Create and edit content
Introduces creating and editing content, and content versioning in Optimizely Content Management System (CMS), from a code perspective.
Examples in this topic are based on an empty Optimizely Content Management System (CMS) project, as described in Create a starter project.
Create content
A content instance is an instance of a content type. You usually define content types in code as classes based on a model decorated with ContentTypeAttribute
inheriting from some base class like, for example EPiServer.Core.PageData
.
Inheriting from a base class like PageData
provides access to many built-in properties, for example Name
, ContentLink
, and ParentLink
. Built-in properties are predefined and set by the system when you create a page and are available regardless of content type. You can also add your own user-defined properties, based on available property types. See Built-in property types and Property attributes.
The following code shows a simple page type. MainBody
is a property of type XhtmlString
for adding content using a text editor.
using System;
using System.ComponentModel.DataAnnotations;
using EPiServer.Core;
using EPiServer.DataAbstraction;
using EPiServer.DataAnnotations;
using EPiServer.SpecializedProperties;
namespace MyEpiSite.Models.Pages {
[ContentType(
DisplayName = "TestPage",
GUID = "61f028c8-be3d-4942-b58c-65ea8b28e7b6",
Description = "Description for this page type")]
public class TestPage: PageData {
[CultureSpecific]
[Display(
Name = "Main body",
Description = "The main body will be shown in the main content area of the page, using the XHTML-editor you can insert for example text, images and tables.",
GroupName = SystemTabNames.Content,
Order = 1)]
public virtual XhtmlString MainBody {
get;
set;
}
The following image shows the same page type in the Admin view:
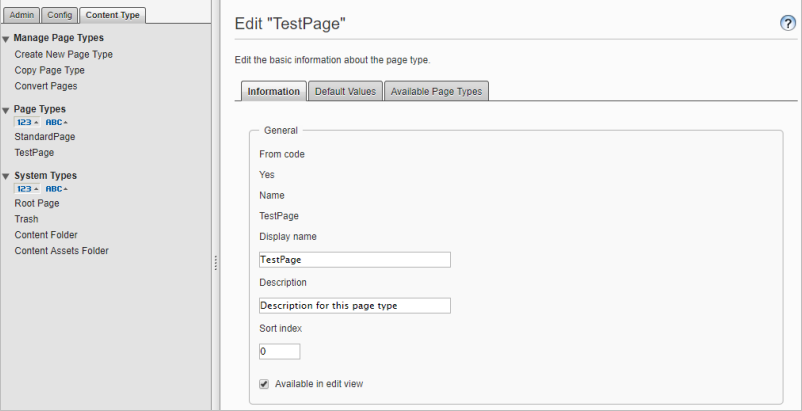
The GUID attribute on the ContentTypeAttribute
is the unique ID for a content type. Having the GUID in code is useful because you can refactor your code without getting duplicate content types in your database. It also reduces problems when you import and export content between databases.
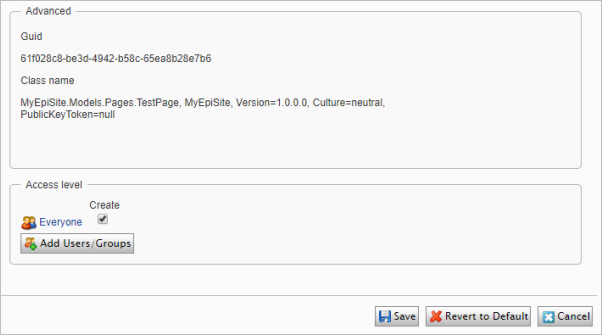
The following image shows a page based on the same page type in the All properties view. Notice the default Content and Settings tabs, and their built-in properties.
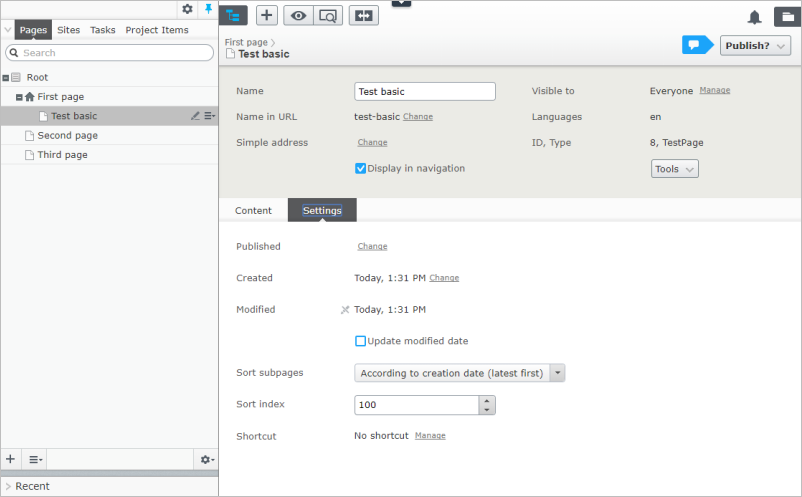
Tabs
Tabs are used for organizing related properties in edit view in a logical order. Use the attributes GroupName
and Order
to specify the tabs and order for properties on a page type. See Group content types and properties for how to create custom tabs and organize properties on them.
Edit content
You can edit content in CMS through the All properties view or On-page editing. The UI framework has a server-side part based on ASP.NET MVC Core and a client-side part using the JavaScript library Dojo. The UI also has context awareness, where components automatically reload when the context changes. See Edit the user interface.
A page based on a page type without a template (controller or view) can only be edited from the All properties view, as it has no rendering. Also, there is no preview available.
Adding rendering to a content type using the HTML helper Html.PropertyFor
, will add rendering of property values based on their property type. Adding this rendering will automatically make preview and on-page editing available. See Content types.
XHTML Editor
The default XHTML editor installed with CMS is TinyMCE editor, a flexible WYSIWYG editor that provides clean, validated XHTML markup. A standard installation of CMS provides built-in editor functionality, like dragging and dropping files and images from the Assets panel into the editor.
Content versioning
You can add a Versions gadget to the Assets panel in the user interface to manage different versions of a page, for example.
Most content items in CMS can be versioned. Implementing IVersionable
for a content item will add support for a set of content version states. The StartPublish
and StopPublish
properties, such as control content publish and expiration dates. See Content versions.
CMS automatically versions content items in a standard installation. The MaximumVersions
attribute on the ContentOptions
defines the number of previously published content versions the system will keep; the default value is 20. You can also change the value from the Admin view under Config > System Settings. Selecting Unlimited versions will set "uiMaxVersions = 0"
, meaning it keeps an unlimited number of versions.
You can compare different page versions to see what changes are in each version.
Updated about 1 year ago