Refactor content type classes
Explains how to work with refactoring, when you change, rename or delete content types in Optimizely Content Management System (CMS).
Rename a content type by GUID
When you rename a content type class, you create a new content type as long as you do not specify a GUID in the ContentTypeAttribute
of the class. If there is no data in the previous content type, it is deleted. However, if you created pages with the previous content type named, the previous page type remains, and the previous pages use the previous content type. When you view the content type in admin view under the Page Type or Block Type tab, the previous content type is marked as missing its code.
If you specify a GUID in the ContentTypeAttribute
and the GUID matches an existing content type, you rename the content type, and any previous data uses the renamed content type. The admin view displays the GUID of an existing content type when you edit the basic information for a content type.
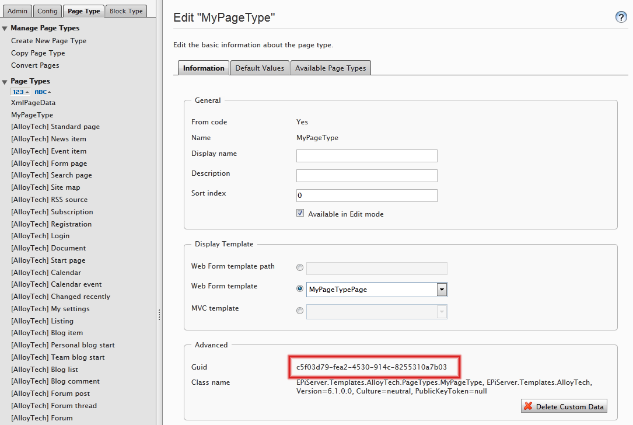
Rename a content type by API
When you rename a content type or property using the specific API, create a MigrationStep
class with an AddChanges
method where you specify the names of the content types or properties to ensure that the existing properties are renamed and retain values they had before you renamed them. The API is based on strings to know the names of previous types and properties.
Note
You do not need to manually register the
MigrationStep
class, since it will be automatically detected.
The following example shows how to rename a content type and a property on that content type:
using EPiServer.DataAbstraction.Migration;
namespace CodeSamples {
public class MigrationStepExample: MigrationStep {
public override void AddChanges() {
RenameContentType();
RenameProperty();
}
private void RenameContentType() {
//The content type formerly known as "Velocipede" should hereby be known as "Bicycle".
ContentType("Bicycle")
.UsedToBeNamed("Velocipede");
}
private void RenameProperty() {
ContentType("Bicycle") //On the content type "Bicycle"
.Property("PneumaticTire") //There is a property called "PneumaticTire"
.UsedToBeNamed("WoodenTire"); //That used to be called "WoodenTire"
}
}
}
Change the type for a property
The following criteria are prerequisites before you can change a property's type. They ensure that a change of type in the code does not lose data.
- You must be able to set the previous value to the new type, or you can parse it in the
ParseToSelf
method of the new types backingPropertyData
. - The value of the backing
PropertyData
you are changing to must implement theSystem.IConvertible
interface. - The backing
PropertyData
also must have itsType
property set to any of the seven first values of thePropertyDataType
enumeration. - The previous or new property type cannot be a block.
- If the new type is
String
, then any previous value cannot be longer than 255 characters.
If converting existing data to the new type fails, it logs an error and commits nothing.
If you need a change of type and any data loss is acceptable, you can change the property type in admin mode, but you must temporarily remove the property from the code.
Delete a content type class or property
When you delete a content type or property from the code, the system synchronizes the database as much as possible without deleting data (values saved on any page). If no data is present, the content type or property is deleted from the database. If any data is present, the content type or property remains and (in the admin view) marks it as missing its code.
Delete a culture-specific attribute on a property
When you manually change a property in the admin view from being culture-specific to not being culture-specific, the change may not be reflected on the site when you store culture-specific data in the database. If this occurs, it logs an error but makes no change to the property in the database.
Updated about 1 year ago