Configure Contentful
Use Contentful to manage the content of your Optimizely Feature Experimentation experiment.
Note
See Optimizely's Third-Party Add-Ons & Platform Integration Terms.
The Contentful and Optimizely integration lets you experiment with structured content while minimizing dependency on a developer. After setting up, you can configure and run experiments in Optimizely Feature Experimentation and choose what content from Contentful to show visitors for each variation. For help setting up the integration, see the Contentful integration demo walkthrough.
Prerequisites
- A Contentful account with a space for your website.
- An Optimizely account with a Feature Experimentation project.
- A developer to integrate the initial solution with your front end.
Terminology
Reference fields
A reference field is Contentful-specific. It is a field within a content type that does not hold its value but instead points to one or more entries. The following image has a content type called Page
. Page
has a reference field called component
. The component
reference field is a list of items (content types, such as Hero, Featured, and CTA) found on a page.
Variation containers
A variation container is a content type created by Optimizely that is used instead of a value in a reference field. In the following image, the Call To Action (CTA) entry replaces a variation container that holds two different CTAs, which is how Optimizely transforms your content model and prepares it for experimentation.
Install the Optimizely app in Contentful
-
From the Contentful app Marketplace, select Optimizely.
-
Click Install.
-
Sign in and authorize access to your account to connect it with Contentful.
-
Select an Optimizely Feature Experimentation project you want to use for your experiments.
Note
If you do not see a list of projects, you do not have any projects configured in Optimizely. You must create a project and add an A/B test rule before continuing.
-
Click Install.
Configure the Optimizely app
After installing the Optimizely app in Contentful, configure an experiment. The following example shows how to configure an A/B test for a CTA button on the page. To experiment with the component
, you must first activate the reference field in the Optimizely app.
To pick the page content type:
- Click Add content type.
- Select Page from the Content Type drop-down list.
- Select the Component checkbox.
- Click Save.
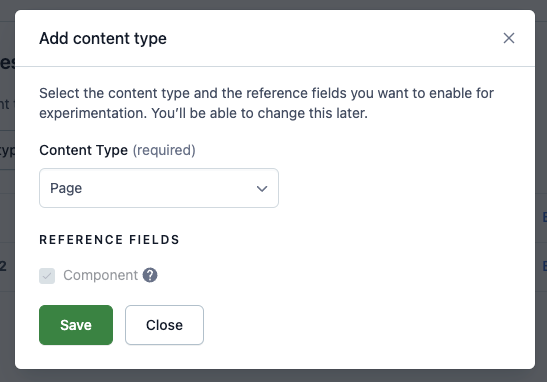
Example usage
After installing and configuring the Optimizely app, you can edit your content to use the variation container.
In the Optimizely app interface in Contentful:
-
Add a variation container to your component.
-
In the variation container, click Create entry and link for the variation.
-
Select the CTA experiment from the drop-down list.
-
Enter Buy Now! in the CTA field.
-
Click Publish.
-
Return to the variation and click Create entry and link for the control.
-
Select the CTA experiment from the drop-down list
-
Enter 20% Off!.
-
Click Publish.
-
Publish the variation container.
-
Click Publish changes to publish your page.
You can swap out values and turn them into variation containers to change any reference field used to display content and change it into a variation container that displays content based on an experiment.
How the Optimizely App changes the Contentful API response
The Optimizely app creates a content type: the variation container. Below is the CTA example that says, "Buy now!":
The Optimizely app in Contentful transforms the same content model. Where you originally received the CTA of Buy Now!, you receive a variation container holding the possible CTAs:
The variation container is a content type that nests the possible values for the CTA and holds metadata about the Optimizely experiment and the variation names.
Important
If you use a custom content model for experimentation with Optimizely other than the default variation container created by the Optimizely app (for instance, you have duplicated the variation container content model and are using this custom content model for testing), your custom content model will not immediately work with the Optimizely app.
To ensure your custom content model functions properly, add the following fields to the content model:
- flagKey
- environmentKey
- revisionId
Use Optimizely to pick the right variation
The following example uses a pseudo-backend that takes the Contentful API response and uses the Optimizely Feature Experimentation SDK to determine which variation to show the user.
First, look at the variation container JSON response from Contentful. The content type exposes a meta
, variations
, and experimentKey
property:
{
"sys": {
"space": { ... },
"id": "41nZggHEplcBOsrPXLOEU",
"type": "Entry",
"createdAt": "2019-07-17T14:32:24.306Z",
"updatedAt": "2019-07-17T14:32:24.306Z",
"environment": { ... },
"revision": 1,
"contentType": {
"sys": {
"type": "Link",
"linkType": "ContentType",
"id": "variationContainer"
}
},
"locale": "en-US"
},
"fields": {
"experimentTitle": "CTA Variation Experiment",
"experimentId": "15249150297",
"meta": {
"control": "2hoYOxnZVV3Imvry1DhmtG",
"variation": "5IlB5PCsca3zZnzlVd7WvI"
},
"variations": [
{
"sys": {
"type": "Link",
"linkType": "Entry",
"id": "2hoYOxnZVV3Imvry1DhmtG"
}
},
{
"sys": {
"type": "Link",
"linkType": "Entry",
"id": "5IlB5PCsca3zZnzlVd7WvI"
}
}
],
"experimentKey": "CTA_test",
“flagKey”: “homepage_flag”,
“Environment”: “production”
}
}
Before choosing which entry to pick from the variation container, you must see how to get the right variation from Optimizely. The following is an example with a hardcoded test to get a variation for a user:
import { createInstance } from '@optimizely/optimizely-sdk';
import datafile from './optimizelyDataFile';
// optimizelyClientInstance can be used immediately with the given datafile,
// but will download the latest datafile and update itself
const optimizelyClient = createInstance({
sdkKey: '<Your_SDK_Key>',
datafile,
});
// Create a user for which you can make flag decisions and track events
const userContext = optimizelyClient.createUserContext('<USER_ID>');
// Use Decide methods to return flag decisions for a user (includes variation).
const decision = userContext.decide('homepage_flag')
// Get variation key for decision
const variationKey = decision['variationKey'];
Optimizely determined that this user sees the variation. You can get this value from the variation container's meta
field.
The following code sample includes pulling the variation container itself and using its values to populate the Optimizely experiment:
import { createInstance } from '@optimizely/optimizely-sdk';
import datafile from './optimizelyDataFile';
// optimizelyClientInstance can be used immediately with the given datafile, but
// will download the latest datafile and update itself
const optimizelyClient = createInstance({
sdkKey: '<Your_SDK_Key>',
datafile,
});
// Create a user for which you can make flag decisions and track events
const userContext = optimizelyClient.createUserContext('<USER_ID>');
// The id of the variation container from the previous JSON sample.
// In a real-world implementation the ID would be set dynamically, for example through a slug.
const variationEntry = await sdk.getEntry('41nZggHEplcBOsrPXLOEU');
// Use Decide methods to return flag decisions for a user (includes variation).
const decision = userContext.decide(variationEntry.flagKey)
// Get variation key for decision
const variationKey = decision['variationKey'];
// ctaEntryId 5IlB5PCsca3zZnzlVd7WvI maps to experiment treatment variation
const ctaEntryId = variationEntry.meta[variationKey];
You now have the entryId
of the CTA content block you want to display; the content should show 20% off!. You can make minimal changes to your display logic to show the correct entry.
The example uses <USER_ID>
as a placeholder value for a unique user ID. You must identify the current user so Optimizely can determine which variation to place them into. This ID should stick with the user and properly identify them throughout their sessions. If you use a random number as an ID, your A/B test will not work correctly, making the results meaningless. A datafile is used in the example above to create the Optimizely client.
When setting up your experiment in Feature Experimentation, you must create a flag first. You can create an A/B test by adding a rule to the flag. When launching your Contentful experiment, activate the feature flag and individual A/B test flag rule in Feature Experimentation. If you added multiple A/B test flag rules to a single flag and want to use one of these A/B tests with Contentful, you should understand the interaction between flag rules.
Contentful exposes only published content in the variation container. Using a Contentful environment, you can test how a variation container works before promoting your experiment to production.
Questions and answers
What do I do after an experiment is over?
You can manually remove the variation container and replace it with the value that has won the experiment. This prevents you from triggering consumption metrics in Optimizely when delivering one winning variation. You do not need to rely on Optimizely to serve the right content.
Does the Optimizely app in Contentful support both Feature Experimentation and legacy Full Stack?
Yes. The Optimizely app in Contentful supports legacy Full Stack Experimentation and Feature Experimentation projects.
When I upgrade from legacy Full Stack Experimentation to Feature Experimentation, what happens to the experiments that I am using in Contentful?
Legacy Full Stack experiments that you previously set up in Contentful continue to run and serve content to visitors on your site without issue. You do not need code changes when migrating your legacy Full Stack project to Feature Experimentation.
After migrating your project to Feature Experimentation, the experiments, variation containers, and related content you previously set up in Contentful pre-migration are unaffected. See Optimizely's end-user documentation on Migration considerations for the Contentful integration.
If I already use the Optimizely app in Contentful with legacy Full Stack Experimentation, how does the experiment setup differ when using it with Feature Experimentation?
There are some important differences in how you set up your experiment in legacy Full Stack Experimentation and Feature Experimentation:
- When setting up your experiment in Feature Experimentation, you must first create a flag. You can then add a flag rule to this flag to create an A/B test. When launching your Contentful experiment, activate the feature flag and individual A/B test flag rule in Feature Experimentation.
- If you are migrating from legacy Full Stack Experimentation to Feature Experimentation, Optimizely converts the A/B tests you previously set up in Full Stack Experimentation into A/B test rules under a flag. See Migrate legacy A/B test and Migration considerations for the Contentful integration in Optimizely's end-user documentation.
If I set up multiple experiments per flag in Feature Experimentation, will this affect how I can use experiments in Contentful?
If you added multiple A/B test flag rules to a single flag and want to use one of these A/B tests with Contentful, you should understand the interaction between flag rules.
For example, you created an A/B test in Feature Experimentation and want to use it in Contentful. Suppose you added other flag rules in the same ruleset for the same flag, and these other rules are prioritized higher than the A/B test you selected in Contentful. In that case, users may not be exposed to the Contentful experiment because they have already met the higher-priority audience conditions for another flag rule. Feature Experimentation intentionally did not expose the user to other A/B tests in the ruleset.
How does the Optimizely app affect my Contentful environment?
The app needs to create a content type called the variation container. The variation container is a custom type that uses a custom editor interface inside the web app and collates data from Optimizely and Contentful.
Where can I activate my experiments?
Contentful controls the content, and Optimizely controls the experiment. The Optimizely app provides links on the sidebar of Contentful to Optimizely for access to your experiments, where you can set up and launch experiments.
Why is my app configuration not being saved?
Click Save in the Optimizely app to save the full configuration.
Why does one or more of my variations not show up?
When you pick variations inside a variation container, you must ensure they are published and not a draft. Furthermore, you must ensure that your variation container is published to display in the API response.
How can I define a fallback or default value for my experiment?
A default value or fallback is usually the control variation. If you have a certain audience to which you do not want to expose the experiment, ensure your front end can handle the scenario of falling back to your control group.
How do I target a certain audience?
Optimizely targets an audience when you provide a unique value (user ID) to let Optimizely know who is currently seeing an experiment. If you need a custom solution, provide more data points to target your audience correctly. See the previous question about defining a default or fallback value to exclude an audience.
Does uninstalling the Optimizely app in Contentful break my experiments?
Your existing experiments will still run. Contentful never tells Optimizely what to do with your experiments. However, the editor's experience dealing with experiments inside the Contentful Web App is highly degraded. Variation containers will still exist, but their custom editor is uninstalled.
Why is the variation container not an option in my reference field?
Your reference field may have specific validations enabled. You must enable the variation container as a valid content type for that field, which you can do in the app or find the specific content type and change the validations to include the variation container.
Additional information
See the following:
Updated about 2 months ago