Real-Time Segments for Feature Experimentation segment qualification methods for the Android SDK
Use the Real-Time Segments for Feature Experimentation segment methods to fetch external audience mapping for the user. You can fetch user segments with the user identifier of the user context.
Prerequisites
You must enable the Real-Time Segments for Feature Experimentation integration before fetching qualified segments and checking if the user is qualified for the given audience segment.
fetchQualifiedSegments
Minimum SDK version
4.0.0
Description
You can use the fetchQualifiedSegments
method to retrieve the external audience mapping for a specific user from the ODP server. The Optimizely Feature Experimentation Android SDK provides a synchronous and asynchronous version of the fetchQualifiedSegments
method. The qualified segments are cached.
- The caller is blocked until the synchronous fetch is completed.
- The caller of asynchronous API will not be blocked.
fetchQualfiedSegments
is a method of the UserContext
object. See OptimizelyUserContext for details.
Parameters
The following table describes the parameters for the fetchQualifiedSegments
method:
Parameter | Type | Description |
---|---|---|
options (optional) | String | A set of options for fetching qualified segments. |
completion (for asynchronous calls) | Callback function | A completion handler to be called with the fetch result. |
Returns – Synchronous call
The fetchQualifiedSegments
synchronous method returns true
if the qualified segments array in the user context was updated.
Returns – Asynchronous call
- If the fetch completes successfully, the Android SDK will update the qualified segments array in the OptimizelyUserContextand then call the completion handler with a success status.
- If the fetch fails, the SDK will call the handler with a failure status.
Note
You can read and write directly to the qualified segments array. This lets you bypass the remote fetching process from Optimizely Data Platform (ODP) or utilize your own fetching service. This can be helpful when testing or debugging.
Example fetchQualifiedSegments
The following is an example of calling the fetchQualifiedSegments
method and accessing the returned completion object.
Kotlin example
val attributes: MutableMap<String, Any> = HashMap()
attributes.put("app_version", "1.3.2")
val user = optimizelyClient.createUserContext("user123", attributes)
// Without segment option
user!!.fetchQualifiedSegments { isFetchSuccessful: Boolean? ->
println(isFetchSuccessful)
val decision = user.decide("flag1")
user.trackEvent("purchase_event")
}
// With segment options
val odpSegmentOptions: MutableList<ODPSegmentOption> = ArrayList()
odpSegmentOptions.add(ODPSegmentOption.IGNORE_CACHE)
odpSegmentOptions.add(ODPSegmentOption.RESET_CACHE)
user.fetchQualifiedSegments(
{ isFetchSuccessful: Boolean? ->
println(isFetchSuccessful)
val decision = user.decide("flag1")
user.trackEvent("purchase_event")
},
odpSegmentOptions
)
val attributes: MutableMap<String, Any> = HashMap()
attributes.put("app_version", "1.3.2")
// Method 1 create with user id
val userWithUserId = optimizelyClient.createUserContext("user123", attributes)
// Without segment option
val response = userWithUserId!!.fetchQualifiedSegments()
// With segment options
val odpSegmentOptions: MutableList<ODPSegmentOption> = ArrayList()
odpSegmentOptions.add(ODPSegmentOption.IGNORE_CACHE)
odpSegmentOptions.add(ODPSegmentOption.RESET_CACHE)
val response = userWithUserId!!.fetchQualifiedSegments(odpSegmentOptions)
val decision = userWithUserId!!.decide("flag1")
userWithUserId!!.trackEvent("myevent")
// Method 2 create OptimizelyUserContext with auto-generated VUID instead of user id
val userWithVuid = optimizelyClient.createUserContext(attributes)
// Without segment option
val response = userWithVuid!!.fetchQualifiedSegments()
// With segment option
val response = userWithVuid!!.fetchQualifiedSegments()
val odpSegmentOptions: MutableList<ODPSegmentOption> = ArrayList()
odpSegmentOptions.add(ODPSegmentOption.IGNORE_CACHE)
odpSegmentOptions.add(ODPSegmentOption.RESET_CACHE)
val response = userWithVuid.fetchQualifiedSegments(odpSegmentOptions)
val decision = userWithVuid.decide("flag1")
userWithVuid.trackEvent("myevent")
Android example
Map<String, Object> attributes = new HashMap<>();
attributes.put("app_version", 1.3.2");
OptimizelyUserContext user = optimizelyClient.createUserContext("user123", attributes);
// Without segment option
Boolean response = user.fetchQualifiedSegments((Boolean isFetchSuccessful) -> {
System.out.println(isFetchSuccessful);
OptimizelyDecision decision = user.decide("flag1");
user.trackEvent("purchase_event");
});
// With segment options
List<ODPSegmentOption> odpSegmentOptions = new ArrayList<>();
odpSegmentOptions.add(ODPSegmentOption.IGNORE_CACHE);
odpSegmentOptions.add(ODPSegmentOption.RESET_CACHE);
user.fetchQualifiedSegments((Boolean isFetchSuccessful) -> {
System.out.println(isFetchSuccessful);
OptimizelyDecision decision = user.decide("flag1");
user.trackEvent("purchase_event");
},
odpSegmentOptions);
Map<String, Object> attributes = new HashMap<>();
attributes.put("app_version", "1.3.2");
// Method 1 create with user id
OptimizelyUserContext userWithUserId = optimizelyClient.createUserContext("user123", attributes);
// Without segment option
Boolean response = userWithUserId.fetchQualifiedSegments();
// With segment options
List<ODPSegmentOption> odpSegmentOptions = new ArrayList<>();
odpSegmentOptions.add(ODPSegmentOption.IGNORE_CACHE);
odpSegmentOptions.add(ODPSegmentOption.RESET_CACHE);
Boolean response = userWithUserId.fetchQualifiedSegments(odpSegmentOptions);
OptimizelyDecision decision = userWithUserId.decide("flag1");
userWithUserId.trackEvent("myevent");
// Method 2 create OptimizelyUserContext with auto-generated VUID instead of user id
OptimizelyUserContext userWithVuid = optimizelyClient.createUserContext(attributes);
// Without segment option
Boolean response = userWithVuid.fetchQualifiedSegments();
// With segment options
List<ODPSegmentOption> odpSegmentOptions = new ArrayList<>();
odpSegmentOptions.add(ODPSegmentOption.IGNORE_CACHE);
odpSegmentOptions.add(ODPSegmentOption.RESET_CACHE);
Boolean response = userWithVuid.fetchQualifiedSegments(odpSegmentOptions);
OptimizelyDecision decision = userWithVuid.decide("flag1");
userWithVuid.trackEvent("myevent");
The following diagram shows the network calls between your application, the Android SDK, and the Optimizely Data Platform (ODP) server when calling fetchQualifiedSegments
:
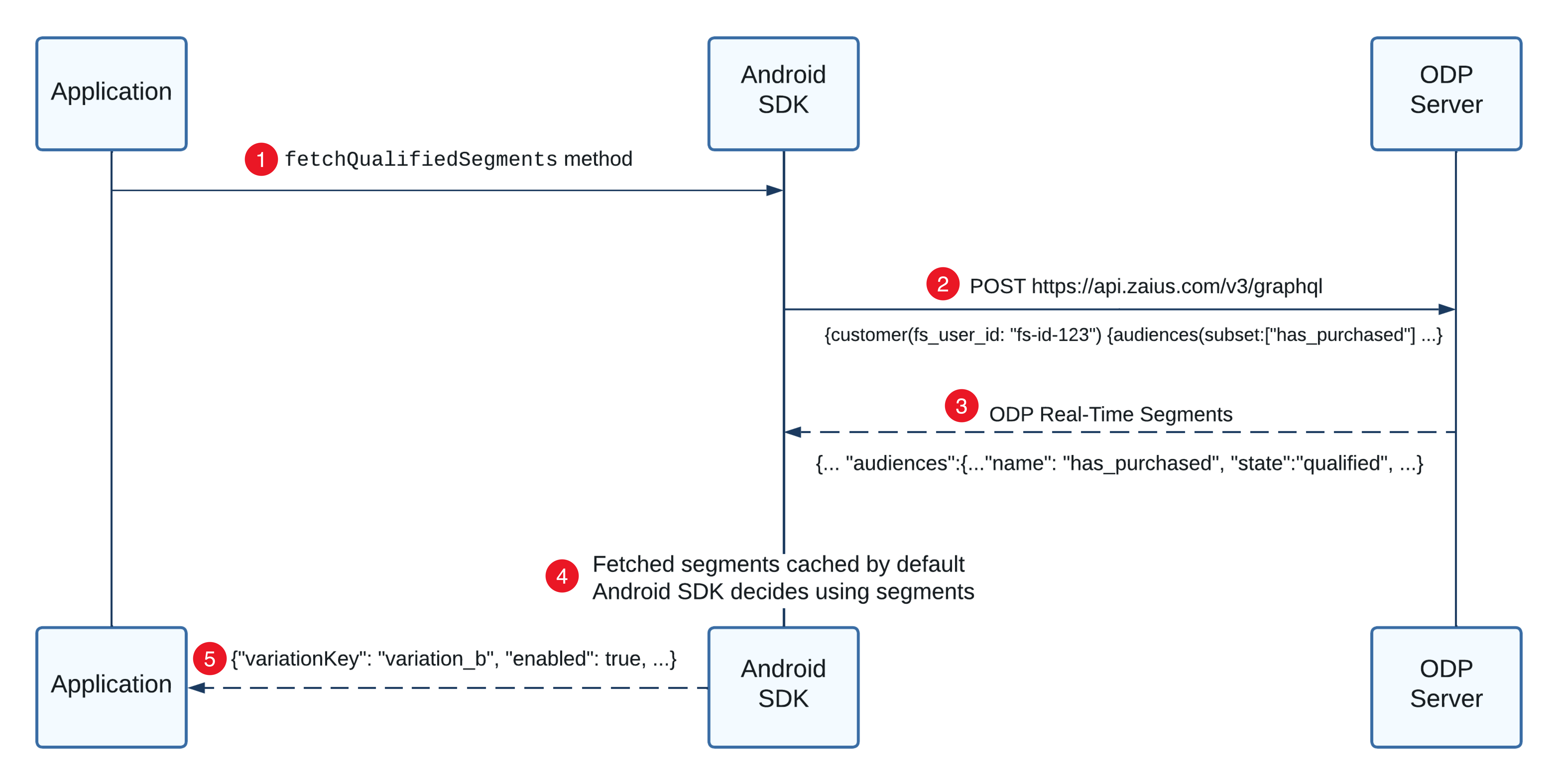
- Call
fetchQualifiedSegments
method. - Android SDK makes a GraphQL call to ODP to fetch segments.
- ODP responds with segments.
- Fetched segments mapping user IDs to segments are cached. See OdpSegmentManager.
- Appropriate variations are returned for the user.
OdpSegmentManager
When the segments are fetched, they are cached. This means that if the same user requests the segments again (when new user contexts are created), the audience segment information can be retrieved from the cache instead of being fetched from the remote ODP server.
The cache is used for the fetchQualifiedSegment
call. This method is called on the user context (the user context is fixed, including the real-time segments the user qualifies for).
The cache only applies when calling the fetchQualifiedSegments
call. If you set the cache timeout to 0, the cache is disabled. Optimizely uses the LRU algorithm, so the oldest record is bumped out when the maximum size is reached. If there is a cache miss upon the method call, Optimizely makes a network request.
The Android SDK has an OdpSegmentManager
module, which manages a segments cache that is shared for all user contexts. The cache is in memory (not persistent), so it is reset when the application is terminated or the device is rebooted.
Specifics about the cache:
- Stores a mapping of user IDs to segments.
- The Android SDK calls the ODP server on cache miss or expiration.
- Uses least recently used (LRU).
- Expires on timeout.
- Both cache size and timeout are configurable (default values provided).
- Is enabled by default and can be disabled.
If you would like to bypass caching, you can add the following options to your odpSegmentOptions
array:
- ignoreCache – Bypass segments cache for lookup and save.
- resetCache – Reset all segments cache.
See the Kotlin and Android code samples under the "With segment options" comment to see how to call fetchQualifiedSegments
with caching options.
isQualifiedFor
Minimum SDK Version
4.0.0
Description
Check if the user is qualified for the given audience segment.
Parameters
The following table describes the parameters for the isQualifiedFor
method:
Parameter | Type | Description |
---|---|---|
segment | String | The audience segment name to check if the user is qualified for. |
Returns
true
if the user is qualified.
Examples
The following is an example of whether or not the user is qualified for a segment:
val attributes: MutableMap<String, Any> = HashMap()
attributes.put("mobile_os", "ios")
val user: OptimizelyUserContext = optimizely.createUserContext("user123", attributes)
val response = user.fetchQualifiedSegments()
val isQualified = user.isQualifiedFor("segment1")
Map<String, Object> attributes = new HashMap<>();
attributes.put("mobile_os", "ios");
OptimizelyUserContext user = optimizely.createUserContext("user123", attributes);
Boolean response = user.fetchQualifiedSegments();
Boolean isQualified = user.isQualifiedFor("segment1");
Source files
The language and platform source files containing the implementation for Android are available on GitHub.
Updated 8 months ago