Content templates
Describes the concept of content templates used for rendering content in Optimizely.
Using templates, you can apply multiple templates for any content, and have the system decide when to use which template.
See Render content for information about rendering in CMS.
Note
The examples in this topic are based on ASP.NET Core.
MVC controllers and views
Models, controllers, and views in MVC separate data, business logic, and presentation. The controller contains the business logic, handles URL requests, and selects the view, a visual data model presentation. In MVC, the controller is the class registered to support specific page types, and can be regarded as the template. The template defines which content types it can render in a specific context.
Create a controller and a view
To add a new template controller, create a MVC controller in your project's Controllers folder. Your controller should inherit from EPiServer.Web.Mvc.ContentController<T>
(or optionally EPiServer.Web.Mvc.PageController<T>
for PageData
instances), where T is your content model. This controller is called when an instance of the content type is routed if chosen as the renderer for the content instance.
To add the corresponding view for the controller, create a subfolder under Views and add an item of type View. You should follow the standard naming conventions in MVC for your model, controllers, and views.
A page controller base class lets multiple pages reuse logic, which holds logic for a logout action, and inherits from PageController
. You can also add a view models to add more than just page objects to the views. (View model is not used in these examples to keep the examples simpler.)
To render properties, you can use HTML helpers in MVC, for example, Html.PropertyFor
, which renders property values based on their property type. HTML helpers are described more below.
Example: The following example uses the content types created in Content types. The controller for displaying the Article page type, inheriting from PageControllerBase
.
using AlloyTemplates.Controllers;
using AlloyTemplates.Models.Pages;
using EPiServer.Framework.DataAnnotations;
using EPiServer.Shell.Security;
using Microsoft.AspNetCore.Mvc;
namespace MyOptimizelySite.Controllers {
public class ArticlePageController: PageControllerBase<ArticlePage> {
public ArticlePageController(UISignInManager uiSignInManager): base(uiSignInManager) {}
public ActionResult Index(ArticlePage currentPage) {
return View(currentPage);
}
}
}
Example:Â The page controller base, inheriting from PageController
, and with SitePageData
as generic type.
namespace AlloyTemplates.Controllers {
public abstract class PageControllerBase<T>: PageController<T>, IModifyLayout
where T: SitePageData {
private readonly UISignInManager _uiSignInManager;
public PageControllerBase(UISignInManager uiSignInManager) {
_uiSignInManager = uiSignInManager;
}
public async Task<IActionResult> Logout() {
await _uiSignInManager.SignOutAsync();
return RedirectToAction("Index");
}
}
}
Example: The corresponding rendering view for displaying the Article page.
@using EPiServer.Core
@using EPiServer.Web.Mvc.Html
@model MyOptimizelySite.Models.Pages.ArticlePage
<h1>
@Html.DisplayFor(m => m.Heading)
</h1>
<h3>
@Html.PropertyFor(m => m.Introduction)
</h3>
<div>
@Html.PropertyFor(m => m.MainBody)
</div>
With the added rendering using Html.PropertyFor
, you can edit the property in the On-page editing view.
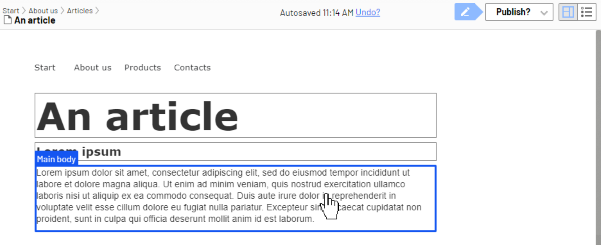
Create a Razor page
Using controllers and views to render requests is an alternative to using Razor Pages. A razor contains of two different files (similar to a controller and a view), one Razor Page file with extension .cshtml and a corresponding page model file with extension .cshtml.cs. This separates the logic of a page from its presentation.
To add new template as a Razor Page, create a new Razor Page in the Pages folder in your project. Your page model should inherit from EPiServer.Web.Mvc.RazorPageModel<T>
, where T is your content model. This Razor Page is called when an instance of the content type is routed to, if chosen as the renderer for the content instance.
To render properties you can use HTML helpers in MVC, for example Html.PropertyFor
, which render property values based on their property type. HTML helpers are described more below.
Example: This example uses the content types created in section Content types. The Razor Page for displaying the Article page type, where page model inherits from RazorPageModel<ArticlePage>
.
namespace AlloyMvcTemplates.Pages {
public class ProductModel: RazorPageModel<ArticlePage>, IPageViewModel<ArticlePage> {
public ProductPage CurrentPage => CurrentContent;
public LayoutModel Layout {
get;
set;
}
public IContent Section {
get;
set;
}
public void OnGet() {
Layout = HttpContext.RequestServices.GetService<PageViewContextFactory> ()
.CreateLayoutModel(CurrentContent.ContentLink, HttpContext);
}
}
}
Example: The corresponding rendering view for displaying the Article page.
@page
@model AlloyMvcTemplates.Pages.ProductModel
@{
Layout = "~/Views/Shared/Layouts/_Root.cshtml";
}
<h1 @Html.EditAttributes(x => x.CurrentContent.PageName)>@Model.CurrentContent.PageName</h1>
<p class="introduction" @Html.EditAttributes(x => x.CurrentContent.MetaDescription)>@Model.CurrentContent.MetaDescription</p>
<div class="row">
<div class="span8 clearfix" @Html.EditAttributes(x => x.CurrentContent.MainBody)>
@Html.DisplayFor(m => m.CurrentContent.MainBody)
</div>
</div>
@Html.PropertyFor(x => x.CurrentContent.MainContentArea, new { CssClass = "row", Tag = Global.ContentAreaTags.TwoThirdsWidth })
Block components and views
In MVC, the rendering of blocks is done by using view components and/or views and associated templates, similar to the way you render pages. You should use a block component if some logic needs to be applied when the block is rendered; otherwise, use a partial view.
- Create a view component that inherits fromÂ
EPiServer.Web.Mvc.BlockComponent<TBlockData>
orEPiServer.Web.Mvc.AsyncBlockComponent<TBlockData>
, where TBlockData is your block type. The system calls this view component for the block type if it is chosen as the renderer of the current block instance.EPiServer.Web.Mvc.BlockComponent<TBlockData>
has an implementation of the methodInvoke
, which calls a partial view according to the MVC conventions that apply to view components. - Create a partial view without a view component, naming the view the same as the block type. If the view is chosen as the renderer of the block type, the view is called with the block instance directly, without controller involvement. You should render blocks with this approach.
Create a block component
To add a template for a block as a view component, create a ViewComponent
in the Components folder in your project. Your component model should inherit from EPiServer.Web.Mvc.BlockComponent<TBlockData>
or EPiServer.Web.Mvc.AsyncBlockComponent<TBlockData>
.
To add the corresponding view for the view component, create a view following the convention /Views/Shared/Components/{View Component Name}/Default.cshtml
.
Example: The view component for a block:
namespace AlloyTemplates.Components {
public class PageListBlockViewComponent: BlockComponent<PageListBlock> {
private readonly IContentLoader _contentLoader;
public PageListBlockViewComponent(IContentLoader contentLoader) {
_contentLoader = contentLoader;
}
protected override IViewComponentResult InvokeComponent(PageListBlock currentBlock) {
var model = new PageListModel(currentBlock) {
Pages = _contentLoader.GetChildren<PageData>(currentBlock.Root, new LoaderOptions(), 0, currentBlock.Count)
};
return View(model);
}
}
}
Example: The view for the PageListBlock
view component:
@model PageListModel
@Html.FullRefreshPropertiesMetaData(new[] { "IncludePublishDate", "IncludeIntroduction", "Count", "SortOrder", "Root", "PageTypeFilter", "CategoryFilter", "Recursive" })
<h2 @Html.EditAttributes(x => x.Heading)>@Model.Heading</h2>
<hr />
@foreach(var page in Model.Pages) {
<div class="listResult @string.Join(" ", page.GetThemeCssClassNames())">
<h3>
@Html.PageLink(page)
</h3>
@if(Model.ShowPublishDate && page.StartPublish.HasValue) {
<p class="date">@Html.DisplayFor(x => page.StartPublish)</p>
}
@if(Model.ShowIntroduction && page is SitePageData sitePageData) {
<p>@sitePageData.TeaserText</p>
}
<hr />
</div>
}
Create a partial view
In Visual Studio, add a partial view with the same name as your block type and, based on your block model class, to the Views/Shared
 folder of your project.
Example:Â The partial view for the TeaserBlock block type, displaying a heading and an image.
@model MyOptimizelySite.Models.Blocks.TeaserBlock
<div>
<h2>@Html.PropertyFor(x => x.Heading)</h2>
<img src="@Url.ContentUrl(Model.Image)" />
</div>
Partial templates
Content types other than blocks (for example, pages) can also have associated partial templates (view components or partial views). These are then used if the content item is added to a ContentArea
. As for block templates, partial templates for other content types can also be view components or partial views. If view components are used, then the base class should be EPiServer.Web.Mvc.PartialContentComponent<TContentData>
or EPiServer.Web.Mvc.AsyncPartialContentComponent<TContentData>
.
Endpoint templates
In addition to MVC controllers and Razor Pages, you also can add a template mapping to a Endpoint. For example, content media requests in CMS are handled through an endpoint template. Custom-content endpoints that serve content needs to add a ContentActionDescriptor
to the endpoint metadata. You also should add the policies for CmsPolicyNames.Read
and CmsPolicyNames.Preview
to ensure access rights are checked.
Example: The following example shows a custom endpoint template for PDF files that adds a header to the response.
[TemplateDescriptor(Inherited = true, TemplateTypeCategory = TemplateTypeCategories.HttpHandler)]
public class PdfEndpoint: Endpoint, IRenderTemplate<PdfFile> {
private readonly static ContentActionDescriptor _mediaContentActionDescriptor = new ContentActionDescriptor {
Inherited = true, ModelType = typeof (PdfFile)
};
private static readonly AuthorizeAttribute _authorizeAttribute = new AuthorizeAttribute(CmsPolicyNames.Read);
private static readonly AuthorizeAttribute _previewAttribute = new AuthorizeAttribute(CmsPolicyNames.Preview);
public PdfEndpoint(IBlobHttpHandler blobHttpHandler): base(context => ProcessRequest(blobHttpHandler, context),
new EndpointMetadataCollection(_mediaContentActionDescriptor, _authorizeAttribute, _previewAttribute), nameof(PdfEndpoint)) {}
private static Task ProcessRequest(IBlobHttpHandler blobHttpHandler, HttpContext context) {
context.Response.Headers.Add("Content-Disposition", "attachment");
return blobHttpHandler.Invoke(context);
}
}
The example above sets a header for a media request. Even though you can define custom endpoints for media, in many cases, it is easier to extend the default media handling by registering an implementation of IStaticFilePreProcessor
.
Example: The following example shows a custom pre-processor that adds a header to the response for PDF requests.
public class PdfStaticFilePreProcessor: IStaticFilePreProcessor {
//The built-in pre processor that handles MediaOptions has order 0, run after that
public int Order => 10;
public void PrepareResponse(StaticFileResponseContext staticFileResponseContext) {
if (staticFileResponseContext.Context.Response.ContentType == "application/pdf") {
staticFileResponseContext.Context.Response.Headers.Add("Content-Disposition", "attachment");
}
}
}
The static file pre-processor is registered using MediaOptions
, like:
services.Configure<MediaOptions>(o => o.AddPreProcessor<PdfStaticFilePreProcessor>());
Model binding
If an MVC controller has a parameter that is a content instance, then CMS automatically binds the routed content to the parameter, see Action
method on ArticleController
above. For Razor Pages, is the routed content bound to the property RazorPageModel<T>
.CurrentContent, see usage in ArticleModel
above. For view components, the current content is passed as arguments for arguments named currentContent
or currentBlock
.
HTML Helpers
You can also use other specific Optimizely HTML helpers as an alternative to Html.PropertyFor
. There are helpers for rendering links, content areas, translations, and navigation. These HTML helpers are called through Html.DisplayFor
, but you can also use them directly.
Templates
Templates define how content is rendered. The template (controller, partial view, and so on) selected to render a content instance, depends on the specific context. Use the TemplateDescriptor
attribute to add metadata and define default templates, and Tags to define which template to use. Based on this information, and any defined display channels and display options settings, the TemplateResolver
decides which template to use in a specific context. Note that if you are using partial views and no view component for partial templates, you cannot implement the TemplateDescriptor
. Instead, you can implement the IViewTemplateModelRegistrator
interface to register templates. See Render content and the CMS sample site for examples.
Shared blocks folders
As previously mentioned, shared blocks are stored, loaded, and versioned individually as an entity in the database. Shared blocks are structured using folders, and a Folder is an instance of EPiServer.Core.ContentFolder
. Content folders do not have associated rendering, and, therefore no visual appearance on the website.
A folder in the shared blocks structure can have other folders or shared blocks as children, and a shared block cannot have any children.
You set editorial access on folders to specify which folders are available for an editor. The global folder root EPiServer.Web.SiteDefinition.Current.GlobalAssetsRoot
, is the root folder for shared blocks available for sites in an enterprise scenario. There can be a site-specific folder EPiServer.Web.SiteDefinition.Current.SiteAssetsRoot
, containing the folder structure for shared blocks. GlobalBlocksRoot and SiteBlocksRoot typically point to the same folder in a single-site scenario.
Updated 7 months ago