Create a CMS starter project
Describes how to create an empty Optimizely Content Management System (CMS) project, add a page type and configure a start page for the site.
You should start developing in an empty project for better control and understanding. Before doing this (and if you are new to Optimizely), you should install and explore a sample site and get a Optimizely user interface.
Create the project
Follow Set up a development environment to install the Optimizely templates and command line tool. Then run the following commands. Check command line options for more configuration options.
dotnet new epi-cms-empty
The project structure
The project creation process completes these actions:
- Installs main components through NuGet packages (
EPiServer.CMS
). - Creates a database and database user.
- Updates configuration files, such asÂ
appSettings.json
, with connection strings. - Installs the Optimizely user interface components and places them under the URL /episerver.
When finalized, you will have a project folder structure containing the following:
- Properties with the launch settings for the development server.
- wwwroot for client resources.
- modules for package modules.
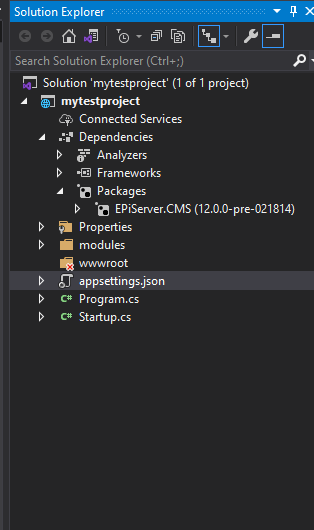
Note
When you install an empty site, there is no search feature, which is added later.
Create a page typeÂ
Create a simple standard page type based on MVC, with an editorial area and a heading. Page types created this way inherit from the IContent
interface in Content model and views, providing some built-in functionality used in many content types.
Note
You need to be an administrator for the site to create a page under the website root.
-
Add a new class named
StandardPage.cs
.using EPiServer.Core; using EPiServer.DataAbstraction; using EPiServer.DataAnnotations; using System.ComponentModel.DataAnnotations; namespace EPiServer.Reference.Commerce.Site.Features.Shared.Pages { [ContentType( DisplayName = "Standard page", GUID = "934E7266-FB8C-4DEA-B033-3B4E6AE6CBCF", Description = "The standard page.", AvailableInEditMode = true)] [ImageUrl("~/styles/images/page_type.png")] public class StandardPage: PageData { [CultureSpecific] [Display( Name = "Title", Description = "Title for the page", GroupName = SystemTabNames.Content, Order = 1)] public virtual string Title { get; set; } [CultureSpecific] [Display( Name = "Main body", Description = "Main body", GroupName = SystemTabNames.Content, Order = 2)] public virtual XhtmlString MainBody { get; set; } } }
-
Compile your solution, log in, and go to edit view.
-
Under Start (root level), create a page named First page. The StandardPage is automatically applied because this is currently the only available page type.
-
Add some text in the heading and main body properties, and publish the page. Because there is no rendering, the page will open in the All Properties editing view and will not be accessible on the front-end side.
Add page rendering
Add rendering to make the page accessible on the front-end side. This also automatically makes the On-page editing view accessible for editing selected properties.
-
Add a new class named
StandardPageController.cs
using EPiServer.Core; using EPiServer.Reference.Commerce.Site.Features.Shared.Pages using EPiServer.Web.Mvc; using EPiServer.Web.Routing; using Microsoft.AspNetCore.Mvc; using System; namespace EPiServer.Reference.Commerce.Site.Features.Shared.Controllers { public class StandardPageController: PageController < StandardPage > { [HttpGet] public IActionResult Index(StandardPage currentPage) { return View(currentPage); } } }
-
Right-click the Views folder, select Add > New Folder, and name it StandardPage.
-
Right-click the StandardPage folder, select Add > New item, and then Razor View Empty.
-
Name the view
Index.cshtml
and click Add. -
In the
Index.cshtml
file, change the model toYourSite.Models.Pages.StandardPage
, add the rendering for the Title property with an <h2> tag before the MainBody property.@using EPiServer.Web.Mvc.Html @model EPiServer.Reference.Commerce.Site.Features.Shared.Pages.StandardPage <div class="container-fluid"> <div class="row myPage"> <div class="col-xs-12 col-md-9"> <h2>@Html.PropertyFor(x => x.Title)</h2> @Html.PropertyFor(x => x.MainBody) </div> </div> </div>
-
Build your solution, and log in to the edit view. The page you created now has rendering and can be edited through the On-Page edit view.
-
Select View on website under Publishto verify that the page can be accessed from the front-end side. Notice the URL reflecting the language and page structure of the site.
-
Add a few more pages to your site for a page tree structure.
Configure the start page
After you have a set of accessible pages, you still must configure the site. In this final step, you configure the site from the Optimizely admin view.
- Configure the site start page by going to Admin > Configuration > Manage Sites.
- Click Add, and enter the site name and URL (localhost).
- Select a page in the page tree structure as the start page, and click Save.
Notice the new start page in your page tree structure. This also means you can access your site from the front-end by browsing to the URL you have configured.
After completing this, you have a working site with a start page configured, to be used as a starting point for continued development. The next steps are typical in an Optimizely implementation.
Updated 5 months ago