Create feature flags
Describes how to create and implement feature flags in Optimizely Feature Experimentation.
Feature flags, also called feature toggles or permission toggles, let you turn certain functionality on and off without deploying code. They give you full control over the lifecycle of a feature. With feature flags, you can:
- Release code without exposing it to users by keeping the flag off.
- Gradually roll out a feature to a portion of your user base.
- Validate functionality and performance before a full release.
When a collaborator creates a flag, they are automatically assigned the Admin role for that flag.
Permissions required to create a flag
To create a flag in Feature Experimentation, you must have access to an environment in the project.
When a collaborator creates a flag, they are automatically assigned the Admin role for that flag.
Note
To create a flag or audience, a collaborator must have Editor or above access in at least one environment.
If your project does not use granular permissions, the collaborator must have an Editor or above project role.
Create a flag
Go to Flags > Create New Flag.
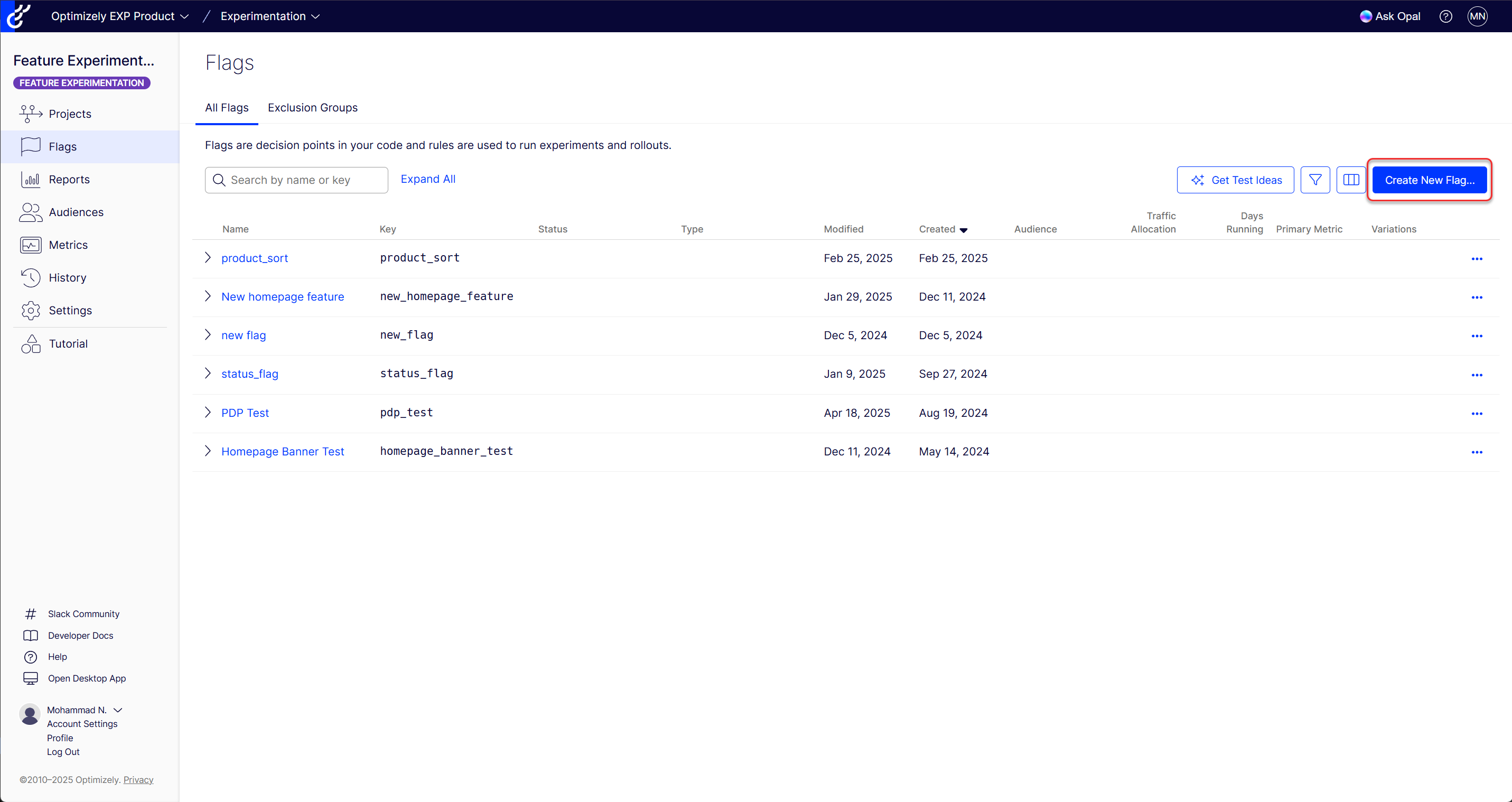
-
In the Name field, enter a unique name. Valid keys contain alphanumeric characters, hyphens, and underscores, are limited to 64 characters, and cannot contain spaces.
Note
Feature Experimentation automatically assigns a key to the flag based on this name. You use the flag key to implement the flag in your application's code.
Flag keys cannot be changed after they are created.
-
(Optional) Enter a Description.
-
Click Create Flag to save your flag.
- Feature Experimentation automatically creates a read-only
flag_off
variation to use as the control in experiments. - Feature Experimentation automatically creates two environments, Production and Development.
- Feature Experimentation automatically creates a read-only
Get test ideas with Optimizely Opal
Important
Administrators must grant users access to Optimizely Opal.
Opal is enabled by default for eligible customers, but users do not have access until an administrator enables it for each individual using Opti ID. For steps, see Get started with Optimizely Opal for Admins.
You can get potential test ideas with Optimizely Opal. Opal incorporates best practices developed internally within Optimizely to provide potential experiment ideas.
Note
If you use Opti ID, administrators can turn off generative AI in the Opti ID Admin Center. See Turn generative AI off across Optimizely applications.
Otherwise, contact Optimizely Support to turn off AI features.
-
Click Flags.
-
Click Get Test Ideas. The Optimizely Opal chat displays with the following message "Please provide a website URL or image and I'll start my analysis!"
-
Enter the URL or upload an image, then click Submit.
-
Optimizely Opal analyzes a screenshot of the provided URL or image and then provides some test ideas for improvement. Improvements can include clarity of the value proposition, call-to-action, readability, and so on.
Implement the feature flag
Note
Before implementing the flag, decide how to pass user identifiers to Feature Experimentation. This ensures each user has a consistent experience of the flag being on or off.
See Handle user ids for information.
After you create the flag in the Optimizely Feature Experimentation dashboard, you need to integrate it with your application code. Use the Decide
method to enable or disable the flag for a user.
// Decide if user sees a feature flag variation
user := optimizely.CreateUserContext("user123", map[string]interface{}{"logged_in": true})
decision := user.Decide("flag_1", nil)
enabled := decision.Enabled
// Decide if user sees a feature flag variation
var user = optimizely.CreateUserContext("user123", new UserAttributes { { "logged_in", true } });
var decision = user.Decide("flag_1");
var enabled = decision.Enabled;
// Decide if user sees a feature flag variation
var user = await flutterSDK.createUserContext(userId: "user123");
var decideResponse = await user.decide("product_sort");
var enabled = decision.enabled;
// Decide if user sees a feature flag variation
OptimizelyUserContext user = optimizely.createUserContext("user123", new HashMap<String, Object>() { { put("logged_in", true); } });
OptimizelyDecision decision = user.decide("flag_1");
Boolean enabled = decision.getEnabled();
// Decide if user sees a feature flag variation
const user = optimizely.createUserContext('user123', { logged_in: true });
const decision = user.decide('flag_1');
const enabled = decision.enabled;
// Decide if user sees a feature flag variation
$user = $optimizely->createUserContext('user123', ['logged_in' => true]);
$decision = $user->decide('flag_1');
$enabled = $decision->getEnabled();
# Decide if user sees a feature flag variation
user = optimizely.create_user_context("user123", {"logged_in": True})
decision = user.decide("flag_1")
enabled = decision.enabled
// Decide if user sees a feature flag variation
var decision = useDecision('flag_1', null, { overrideUserAttributes: { logged_in: true }});
var enabled = decision.enabled;
# Decide if user sees a feature flag variation
user = optimizely_client.create_user_context('user123', {'logged_in' => true})
decision = user.decide('flag_1')
decision.enabled
// Decide if user sees a feature flag variation
let user = optimizely.createUserContext(userId: "user123", attributes: ["logged_in":true])
let decision = user.decide(key: "flag_1")
let enabled = decision.enabled
For more detailed examples of each SDK, see the following:
- Android SDK example usage
- Go SDK example usage
- C# SDK example usage
- Flutter SDK example usage
- Java SDK example usage
- JavaScript example usage – SDK versions 6.0.0 and later.
- Javascript (Browser) SDK example usage – SDK versions 5.3.5 and earlier.
- JavaScript (Node) SDK example usage – SDK versions 5.3.5 and earlier.
- PHP SDK example usage
- Python SDK example usage
- React SDK example usage
- React Native SDK example usage
- Ruby SDK example usage
- Swift SDK example usage
Adapt the integration code in your application. Show or hide the flag's functionality for a given user ID based on the boolean value your application receives.
The Decide
method separates code deployment from the decision to show a feature. The flag's rules determine the result. For example, it returns false if the user is assigned to the control or "off" variation in an experiment.
Next steps
To deliver feature flag configurations, see Create flag variations.
Updated about 2 months ago