Extend the Tasks pane with custom queries
Describes how to extend the task pane that is part of the Optimizely Content Management System (CMS) user interface.
The Tasks panel contains predefined lists with queries like Draft, Rejected, or Recently Changed. You can also build a query and plug it into the tasks list.
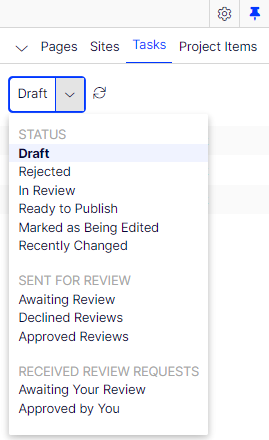
To add your query, implement IContentQueryInterface
or inherit from one of the base classes that already have this interface (ContentQueryBase
, ContentActivitiesQueryBase
).
public class CustomQuery : ContentQueryBase {
// …
}
The query has to be registered in an IOC container under the IContentQuery
type (using ServiceConfiguration
attribute).
[ServiceConfiguration(typeof(IContentQuery))]
public class CustomQuery : ContentQueryBase {
// …
}
Implement the following properties:
Name
– unique name for the query.DisplayName
– label displayed in the drop-down list, used for grouping queries in the list.SortOrder
– defines the position on the list.VersionSpecific
– determines if query results should be considered version-specific when comparing links.PluginAreas
– to make the query visible in the tasks list, setPluginAreas
toEPiServer.Shell.ContentQuery.KnownContentQueryPlugInArea.EditorTasks
string constant.
public override IEnumerable<string> PlugInAreas = new string[] { KnownContentQueryPlugInArea.EditorTasks };
Implement a custom query
In this example, create a query to display pages manually marked as being edited.
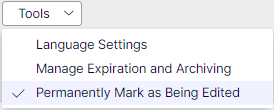
There is a repository registered under the IInUseNotificationRepository
interface that returns its content, used in GetContentMethod
.
[ServiceConfiguration(typeof (IContentQuery))]
public class MarkAsEditedQuery: ContentQueryBase {
private readonly IContentRepository contentRepository;
private readonly IInUseNotificationRepository inUseNotificationRepository;
public MarkAsEditedQuery(IContentQueryHelper queryHelper, IContentRepository contentRepository,
IInUseNotificationRepository inUseNotificationRepository): base(contentRepository, queryHelper) {
this.contentRepository = contentRepository;
this.inUseNotificationRepository = inUseNotificationRepository;
}
public override string Name => "markedAsEdit";
public override string DisplayName => "Marked as edit";
public override IEnumerable<string> PlugInAreas => new string[] {
KnownContentQueryPlugInArea.EditorTasks
};
public override int SortOrder => 105;
public override bool VersionSpecific = rt false;
protected override IEnumerable<IContent> GetContent(ContentQueryParameters parameters) {
return inUseNotificationRepository.GetAllInUseNotifications()
.Where(n => n.AddedManually)
.Select(c => contentRepository.Get<IContent>(c.ContentGuid));
}
}
After running the application, the query is placed under the OTHERS category in the Tasks menu.
To place it under one of the existing categories, such as STATUS, add a generic type to ContentQueryBase
or add a generic IContentQuery
interface.
public class MarkAsEditedQuery : ContentQueryBase<TasksStatusQueryCategory>
// or
public class MarkAsEditedQuery : ContentQueryBase, IContentQuery<TasksStatusQueryCategory>
Create custom query categories
Category
is a class that implements the IContentQueryCategory
interface and is registered in the IOC container under the IContentQueryCategory
type (using ServiceConfiguration
attribute).
[ServiceConfiguration(typeof(IContentQueryCategory), Lifecycle = ServiceInstanceScope.Singleton)]
public class AlloyQueryCategory : IContentQueryCategory {
public string DisplayName => "Alloy tasks";
public int SortOrder => 100;
}
The class has to implement DisplayName
, which could be the name or key to a localization service, and SortOrder
, which is used when sorting categories in the list. Instead of using TasksStatusQueryCategory
, use the AlloyQueryCategory
.
Updated 11 months ago