Create custom visitor group criteria
Describes visitor group criteria, which are the building blocks of a visitor group.
Optimizely Content Management System (CMS) comes with several predefined visitor group criteria, but you can develop your own.
Each criterion defines a condition that determines whether a visitor is part of a group. If a visitor fulfills a sufficient number of criteria, the visitor is considered a member of that group. The following example shows a fulfilled Time of Day criterion when the current time is between 8 a.m. and 12 p.m., except on weekends:
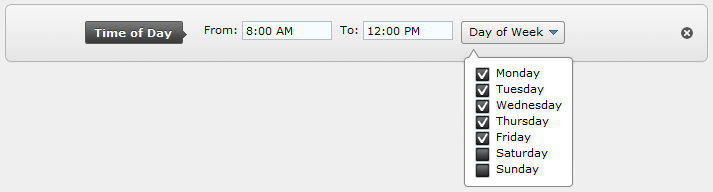
From a development standpoint, a visitor group criterion is a combination of (at least) two classes:
- A model class stores and persists user input from the user interface.
- A criterion class evaluates the context and the data stored in the model to determine if it fulfills the criteria.
Prerequisites
Ensure your project references and the following assemblies contain the classes you need to create criteria and models.
DojoWidget property attribute
If you want to use another Dojo widget than default, you can control that by decorating the properties on your model class with the DojoWidget
attribute. You also can use that attribute to set things like default value and where to find translations for the label. If you want more control over the user interface, create your own Editor templates or write a Custom Script as shown.
[DojoWidget(WidgetType = "dijit.form.FilteringSelect")]
public string MyString {
get;
set;
}
If you want the input for a value to be a drop-down list with predefined options, set SelectionFactoryType
on the DojoWidget
attribute. The SelectionFactoryType
is a type that implements ISelectionFactory
. The ISelectionFactory
has a GetSelectListItems
method that is responsible for supplying options for the drop-down list.
You can use an EnumSelectionFactory
included in the CMS to present the options of an Enum
in a drop-down list. When you use EnumSelectionFactory
, provide translations of the Enum
values and place the translated values in your own XML file in the /lang
directory. See Enumeration Localization in Localize the visitor group criterion.
[DojoWidget(
WidgetType = "dijit.form.FilteringSelect",
SelectionFactoryType = typeof (EnumSelectionFactory))]
public SomeEnum MyEnumSelector {
get;
set;
}
Validate the server side
You can add input validation to your properties by using the attribute classes in System.ComponentModel.DataAnnotations
. The following validation rules are supported:
[Required]
[Range(double Minimum, double maximum)]
[StringLength(int maximumLength)]
[RegularExpression(string pattern)]
[Required]
[StringLength(10)]
public string MyString {
get;
set;
}
If you want to add custom server-side validation, you can implement the interface IValidateCriterionModel
on your model. The IValidateCriterionModel
supplies a Validate
method called when you save a visitor group containing the criterion.
public class YourModelClass: CriterionModelBase, IValidateCriterionModel {
public string MyString {
get;
set;
}
public CriterionValidationResult Validate(VisitorGroup currentGroup) {
if (MyString.Length > 5) {
return new CriterionValidationResult(false, "MyString is too long!", "MyString");
}
return new CriterionValidationResult(true);
}
...
}
Create a criterion class
After you have a model class, create the criterion class to evaluate the context and the data stored in the model to determine whether it fulfills the criteria. CriterionBase
creates the connection between the criterion and model classes and is the base class that you must use for the criterion class, which is a generic class that accepts ICriterionModel
parameters. You must override the CriterionBase.IsMatch
method, which is the central method for a criterion; it is called when evaluating if a user is a member of a visitor group.
Decorate the criterion class with VisitorGroupCriterion
attribute, which identifies your class as a criterion and makes it available for use. VisitorGroupCriterion
has the following settings:
Category
– This group name in the criteria picker user interface where you can find this criterionDescription
– A text describing how the criterion works.DisplayName
– A short name that identifies the criterion in menus and visitor groups.LanguagePath
– The path in the XML language files where you can find the strings associated with this criterion. See Localize the visitor group criterion.ScriptUrl
– A URL referring to a JavaScript file you load when you edit this criterion.
[VisitorGroupCriterion(
Category = "My Criteria Category",
Description = "How the criterion works",
DisplayName = "Short Name",
LanguagePath = "/xml/path/to/translations/",
ScriptUrl = "javascript-that-should-be-loaded-for-the-UI.js")]
public class YourCriterionClass: CriterionBase<YourModelClass> {
public override bool IsMatch(IPrincipal principal, HttpContextBase httpContext) {
// Your evaluation code here.
// The model class instance is available via the Model property.
}
}
Subscribe to events
You can subscribe to specific events by overriding the Subscribe
method, which gathers information about events that occur before the call to the IsMatch
method. For example, the built-in Visited Page criterion needs to keep track of visited pages in the current session. You can subscribe to the following events:
EndRequest
StartRequest
StartSession
VisitedPage
If you override the Subscribe
method and attach event handlers, make sure that you also override the Unsubscribe
method and detach the event handlers.
public override void Subscribe(ICriterionEvents criterionEvents) {
criterionEvents.StartRequest += criterionEvents_StartRequest;
}
public override void Unsubscribe(ICriterionEvents criterionEvents) {
criterionEvents.StartRequest -= criterionEvents_StartRequest;
}
void criterionEvents_StartRequest(object sender, CriterionEventArgs e) {
// Handle the event
}
Updated 3 months ago