Real-Time Segments for Feature Experimentation segment qualification methods for Flutter
Use the Optimizely Data Platform (ODP) Real-Time Segments for Feature Experimentation segment methods to fetch external audience mapping for the user.
Prerequisites
You must enable and configure Real-Time Segments for Feature Experimentation before fetching qualified segments and checking if the user is qualified for the given audience segment.
fetchQualifiedSegments
You can fetch user segments with the user identifier of the user context.
Minimum SDK Version
v2.0.0 and higher
Description
The fetchQualifiedSegments
method retrieves the external audience mapping for a specific user from the Optimizely Data Platform (ODP) server. The Optimizely Feature Experimentation Flutter SDK provides a synchronous and asynchronous version of the fetchQualifiedSegments
method. The caller is blocked until the synchronous fetch is completed.
fetchQualfiedSegments
is a method of the UserContext
object. See OptimizelyUserContext
for details.
Parameter
The following table describes the parameter for the fetchQualifiedSegments
method:
Parameter | Type | Description |
---|---|---|
options (optional) | Set<OptimizelySegmentOption> | A set of options for fetching qualified segments from ODP. |
Returns
The fetchQualifiedSegments
method returns true
if the qualified segments array in the user context was updated.
Note
You can read and write directly to the qualified segments array. This lets you bypass the remote fetching process from ODP or utilize your own fetching service. This can be helpful when testing or debugging.
Example fetchQualifiedSegments
The following is an example of calling the fetchQualifiedSegments
method and accessing the returned completion object:
Map<String, dynamic> attributes = {"app_version": "1.3.2"};
var user = await flutterSDK.createUserContext(
userId: "user123", attributes: attributes);
// Without segment option
var resp = await user!.fetchQualifiedSegments();
if (resp.success) {
var decision = await user.decide("flag1");
await user.trackEvent("purchase_event");
} else {
print(resp.reason);
}
// With segment options
Set<OptimizelySegmentOption> odpSegmentOptions = {
OptimizelySegmentOption.ignoreCache,
OptimizelySegmentOption.resetCache
};
response = await user.fetchQualifiedSegments(odpSegmentOptions);
if (response.success) {
var decision = await user.decide("flag1");
await user.trackEvent("purchase_event");
} else {
print(response.reason);
}
The following diagram shows the network calls between your application, the Flutter SDK, and the Optimizely Data Platform (ODP) server when calling fetchQualifiedSegments
:
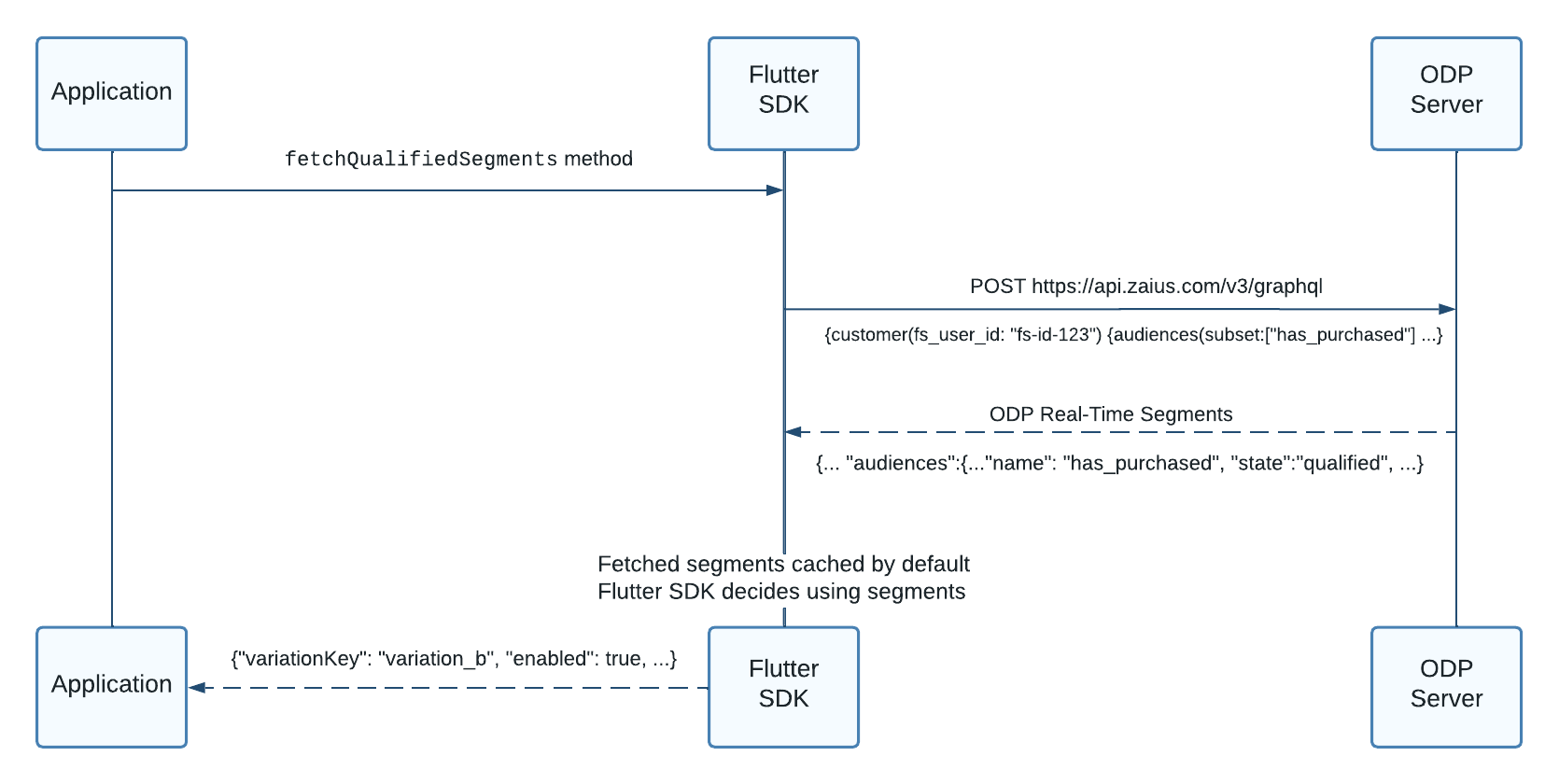
- Call the
fetchQualifiedSegments
method. - Flutter SDK makes a GraphQL call to ODP to fetch segments.
- ODP responds with segments.
- Fetched segments mapping user IDs to segments are cached. See OdpSegmentManager.
- Appropriate variations are returned for the user.
After the segments are fetched, they are cached. This means that if the same user requests the segments again (when new user contexts are created), you can retrieve the audience segment information from the cache instead of being fetched again from the remote ODP server.
The cache is used for the fetchQualifiedSegments
call. This method is called on the user context (the user context is fixed, including the real-time segments the user qualifies for).
The cache only applies when calling the fetchQualifiedSegments
call. If you set the cache timeout to 0, the cache is disabled. Optimizely uses the LRU algorithm, so the oldest record is bumped out when the maximum size is reached. If there is a cache miss upon the method call, Optimizely makes a network request.
If you want to bypass caching, add the following options to your odpSegmentOptions
array:
ignoreCache
– Bypass segments cache for lookup and saveresetCache
– Reset all segments cache
isQualifiedFor
Minimum SDK Version
v2.0.0 or higher
Description
Check if the user is qualified for the given audience segment.
Parameter
The following table describes the parameter for the isQualifiedFor
method:
Parameter | Type | Description |
---|---|---|
segment | String | The ODP audience segment name to check if the user is qualified for. |
Return
true
if the user is qualified.
Example
The following example shows whether the user is qualified for an ODP segment:
Map<String, dynamic> attributes = {"laptop_os": "mac"};
var user = await flutterSDK.createUserContext(
userId: "user123", attributes: attributes);
var response = await user!.fetchQualifiedSegments();
if (response.success) {
var result = await user.isQualifiedFor("segment1");
print(result.success);
} else {
print(response.reason);
}
Source file
The language and platform source files containing the implementation for the Flutter SDK are available on GitHub.
Updated 3 months ago