
,FieldOrder
, and placeholder or watermark text, respectively.EditCaption
is set to the name of the property.HelpText
is NULL. Theprompt
is NULL.UIHint
– Used to select editor or renderer or both by defining a hint string. You can useEPiServer.Web.UIHint
to add hints for known types in the system, for instanceUIHint.Image
. The default editor and renderer for the type is used.StringLength
– Sets a maximum length for strings. Note that this attribute cannot be used for properties of typeXhtmlString
. No length restriction.RegularExpression
– Validates the input format. Usually used for string properties. No validation of the input.Range
– Determines the valid range for numeric properties. No validation of range except the minimum/maximum values for the value type (For instanceInt32.MinValue
andInt32.MaxValue
).Editable
– Indicates whether a property is editable. The property is editable.
The following attribute is located in the EPiServer.Cms.Shell.UI.ObjectEditing
namespace.
ReloadOnChange
– Defines if the whole editing context (i.e. OPE or Forms View) should be reloaded on every value change.
This attribute is useful when dealing with dependent properties where one property depends on the value of one or more other properties from the same model.
Note that this attribute does not work in Quick Edit dialog because there is no Autosave in the Quick Edit dialog box.
This attribute does not work when creating a content instance because there is nothing to refresh yet (the content item has not been created yet). The context will not be reloaded on value change.
The following image shows how the attributes are mapped to settings in the admin view:
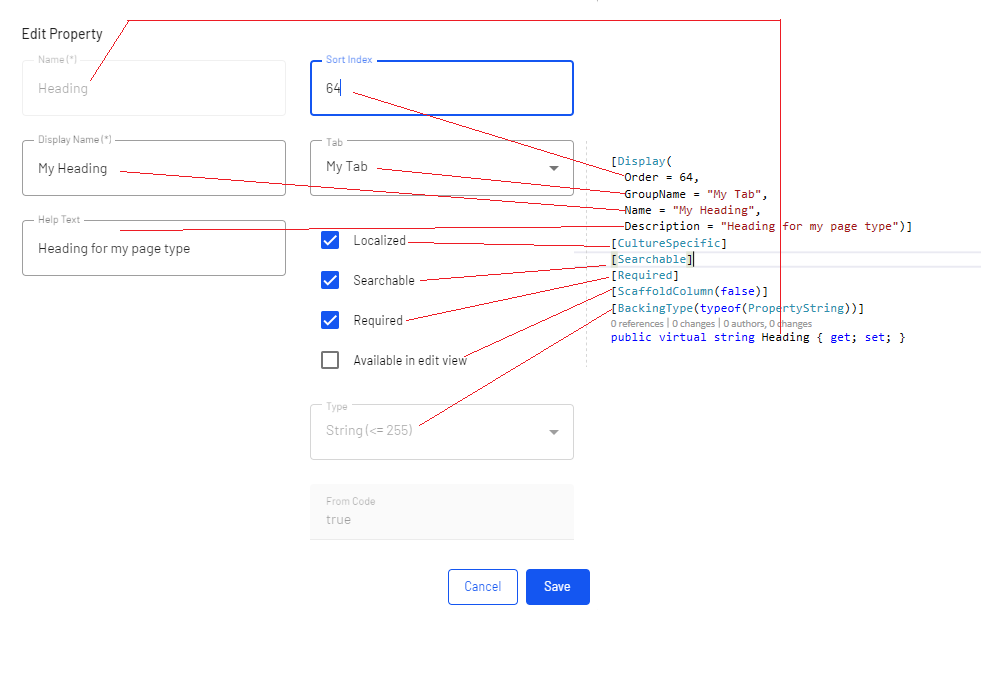
Default backing types
If the BackingType
attribute has not been set for a property, the backing type is automatically assigned to a PropertyDefinitionType
where the corresponding EPiServer.Core.PropertyData.PropertyValueType
matches the property type. That means that if there is a PropertyData
implementation (can be a custom property) with matching PropertyValueType
that definition is used. Otherwise, the backing type is assigned according to this table.
See Built-in property types for details on using the correct type.
Property type | BackingType |
---|---|
ContentArea | PropertyContentArea |
Boolean | PropertyBoolean |
CategoryList | PropertyCategory |
DateTime | PropertyDate |
Double | PropertyFloatNumber |
LinkItemCollection | PropertyLinkCollection |
Int32 | PropertyNumber |
PageType | PropertyPageType |
String | PropertyLongString |
TimeSpan | PropertyTimeSpan |
Url | PropertyUrl |
XhtmlString | PropertyXhtmlString |
Blob | PropertyBlob |
IList<ContentReference> | PropertyContentReferenceList |
IList<T> | PropertyCollection<T> |
Examples
Sample code using many of the attributes described in this article.
using EPiServer;
using EPiServer.Core;
using EPiServer.DataAbstraction;
using EPiServer.DataAnnotations;
using EPiServer.Shell.ObjectEditing;
using EPiServer.Web;
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
namespace CodeSamples {
[ContentType(
DisplayName = "My Page Type",
Description = "Description for this page type",
Order = 1024,
GUID = "9CBBF910-CB5A-4C72-83AA-EDCF02E8A2BD",
GroupName = "My Group",
AvailableInEditMode = true)]
[AvailableContentTypes(Include = new Type[] {
typeof (MyPageType2), typeof (MyPageType3)
})]
[Access(Users = "niis", Roles = "CmsEditors")]
public class TypedPageWithAttributeSample: PageData {
[Required]
[Searchable]
[CultureSpecific]
[Editable(true)]
[Display(
Name = "My Heading",
Description = "Heading for my page type.",
GroupName = "My Tab",
Order = 64)]
public virtual string Heading {
get;
set;
}
//An integer between 0 and 130.
[Range(0, 130)]
public virtual int Age {
get;
set;
}
//Validating against a given regular expression.
[RegularExpression("[SomeRegularExpression]")]
public virtual string CustomValidation {
get;
set;
}
//A string with a maximum length of 20 characters.
[StringLength(20)]
public virtual string Header {
get;
set;
}
//Any URL
public virtual Url RedirectPage {
get;
set;
}
//Reference to an image in the EPiServer media system.
[UIHint(UIHint.Image)]
public virtual ContentReference Logotype {
get;
set;
}
//URL to image is also supported but mostly for upgrade support or when there is a need to add custom query parameters to the URL.
[UIHint(UIHint.Image)]
public virtual Url LogotypeAsUrl {
get;
set;
}
//Creates a selection of predefined values.
[SelectOne(SelectionFactoryType = typeof (CustomLanguageSelectionFactory))]
public virtual string LanguageSelection {
get;
set;
}
//Creates a selection of predefined values with the option to select several items.
[SelectMany(SelectionFactoryType = typeof (CustomLanguageSelectionFactory))]
public virtual string MultipleLanguageSelection {
get;
set;
}
}
public class CustomLanguageSelectionFactory: ISelectionFactory {
public IEnumerable < ISelectItem > GetSelections(ExtendedMetadata metadata) {
var languages = new List < SelectItem > ();
languages.Add(new SelectItem() {
Text = "English", Value = "en"
});
languages.Add(new SelectItem() {
Text = "Swedish", Value = "sv"
});
languages.Add(new SelectItem() {
Text = "Norwegian", Value = "no"
});
return languages;
}
}
[AvailableContentTypes(IncludeOn = new Type[] {
typeof (TypedPageWithAttributeSample)
})]
[ContentType]
public class MyPageType1: PageData {}
[AvailableContentTypes(Exclude = new Type[] {
typeof (TypedPageWithAttributeSample)
})]
[ContentType]
public class MyPageType2: PageData {}
[AvailableContentTypes(ExcludeOn = new Type[] {
typeof (MyPageType1)
})]
[ContentType]
public class MyPageType3: PageData {}
[AvailableContentTypes(Availability = Availability.None)]
[ContentType]
public class MyPageType4: PageData {}
}
Updated about 14 hours ago