Real-Time Segments for Feature Experimentation segment qualification methods for the Ruby SDK
Use the fetch_qualified_segments
method to retrieve the external audience mapping for the user from the Optimizely Data Platform (ODP) server. Use the qualified_for?
method to check if the user qualifies for the specified segment.
Prerequisites
You must enable and configure Real-Time Segments for Feature Experimentation before fetching qualified segments and checking if the user is qualified for the given audience segment.
fetch_qualified_segments
Minimum SDK Version
5.0.0
Description
You can use the fetch_qualified_segments
method to retrieve the external audience mapping for a specific user from the Optimizely Data Platform (ODP) server. The Optimizely Feature Experimentation Ruby SDK lets you make the call to ODP in a synchronous or asynchronous fashion, depending on the block parameter.
- If a block is not provided, the caller is blocked until the synchronous fetch is completed.
- If a block is provided, the caller is not blocked.
fetch_qualified_segments
is a method of the OptimizelyUserContext
object. See OptimizelyUserContext for details.
Parameters
The following table describes the parameters for the fetch_qualified_segments
method:
Parameter | Type | Description |
---|---|---|
options (optional) | String | A set of options for fetching qualified segments from ODP. |
block (optional) | Callback function | A completion handler to be called with the fetch result. |
Returns – Synchronous call
If the SDK does not have a block, thefetch_qualified_segments
method returns true
if the qualified segments array in the user context was updated.
Returns – Asynchronous call
If the SDK has a block, the fetch_qualified_segments
method fetches the segments on a new thread and returns the thread handle.
- If the fetch completes successfully, the Ruby SDK updates the qualified segments array in the user context and then calls the block with a success status.
- If the fetch fails, the SDK calls the block with a failure status.
If the Ruby SDK does not find an ODP audience in the datafile, it will return an empty qualified segments array without sending a request to the ODP server.
Note
You can read and write directly to the qualified segments array instead of calling
fetch_qualified_segments
.This lets you bypass the remote fetching process from ODP or utilize your own fetching service. This can be helpful when testing or debugging.
Example fetch_qualified_segments
call
fetch_qualified_segments
callThe code creates a user context by instantiating an OptimizelyUserContext object with a user ID "user123" and a set of attributes, which includes an "app_version" attribute with a value of "1.3.2".
The next section of the code shows the use of thefetch_qualified_segments
method to fetch all qualified segments with and without segment options.
After that, the code uses the decide
method to decide whether to show the feature flag with the key "flag1" to the user. Finally, the SDK calls the track_event
method to track a custom event called "myevent".
If a callback is provided, the fetch runs on a spawned thread and runs the callback upon completion. If no callback is provided, the fetch is synchronous.
attributes = { 'app_version' => '1.3.2' }
user = optimizely.create_user_context('user123', attributes)
# spawned thread is returned
fetch_thread = user.fetch_qualified_segments do |success|
puts success
return unless success
decision = user.decide('flag1')
user.track_event('purchase_event')
end
# thread must eventually be joined to ensure callback is run to completion
fetch_thread.join
attributes = { "app_version"=> "1.3.2" }
user = optimizely.create_user_context("user123", attributes)
# Without segment option
success = user.fetch_qualified_segments
# With segment options
odp_segment_options = [Optimizely::OptimizelyOdpOption.IGNORE_CACHE, Optimizely::OptimizelyOdpOption.RESET_CACHE]
success = user.fetch_qualified_segments(options: odp_segment_options)
if success
decision = user.decide("flag1")
user.track_event("myevent")
end
The following diagram shows the network calls between your application, the Ruby SDK, and the ODP server when calling fetch_qualified_segments
:
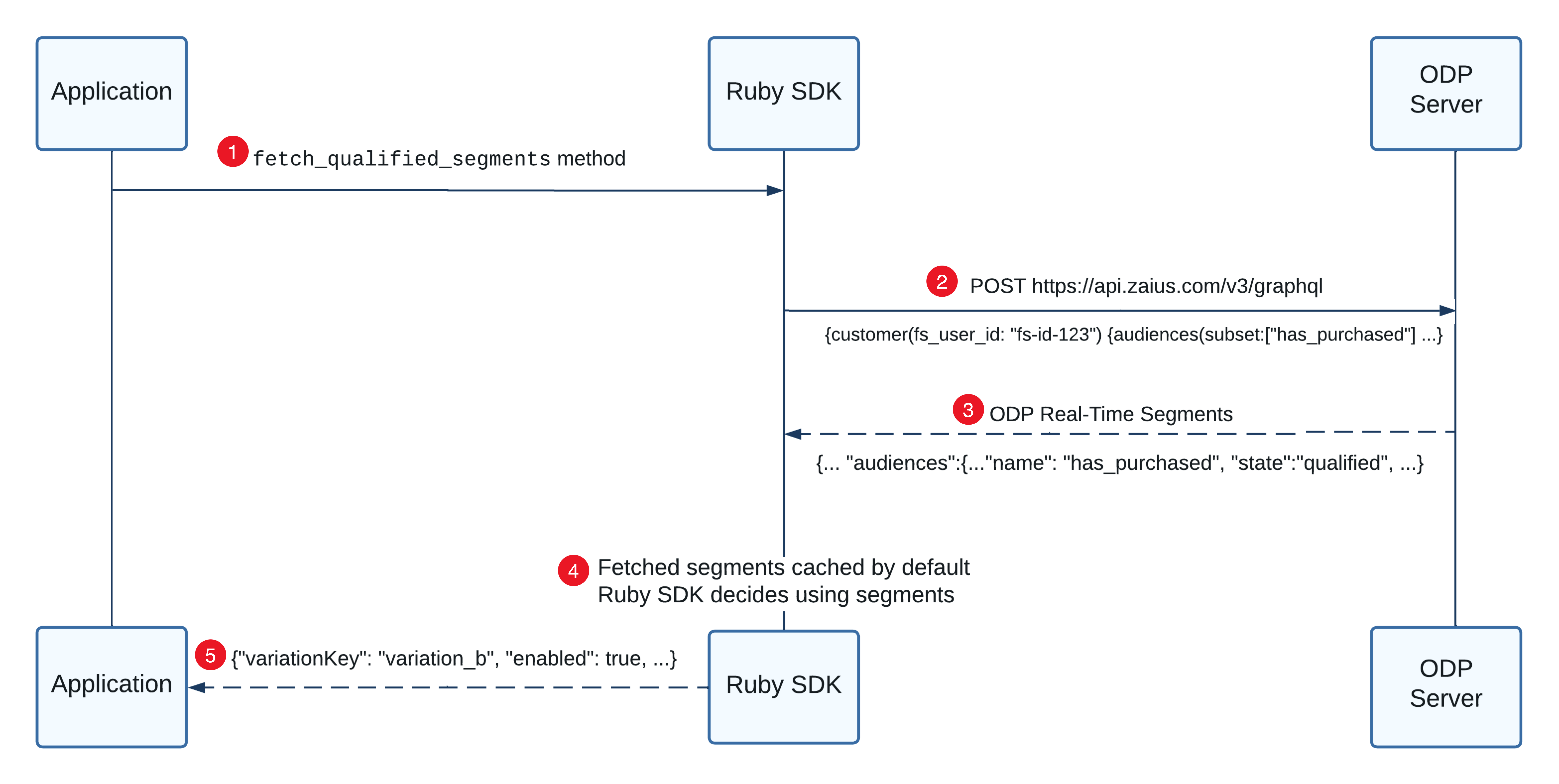
- Call the
fetch_qualified_segments
method. - Ruby SDK makes a GraphQL call to ODP to fetch segments.
- ODP responds with segments.
- Fetched segments mapping user IDs to segments are cached.
- Appropriate variations are returned for the user.
The SDK fetches the segments and then caches them. This means that if the same user is requesting the segments again (when new user contexts are created), you can retrieve the audience segment information from the cache rather than from the remote ODP server.
The cache is used for the fetch_qualified_segments
call. This method is called on the user context (the user context is fixed, including the real-time segments the user qualifies for).
The cache only applies when calling the fetch_qualified_segments
call. If you set the cache timeout to 0, the cache is disabled. Optimizely uses the LRU algorithm, so the oldest record is bumped out when the maximum size is reached. If there is a cache miss upon the method call, Optimizely makes a network request.
You can add the following options to your OptimizelyOdpOption
array to bypass caching:
- IGNORE_CACHE – Bypass segments cache for lookup and save.
- RESET_CACHE – Reset all segments cache.
qualified_for?
Minimum SDK Version
5.0.0
Description
Check if the user is qualified for the given audience segment.
Parameters
The following table describes the parameters for the qualified_for?
method:
Parameter | Type | Description |
---|---|---|
segment | String | The ODP audience segment name to check if the user is qualified for. |
Returns
true
if the user is qualified.
Examples
The following is an example of whether or not the user is qualified for an ODP segment:
attributes = {'laptop_os' => 'mac'}
user = optimizely.create_user_context('fs-id-12', attributes)
success = user.fetch_qualified_segments
qualified = user.qualified_for?('segment1');
Source files
The language and platform source files containing the implementation for Ruby are available on GitHub.
Updated 3 months ago