Real-Time Segments for Feature Experimentation segment qualification methods for the Python SDK
Use the fetch_qualified_segments
method to retrieve the external audience mapping for the user from the Optimizely Data Platform (ODP) server. Use the is_qualified_for
method to check if the user qualifies for the specified segment.
Prerequisites
You must enable and configure Real-Time Segments for Feature Experimentation before fetching qualified segments and checking if the user is qualified for the given audience segment.
fetch_qualified_segments
Minimum SDK version
5.0.0
Description
You can use the fetch_qualified_segments
method to retrieve the external audience mapping for a specific user from the Optimizely Data Platform (ODP) server. The Optimizely Feature Experimentation Python SDK lets you make the call to ODP in a synchronous or asynchronous fashion, depending on the callback parameter.
- If you do not provide a callback, the caller is blocked until the synchronous fetch is complete.
- Providing a callback does not block the caller.
fetch_qualfied_segments
is a method of the OptimizelyUserContext
object. See OptimizelyUserContext for details.
Parameters
The following table describes the parameters for the fetch_qualified_segments
method:
Parameter | Type | Description |
---|---|---|
options (optional) | list[str] | A list of options for fetching qualified segments from ODP. |
callback (optional) | Callback function | A callback function to be called with the fetch result. |
Returns – synchronous call
If you do not provide a callback, the fetch_qualified_segments
method returns True
if the qualified segments array in the user context was updated.
Returns – asynchronous call
If you provide a callback, the fetch_qualified_segments
method fetches the segments on a new thread and returns the thread handle.
- If the fetch completes successfully, the Python SDK updates the qualified segments list in the user context and then calls the callback function with a success status.
- If the fetch fails, the SDK calls the callback function with a failure status.
If the Python SDK does not find an ODP audience in the datafile, it does return an empty qualified segments array without sending a request to the ODP server.
Note
You can read and write to the qualified segments array instead of calling
fetch_qualified_segments
usingget_qualified_segments
andset_qualified_segments
.This lets you bypass the remote fetching process from ODP or utilize your own fetching service. This can be helpful when testing or debugging.
Example fetch_qualified_segments
call
fetch_qualified_segments
callattributes = { "app_version": "1.3.2" }
user = optimizely_client.create_user_context("user123", attributes)
def fetch_callback(fetch_successful):
print(fetch_successful)
if fetch_successful:
decision = user.decide("flag1")
user.track_event("purchase_event")
# spawned thread is returned
fetch_thread: threading.Thread = user.fetch_qualified_segments(fetch_callback)
# thread must eventually be joined to ensure callback is run to completion
fetch_thread.join()
attributes = { "app_version": "1.3.2" }
user = optimizely.create_user_context("user123", attributes)
# Without segment option
success = user.fetch_qualified_segments()
# With segment options
odp_segment_options = [OptimizelyOdpOption.IGNORE_CACHE, OptimizelyOdpOption.RESET_CACHE]
success = user.fetch_qualified_segments(options=odp_segment_options)
if success:
decision = user.decide("flag1")
user.track_event("myevent")
The following diagram shows the network calls between your application, the Python SDK, and the ODP server when calling fetch_qualified-segments
:
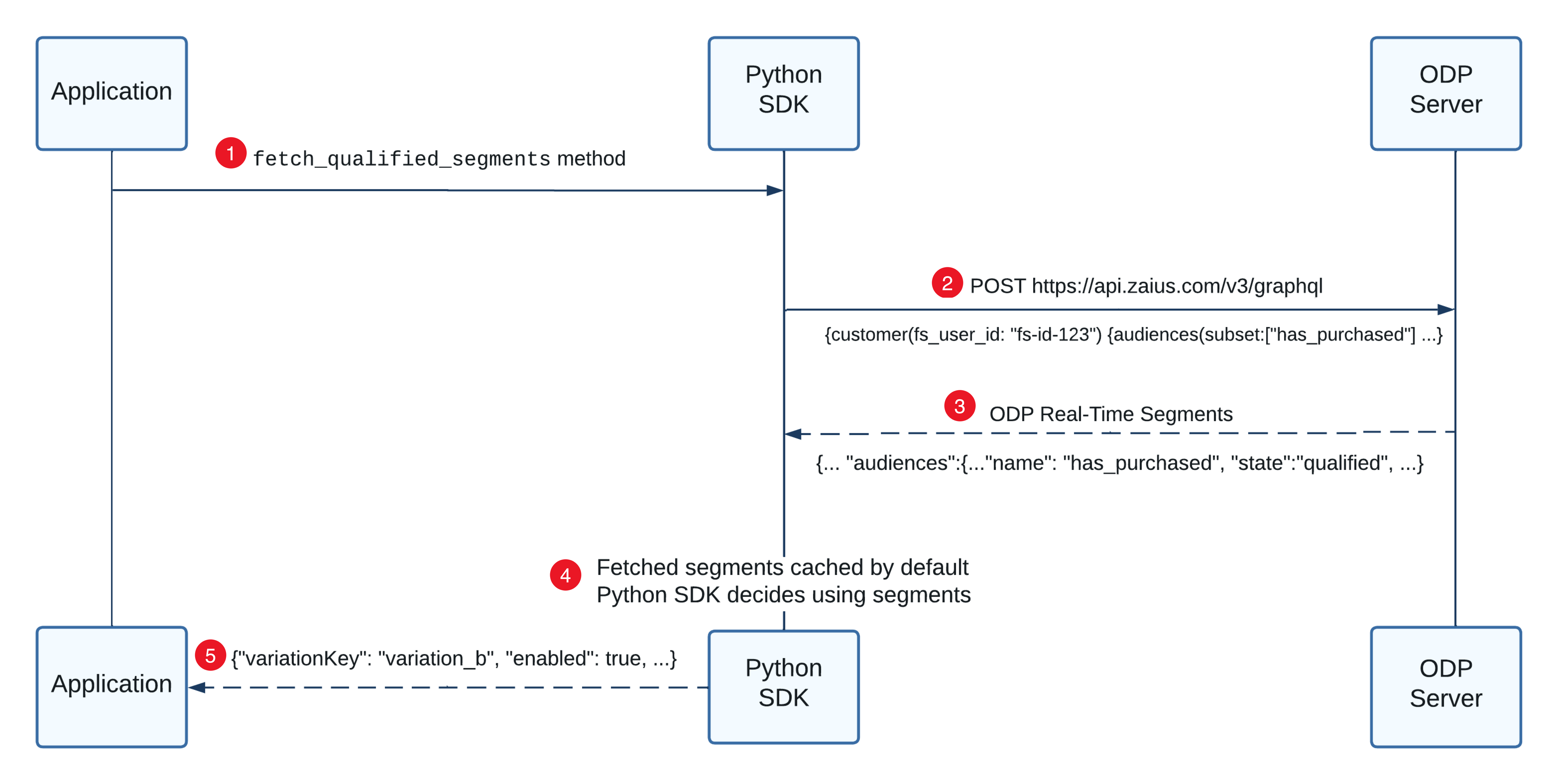
- Call the
fetch_qualified_segments
method. - The Python SDK makes a GraphQL call to ODP to fetch segments.
- ODP responds with segments.
- Fetched segments mapping user IDs to segments are cached.
- Appropriate variations are returned for the user.
After you fetch the segments, they are cached. This means that if the same user requests the segments again (when new user contexts are created), the Python SDK can retrieve the audience segment information from the cache rather than from the remote ODP server.
The cache is used for the fetch_qualified_segments
call. This method is called on the user context (the user context is fixed, including the real-time segments the user qualifies for).
The cache only applies when calling the fetch_qualified_segments
call. If you set the cache timeout to 0, the cache is disabled. Optimizely uses the LRU algorithm, so the oldest record is bumped out when the maximum size is reached. If there is a cache miss upon the method call, Optimizely makes a network request.
If you would like to bypass caching, you can add the following options to your OptimizelyOdpOption
array:
- IGNORE_CACHE – Bypass segments cache for lookup and save.
- RESET_CACHE – Reset all segments cache.
is_qualified_for
Minimum SDK version
5.0.0
Description
Check if the user is qualified for the given audience segment.
Parameters
The following table describes the parameters for the is_qualified_for
method:
Parameter | Type | Description |
---|---|---|
segment | String | The ODP audience segment name to check if the user is qualified for. |
Returns
True
if the user is qualified.
Examples
The following is an example of whether or not the user is qualified for an ODP segment:
attributes = {"laptop_os": "mac"}
user = optimizely_client.create_user_context("fs_id_12", attributes)
success = user.fetch_qualified_segments()
qualified = user.is_qualified_for("segment1")
Source files
The language and platform source files containing the implementation for Python are available on GitHub.
Updated about 1 year ago