Initialize Flutter SDK
How to initialize the Optimizely Feature Experimentation Flutter SDK.
The initializeClient
method initializes the Flutter SDK and instantiates an instance of the Optimizely Feature Experimentation SDK class, which exposes API methods like the CreateUserContext
method. Each client corresponds to the datafile that represents the state of a project for an environment. The datafile is accessible through OptimizelyConfig
.
Version
2.0.0 or higher
Description
The constructor accepts a configuration object to configure Optimizely Feature Experimentation.
Some parameters are optional because the Flutter SDK provides a default implementation, but you may want to override these for your production environments. For example, you can set up event options to manage network calls and datafile handler parameters to set up the datafile download path or interval.
Parameters
start
method parameters:
Parameter | Type | Description |
---|---|---|
sdkKey required | String | Each environment has its own SDK key. |
eventOptions optional | EventOptions | Lets you set batchSize , timeInterval and maxQueueSize of event processor.If you do not set these values, the system uses the defaults of batchSize (10), timeInterval (60) and maxQueueSize (10000). |
datafilePeriodicDownloadInterval optional | int | Sets the interval (in seconds) during which DatafileHandler attempts to update the cached datafile periodically.The minimum interval is 15 minutes (enforced by the Android JobScheduler API). If you are running iOS only, there is no minimum interval. |
datafileHostOptions optional | Map<ClientPlatform, DatafileHostOptions> | Adds support to provide custom datafileHost URL for different platforms.ClientPlatform is enum with two platform options iOS and android .DatafileHostOptions lets you set datafileHostPrefix and datafileHostSuffix .For each platform, the datafileHostSuffix format is a little different. For example,Suffix for iOS should look like: /datafiles/%@.json (%@ will automatically be substituted with the sdkKey ).Suffix for Android should look like: /datafiles/%s.json (%s will automatically be substituted with the sdkKey ). |
defaultDecideOptions optional | Set<OptimizelyDecideOption> | Sets default decide options applied to the Decide calls made during the lifetime of the Optimizely client. You can also pass options to individual Decide methods (does not override defaults). |
sdkSettings optional | SDKSettings | You can configure other SDK settings, such as the Real-Time Segments for Feature Experimentation methods with this sdkSettings construct (see the following code sample). |
// You must configure Real-Time Segments for Feature Experimentation before using the following methods.
class SDKSettings {
// The maximum size of audience segments cache (optional. default = 100). Set to zero to disable caching.
final int segmentsCacheSize;
// The timeout in seconds of audience segments cache (optional. default = 600). Set to zero to disable timeout.
final int segmentsCacheTimeoutInSecs;
// The timeout in seconds of ODP segment fetch (optional. default = 10) - OS default timeout will be used if this is set to zero.
final int timeoutForSegmentFetchInSecs;
// The timeout in seconds of ODP event dispatch (optional. default = 10) - OS default timeout will be used if this is set to zero.
final int timeoutForOdpEventInSecs;
// Set this flag to true (default = false) to disable ODP features
final bool disableOdp;
// Set this flag to true (default = false) to enable VUID feature
final bool enableVuid;
}
Returns
Instantiates an instance of the Optimizely Feature Experimentation class.
Customize ODPManager
OdpManager
contains the logic supporting Real-Time Segments for Feature Experimentation-related features, including audience segments.
Information
If necessary, to disable Real-Time Segments for Feature Experimentation altogether, set
disableOdp
totrue
.
The following SDKSettings
are optionally configurable when the Flutter SDK is initialized:
- ODP SegmentsCache size –
segmentsCacheSize
- Default – 100
- Set to 0 to disable caching.
- ODP SegmentsCache timeout (in seconds) –
segmentsCacheTimeoutInSecs
- Default – 600 secs (10 minutes)
- Set to 0 to disable timeout (never expires).
- ODP SegmentsFetch timeout –
timeoutForSegmentFetchInSecs
- Default – 10 secs
- The Operating System's (OS) default timeout is used if this is set to zero.
- ODP Event timeout –
timeoutForOdpEventInSecs
- Default – 10 secs
- OS default timeout is used if this is set to zero.
- ODP enable/disable –
disableOdp
- Default – false (enabled)
- When
disableOdp
is set totrue
, the Flutter SDK will disable ODP-related features. The Flutter SDK still creates and manages VUID regardless of this flag and supports VUID-based decisions. See anonymous users. - The Flutter SDK returns or logs an
odpNotEnabled
error when ODP is disabled and its features are requested.
- enableVUID – enableVuid
Important
Only available on the Flutter SDK version 3.0.0+. See SDK compatibility matrix.
- Default – false (disabled)
- When enabled, the Flutter SDK creates and manages VUID and supports VUID-based decisions. See anonymous users.
// You must configure Real-Time Segments for Feature Experimentation before using the following methods.
class SDKSettings {
// The maximum size of audience segments cache (optional. default = 100). Set to zero to disable caching.
final int segmentsCacheSize;
// The timeout in seconds of audience segments cache (optional. default = 600). Set to zero to disable timeout.
final int segmentsCacheTimeoutInSecs;
// The timeout in seconds of ODP segment fetch (optional. default = 10) - OS default timeout will be used if this is set to zero.
final int timeoutForSegmentFetchInSecs;
// The timeout in seconds of ODP event dispatch (optional. default = 10) - OS default timeout will be used if this is set to zero.
final int timeoutForOdpEventInSecs;
// Set this flag to true (default = false) to disable ODP features
final bool disableOdp;
}
You can provide segmentsCacheSize
and segmentsCacheTimeoutInSecs
timeout by passing these values when initializing the Flutter SDK.
const odpSettings = SDKSettings(
segmentsCacheSize: 1000,
segmentsCacheTimeoutInSecs: 30 * 60,
disableOdp: false);
var flutterSDK = OptimizelyFlutterSdk("<Your_SDK_Key>", sdkSettings: odpSettings);
var response = await flutterSDK.initializeClient();
Examples
You do not need to manage the datafile directly in the Flutter SDK. The SDK includes a datafile manager that provides support for datafile polling to automatically update the datafile at a regular interval while the application is in the foreground.
To use it complete the following:
-
Create a
OptimizelyFlutterSdk
by supplying your SDK Key and optional configuration settings. You can find the SDK key in Settings > Environments of a Feature Experimentation project. -
To start the client synchronously and use the
initializeClient
method to instantiate a client:
// Initializing Optimizely Client
var flutterSDK = OptimizelyFlutterSdk("<Your_SDK_Key>");
var response = await flutterSDK.initializeClient();
// flag decision
var user = await flutterSDK.createUserContext(userId: "<User_ID>");
var decideResponse = await user!.decide("<Flag_Key>");
Use initialization
To instantiate the Optimizely client: synchronous. This method pings the Optimizely Feature Experimentation servers for a new datafile during initialization.
During initialization, your app requests the newest datafile from the CDN servers. Requesting the newest datafile ensures that your app uses the latest project settings. It also means your app cannot instantiate a client until it downloads a new datafile, discovers the datafile has not changed, or the request times out.
Initializing a client synchronously causes the manager to first attempt to download the newest datafile. The network activity causes an initialization to take longer to complete.
If the network request returns an error, such as when network connectivity is unreliable or if the manager discovers that the cached datafile is identical to the newest datafile, the Optimizely Feature Experimentation manager searches for a cached datafile. If one is available, the manager uses the datafile to complete the client initialization; otherwise initialization fails. If the manager discovers there is an updated datafile that differs from the cached datafile, the manager downloads the new datafile and uses it to initialize the client.
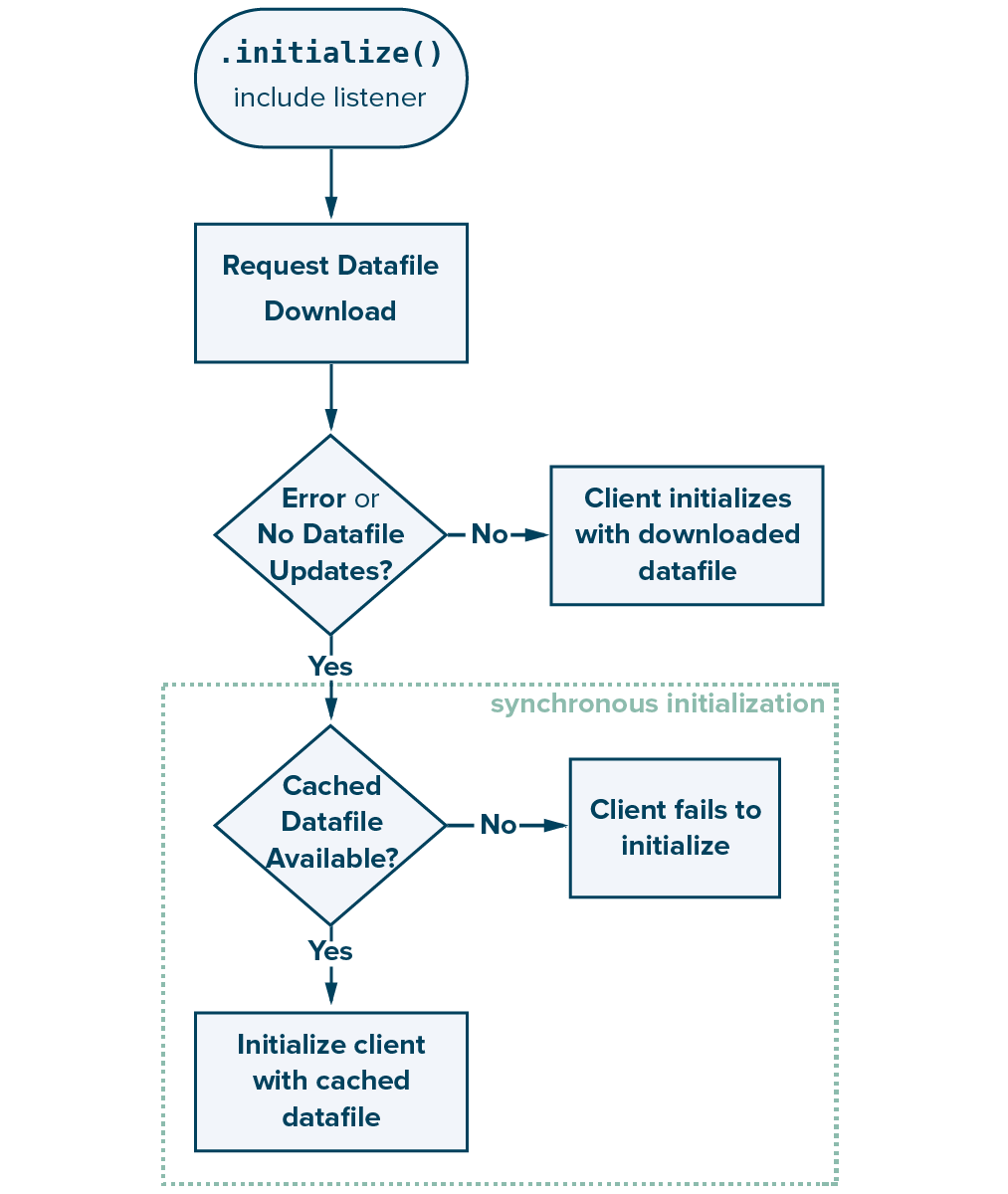
To initialize an OptimizelyClient
object, call flutterSDK.initializeClient()
.
Configure datafile polling
Datafile polling is the process of checking for and downloading a new datafile. After the manager tries to pull the latest datafile from the CDN servers, the Optimizely manager periodically checks for and pulls a new datafile at the time interval you set during its initialization.
Datafile polling is enabled by default. You can set a non-zero interval value to increase or decrease the polling interval. This value is the number of seconds the manager waits between datafile polling attempts.
// Datafile update interval can be configured when the SDK is configured
// Background datafile update is disabled if periodicDownloadInterval is zero
var flutterSDK = OptimizelyFlutterSdk("<Your_SDK_Key>", datafilePeriodicDownloadInterval: 15 * 60);
// every 15 minutes
Usage notes
-
The minimum polling interval is 15 minutes while the app is open. You cannot set a shorter polling interval.
-
The Optimizely manager only checks for new datafiles when the SDK is active, and the app runs on iOS, tvOS, or Android.
-
The Flutter SDK automatically updates when it detects a new datafile to ensure you are always working with the latest datafile. If you want to receive a notification when the project updates, register for a datafile change through the datafile change notification listener. You can also register for datafile change notifications using the
UpdateConfigNotification
method.
flutterSDK.addConfigUpdateNotificationListener((msg) {
print("got datafile change");
});
Dispose of the client
For effective resource management with the Optimizely Flutter SDK, you must properly close the Optimizely client instance when it is no longer needed. This is done by calling .close
.
The .close()
method ensures that the processes and queues associated with the instance are properly released. This is essential for preventing memory leaks and ensuring that the application runs efficiently, especially in environments where resources are limited or in applications that create and dispose of many instances over their lifecycle.
See Close Optimizely Feature Experimentation Flutter SDK on application exit.
More sample code
// Initializing OptimizelyClient without optional parameters
var flutterSDK = OptimizelyFlutterSdk("<Your_SDK_Key>");
var response = await flutterSDK.initializeClient();
// flag decision
var user = await flutterSDK.createUserContext(userId: "<User_ID>");
var decideResponse = await user!.decide("<Flag_Key>");
// Initializing OptimizelyClient with optional datafilePeriodicDownloadInterval
var flutterSDK =
OptimizelyFlutterSdk(sdkKey, datafilePeriodicDownloadInterval: 16 * 60);
var response = await flutterSDK.initializeClient();
// flag decision
var user = await flutterSDK.createUserContext(userId: "<User_ID>");
var decideResponse = await user!.decide("<Flag_Key>");
Source files
The language and platform source files containing the implementation for Flutter are available on GitHub.
Updated 5 months ago