Example: Create visitor group criteria
Shows how to develop a criteria for categorizing visitors according to whether a specific cookie exists in the session.
(Thanks to Ted & Gustaf for this example.)
One usage scenario could be to check for a cookie that says that the visitor has previously completed a purchase on the website and is a returning customer.
Create the settings and criterion classes
Visitor group criteria require a settings
class and a criterion
class.
- The
settings
class is used to persist any settings available to editors when creating visitor groups. - The
criterion
class contains the logic to determine whether or not a visitor belongs to the visitor group.
You should create the two classes in a suitable folder structure:
Implement the settings class
The only setting required for this visitor group criterion is the cookie name you should look for.
-
First, make the
CookieExistsCriterionSettings
class inherits theCriterionModelBase
class:public class CookieExistsCriterionSettings : CriterionModelBase
-
Add a public property to let editors specify the cookie name:
[Required] public string CookieName { get; set; }
-
If you need custom validation logic when you save a criterion, you can make your settings class implement the
IValidateCriterionModel
interface (this is optional). By implementing that interface’sValidate
method, you can customize how you validate settings before saving a criterion for a visitor group.The abstract
CriterionModelBase
class requires you to implement theCopy()
method. Because you are not using complex reference types, you can implement it by returning a shallow copy as shown (see Create custom visitor group criteria):public override ICriterionModel Copy() { return ShallowCopy(); }
The following code shows the complete
CookieExistsCriterionSettings
class:public class CookieExistsCriterionSettings: CriterionModelBase { [Required] public string CookieName { get; set; } public override ICriterionModel Copy() { return ShallowCopy(); } }
Implement the criterion class
-
Make the
CookieExistsCriterionclass
inherit the abstractCriterionBaseclass
with the settings class as the type parameter:public class CookieExistsCriterion : CriterionBase<CookieExistsCriterionSettings>
-
Add a
VisitorGroupCriterion
attribute to set the category, name, and description of your criterion (for more availableVisitorGroupCriterion
properties, see Create custom visitor group criteria:[VisitorGroupCriterion( Category = "Technical", DisplayName = "Cookie Exists", Description = "Checks if a specific cookie exists")]
-
The abstract
CriterionBase
class requires you to implement anIsMatch()
method, which determines whether the current user matches this visitor group criterion. You should check if a specific cookie exists based on the criterion settings:public override bool IsMatch(IPrincipal principal, HttpContextBase httpContext) { return httpContext.Request.Cookies[Model.CookieName] != null; }
The criterion class displays as follows:
[VisitorGroupCriterion( Category = "Technical", DisplayName = "Cookie Exists", Description = "Checks if a specific cookie exists")] public class CookieExistsCriterion: CriterionBase<CookieExistsCriterionSettings> { public override bool IsMatch(IPrincipal principal, HttpContextBase httpContext) { return httpContext.Request.Cookies[Model.CookieName] != null; } }
Note
You access the criterion settings instance through the
Modelproperty
.
Test the criterion
Create a visitor group using the new cookie criterion to test the criterion.
- Set the cookie name to .EPiServerLogin, the name of the cookie created when a user logs in:

If you are on CMS version 10 or 11, it will look like this:
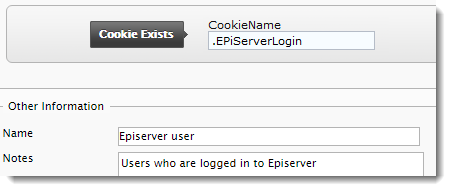
- Select some content in the editor that you should display only to logged-in Episerver users.

- Click Personalized Content and select the Episerver User visitor group.
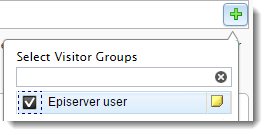
This content is now only displayed to users logged into Episerver:
After publishing the page, you see this:
However, you no longer see the personalized content if you log out.
Updated 10 months ago