Generic PropertyList
Describes how to define an editable list of objects.
Important
This topic describes unsupported functionality for complex types in Optimizely Content Management System (CMS) that the implementing developer is responsible for validating to ensure that it works for the item types you want, because supporting complex types as list items brings a whole set of unknowns. Using
PropertyList<T>
together with complex types requires additional custom functionality to fully ensure data consistency, portability and functionality. This includes, but is not limited to UI, Settings, Export/Import, Permanent link handling and default values.
CMS recommends using ContentArea
and Blocks
for lists of complex objects. Using blocks for complex types provides a much more controlled object structure.
PropertyList
is a property that lets you define an editable list of objects. The content model can implement a property of type IList<T>
where T is a class with property definitions.
public virtual IList<CustomItem> List{
get;
set;
}
Example – list of locations
This example shows how to implement a list of locations.
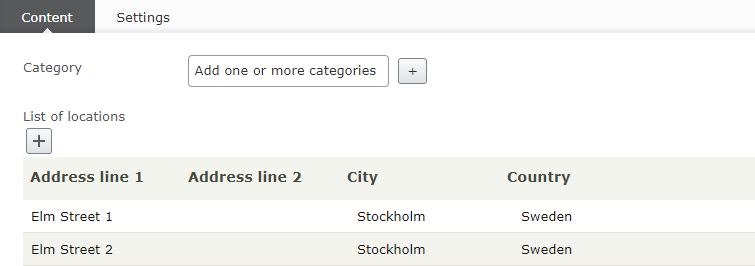
PropertyList
lets the editor edit items using a dialog window.
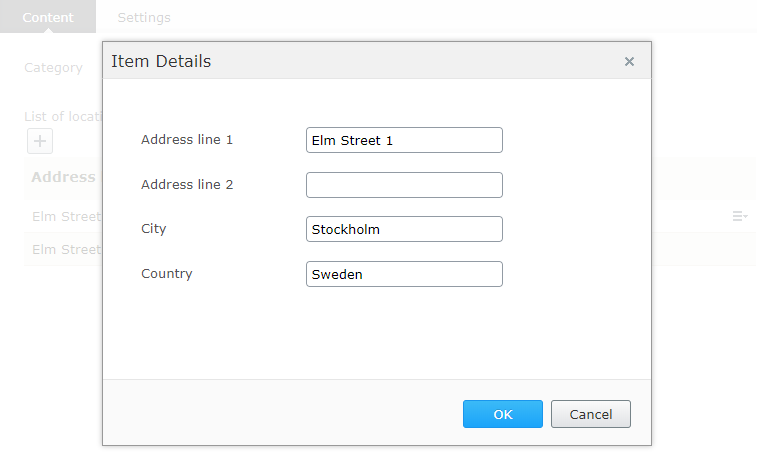
The list contains items with string properties:
- address list 1
- address line 2
- city
- country
First, define the Location
model class. It will represent the property item.
using System.ComponentModel.DataAnnotations;
namespace Site.Models.Pages {
public class Location {
[Display(Name = "Address line 1")]
public virtual string AddressLine1 {
get;
set;
}
[Display(Name = "Address line 2")]
public virtual string AddressLine2 {
get;
set;
}
public virtual string City {
get;
set;
}
public virtual string Country {
get;
set;
}
}
}
The next step is to register the property definition using a custom property class LocationsProperty
that sets the generic type to Location
item class:
using EPiServer.PlugIn;
namespace Site.Models.Pages {
[PropertyDefinitionTypePlugIn]
public class LocationsProperty: PropertyList < Location > {}
}
Finally, add a list of locations on the page:
using System.ComponentModel.DataAnnotations;
using EPiServer.Core;
using EPiServer.DataAnnotations;
using EPiServer.Cms.Shell.UI.ObjectEditing.EditorDescriptors;
using EPiServer.Shell.ObjectEditing;
using System.Collections.Generic;
namespace Site.Models.Pages {
/// <summary>
/// Page with PropertyListDefinition
/// </summary>
[ContentType(GUID = "2CDDC73C-83AC-4F35-BA9D-50F285723A96")]
public class TestPage: PageData {
[Display(Name = "List of locations")]
[EditorDescriptor(EditorDescriptorType = typeof (CollectionEditorDescriptor<Location>))]
public virtual IList<Location> Locations {
get;
set;
}
}
}
Localize item properties
You can similarly translate list item properties as Content
properties translation. You can translate properties using DisplayAttribute
and language files. See Localizing the user interface.
Updated about 1 year ago