Use a block as a property
Describes how to add and render an existing block as a property in an existing page type.
Blocks in Optimizely Content Management System (CMS) are reusable, smaller content parts that editors can add to pages. You can also use blocks in code as properties, which is a convenient way to reuse a set of properties in multiple instances.
You can render blocks only in the context of other content, such as a page. A block instance is part of a page instance (if a PageType
or BlockType
contains a property of the block type) or a shared instance. When you use a block as a property on a page, it is stored, loaded, and versioned with that page.
Add a block as a property
Assume you have an existing page type Standard Page, to which you want to add an existing block type Teaser Block. The page type has a main body property of type XhtmlString
. The block has a Heading
property of type String
, and an Image property of type ContentReference
.
In the example below, we add the block as a property to the page type and update the corresponding view for the page type to display it as a property.
Example: The Teaser Block block type.
using System;
using System.ComponentModel.DataAnnotations;
using EPiServer.Core;
using EPiServer.DataAbstraction;
using EPiServer.DataAnnotations;
using EPiServer.Web;
namespace MyOptimizelySite.Models.Blocks {
[ContentType(DisplayName = "TeaserBlock", GUID = "38d57768-e09e-4da9-90df-54c73c61b270", Description = "Heading and image.")]
public class TeaserBlock: BlockData {
[CultureSpecific]
[Display(
Name = "Heading",
Description = "Add a heading.",
GroupName = SystemTabNames.Content,
Order = 1)]
public virtual String Heading {
get;
set;
}
[Display(
Name = "Image", Description = "Add an image (optional)",
GroupName = SystemTabNames.Content,
Order = 2)]
public virtual ContentReference Image {
get;
set;
}
}
}
Example: The Standard Page page type with Teaser Block added as a property.
using System;
using System.ComponentModel.DataAnnotations;
using EPiServer.Core;
using EPiServer.DataAbstraction;
using EPiServer.DataAnnotations;
using EPiServer.SpecializedProperties;
using MyOptimizelySite.Models.Blocks;
namespace MyOptimizelySite.Models.Pages {
[ContentType(GroupName = "Basic pages", Order = 1, DisplayName = "StandardPage", GUID = "abad391c-5563-4069-b4db-1bd94f7a1eea",
Description = "To be used for basic content pages.")]
public class StandardPage: SitePageData {
[CultureSpecific]
[Display(
Name = "Main body",
Description = "The main body for inserting for example text, images and tables.",
GroupName = SystemTabNames.Content,
Order = 1)]
public virtual XhtmlString MainBody {
get;
set;
}
[Display(Order = 5, GroupName = SystemTabNames.Content)]
public virtual TeaserBlock Teaser {
get;
set;
}
}
}
Example: The view for the Standard Page.
@using EPiServer.Core
@using EPiServer.Web.Mvc.Html
@model MyOptimizelySite.Models.Pages.StandardPage
<div>
@Html.PropertyFor(m => m.MainBody)
@Html.PropertyFor(m => m.Teaser)
</div>
The following image shows the result when editing a page based on the Standard Page page type in the All Properties editing view.
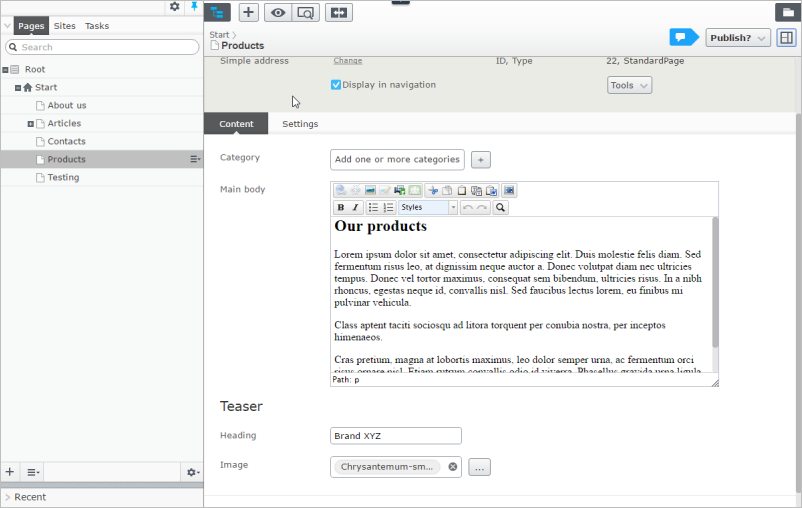
Note
The functionality "Fetch content from another content item" will only apply when a property is considered "Null". For a block property this means that all properties within the block must be null for fetch content to apply. This can be an issue if the block contains a primitive type, such as a bool, nullable bool, int or nullable int, and that has a value. It is currently not possible to set a null value for those properties from the edit UI, meaning that if such a block property has a value it cannot be "nulled" from the user interface to get fetching data to apply. This can be solved by either a custom editor that can set null value as well or by having an event handler to
IContentEvents.PublishingContent
and in the event handler set the value to null if it is default value (false for a bool/nullable boolean or 0 for an int/nullable int).
Updated 12 months ago