Initialize the Android SDK
How to initialize the Optimizely Feature Experimentation Android SDK in your application.
Use the instantiate
method to initialize the Android SDK and instantiate an instance of the Optimizely client class that exposes API methods like the decide methods. Each client corresponds to the datafile representing the state of a project for a certain environment.
You do not need to manage the datafile directly in the Android SDK. The SDK has an additional abstraction called a manager that handles getting the datafile and instantiating the client for you during the SDK's initialization process. The manager includes support for datafile polling to update the datafile on a regular interval automatically.
Version
4.0.0 and higher
Description
The constructor accepts a configuration object to configure Optimizely.
Some parameters are optional because the SDK provides a default implementation, but you may want to override these for your production environments. For example, you may want to override these to configure an error handler and logger to catch issues, an event dispatcher to manage network calls, and a User Profile Service to ensure sticky bucketing.
Parameters
The following are the required and optional parameters (client constructed using the Builder class and passing the following to Build()
).
Parameter | Type | Description |
---|---|---|
sdkKey required | String | The SDK key is used to define the config file for the environment. |
eventProcessor optional | EventProcessor | An event handler object provides an intermediary processing stage for events. The EventProcessor dispatches events through a provided EventHandler. |
eventHandler optional | EventHandler | An event handler dispatching events to the Optimizely event end-point. |
errorHandler optional | ErrorHandler | An error handler object to handle errors. |
logger optional | Logger | A logger implementation to log messages. |
userProfileService optional | UserProfileService | A user profile service. An object with lookup and save methods. |
datafileDownloadInterval optional | Long | Sets the interval (in the specified TimeUnit) at which DatafileHandler attempts to update the cached datafile periodically.If this value is not set, background updates are disabled. The minimum interval is 15 minutes (enforced by the Android JobScheduler API). |
eventFlushInterval optional | Long | Sets the interval (in the specified TimeUnit) in which queued events are flushed periodically. If this value is not set, the default interval (30 seconds) is used. |
defaultDecideOptions optional | Array | An array of OptimizelyDecideOption enums. When the Optimizely client is constructed with this parameter, it sets default decide options applied to all the Decide calls made during the lifetime of the Optimizely client. Additionally, you can pass options to individual Decide methods (does not override defaults).For details on decide options, see OptimizelyDecideOption. |
odpEnabled optional | Boolean | Enable or disable Real-Time Segments for Feature Experimentation integration. |
vuidEnabled optional | Boolean | Enable or disable automatic VUID creation and management. See VUIDs and client-side IDs. |
odpSegmentCacheSize optional | Int | Override the default ODP segment cache size (100). |
odpSegmentCacheTimeoutInSecs optional | Int | Override the default ODP segment cache timeout (10 minutes). |
timeoutForODPSegmentFetchInSecs optional | Int | Override the default timeout of ODP segment fetch (10 seconds). |
timeoutForODPEventDispatchInSecs optional | Int | Override the default timeout of ODP event dispatch (10 seconds). |
odpEventManager optional | ODPEventManager | Provide an optional custom ODPEventManager instance. |
odpSegmentManager optional | ODPSegmentManager | Provide an optional custom ODPSegmentManager instance. |
Customize ODPManager
OdpManager
contains the logic supporting Real-Time Segments for Feature Experimentation integration-related features, including audience segments.
Information
If necessary to disable Real-Time Segments for Feature Experimentation altogether, call
optimizelyManagerBuilder.withODPDisabled()
.
The following settings are optionally configurable when the Android SDK is initialized:
- ODP SegmentsCache size –
.withODPSegmentCacheSize
- Default – 100
- Set to 0 to disable caching.
- ODP SegmentsCache timeout (in seconds) –
.withODPSegmentCacheTimeout
- Default – 600 secs (10 minutes)
- Set to 0 to disable timeout (never expires).
- ODP disable –
.withODPDisabled
- Default – false (enabled)
- When disabled, the Android SDK will disable ODP-related features. The Android SDK still creates and manages VUID regardless of this flag and supports VUID-based decisions. See anonymous users.
- The Android SDK returns or logs an
odpNotEnabled
error when ODP is disabled and its features are requested.
- VUID enable – idEnabled`
Important
.withVuidEnabled
Only available on the Android SDK version 5.0.0+. See SDK compatibility matrix- Default – false (disabled)
- When enabled, the Android SDK creates and manages VUID and supports VUID-based decisions. See anonymous users.
val disableODP = false // Setting this true will disable Real-Time Segments for Feature Experimentation
var enableVuid = false // Set this true to enable VUID
val optimizelyManagerBuilder = OptimizelyManager.builder()
.withSDKKey("SDK_KEY_HERE")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.withEventDispatchInterval(60, TimeUnit.SECONDS)
.withTimeoutForODPEventDispatch(10) // in seconds
.withTimeoutForODPSegmentFetch(10) // in seconds
.withODPSegmentCacheSize(10)
.withODPSegmentCacheTimeout(10, TimeUnit.SECONDS)
if (disableODP) {
optimizelyManagerBuilder.withODPDisabled() // Disables Real-Time Segments for Feature Experimentation
}
if (enableVuid) {
optimizelyManagerBuilder.withVuidEnabled() // Enable VUID
}
if (enableVuid) {
optimizelyManagerBuilder.withVuidEnabled()
}
val optimizelyManager = optimizelyManagerBuilder.build(applicationContext)
// Instantiate a client synchronously with a bundled datafile
val datafile = "REPLACE_WITH_YOUR_DATAFILE"
val optimizelyClient = optimizelyManager.initialize(this, datafile)
// Or, instantiate it asynchronously with a callback
optimizelyManager.initialize(this, null) { client: OptimizelyClient? ->
// flag decision with userID (optional)
val user1 = optimizelyClient.createUserContext("USER_ID_HERE")
val decision = user1!!.decide("FLAG_KEY_HERE")
// flag decision with auto-generated VUID
val user2 = optimizelyClient.createUserContext()
val decision2 = user2!!.decide("FLAG_KEY_HERE")
}
boolean disableODP = false; // Setting this true will disable the odp
OptimizelyManager.Builder optimizelyManagerBuilder = OptimizelyManager.builder()
.withSDKKey("SDK_KEY_HERE")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.withEventDispatchInterval(60, TimeUnit.SECONDS)
.withTimeoutForODPEventDispatch(10) // in seconds
.withTimeoutForODPSegmentFetch(10) // in seconds
.withODPSegmentCacheSize(10)
.withODPSegmentCacheTimeout(10, TimeUnit.SECONDS);
if (disableODP) {
optimizelyManagerBuilder.withODPDisabled(); // This function will disable the odp
}
OptimizelyManager optimizelyManager =
optimizelyManagerBuilder.build(getApplicationContext());
// Instantiate a client synchronously with a bundled datafile
String datafile = "REPLACE_WITH_YOUR_DATAFILE";
OptimizelyClient optimizelyClient = optimizelyManager.initialize(this, datafile);
// Or, instantiate it asynchronously with a callback
optimizelyManager.initialize(context, null, (OptimizelyClient client) -> {
// flag decision with userID (optional)
OptimizelyUserContext user1 = optimizelyClient.createUserContext("USER_ID_HERE");
OptimizelyDecision decision = user1.decide("FLAG_KEY_HERE");
// flag decision with auto generated vuid
OptimizelyUserContext user2 = optimizelyClient.createUserContext();
OptimizelyDecision decision = user2.decide("FLAG_KEY_HERE");
});
Example
The manager is implemented as a factory for Optimizely client instances. To use it, create a manager object by supplying your SDK key and optional configuration settings for datafile polling and datafile bundling. The SDK key is in Settings > Environments.
Next, choose whether to instantiate the client synchronously or asynchronously and use the appropriate initialize
method to instantiate a client.
If you use a bundled datafile, copy the datafile JSON string from the Optimizely application. Typically, you want to copy the datafile string at the latest point possible before release.
// Build a manager
val optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.withEventDispatchInterval(30, TimeUnit.SECONDS)
.build(context)
// Instantiate a client synchronously with a bundled datafile
val datafile = "REPLACE_WITH_YOUR_DATAFILE"
val optimizelyClient = optimizelyManager.initialize(context, datafile)
// Or, instantiate it asynchronously with a callback
optimizelyManager.initialize(context, null) { client: OptimizelyClient ->
// flag decision
val user = client.createUserContext("<User_ID>")!!
val decision = user.decide("<Flag_Key>")
}
// Build a manager
OptimizelyManager optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.withEventDispatchInterval(30, TimeUnit.SECONDS)
.build(context);
// Instantiate a client synchronously with a bundled datafile
String datafile = "REPLACE_WITH_YOUR_DATAFILE";
OptimizelyClient optimizelyClient = optimizelyManager.initialize(context, datafile);
// Or, instantiate it asynchronously with a callback
optimizelyManager.initialize(context, null, (OptimizelyClient client) -> {
// flag decision
OptimizelyUserContext user = client.createUserContext("<User_ID>");
OptimizelyDecision decision = user.decide("<Flag_Key>");
});
See the Android example: _MainActivity.java
.
Get a client
Each manager retains a reference to its most recently initialized client. You can use the getOptimizely
function to return that instance.
A dummy client instance is created and returned if this method is called before any client objects are initialized. This dummy instance logs errors when any of its methods are used.
val optimizelyClient = optimizelyManager.optimizely
OptimizelyClient optimizelyClient = optimizelyManager.getOptimizely();
Use synchronous or asynchronous initialization
You can instantiate the Optimizely client synchronously or asynchronously.
- The behavioral difference between the two methods is whether your application pings the Optimizely Experimentation servers for a new datafile during initialization.
- The functional difference between the two methods is whether your app prioritizes accuracy or speed.
Important
You must decide to initialize the Android SDK either synchronously or asynchronously. You cannot use both initialization methods.
Synchronous initialization
The synchronous method prioritizes speed over accuracy.
Instead of attempting to download a new datafile every time you initialize an Optimizely client, your app uses a local version of the datafile. This local datafile can be cached from a previous network request (see configure datafile polling) or embedded within your app (see enable bundled datafiles).
When you initialize an Optimizely client synchronously, the Optimizely manager first searches for a cached datafile. If one is available, the manager uses it to complete the client initialization. If the manager cannot find a cached datafile, the manager searches for a bundled datafile. If the manager finds a bundled datafile, it uses the datafile to complete the client initialization. If the manager cannot find a bundled datafile, the manager cannot initialize the client.
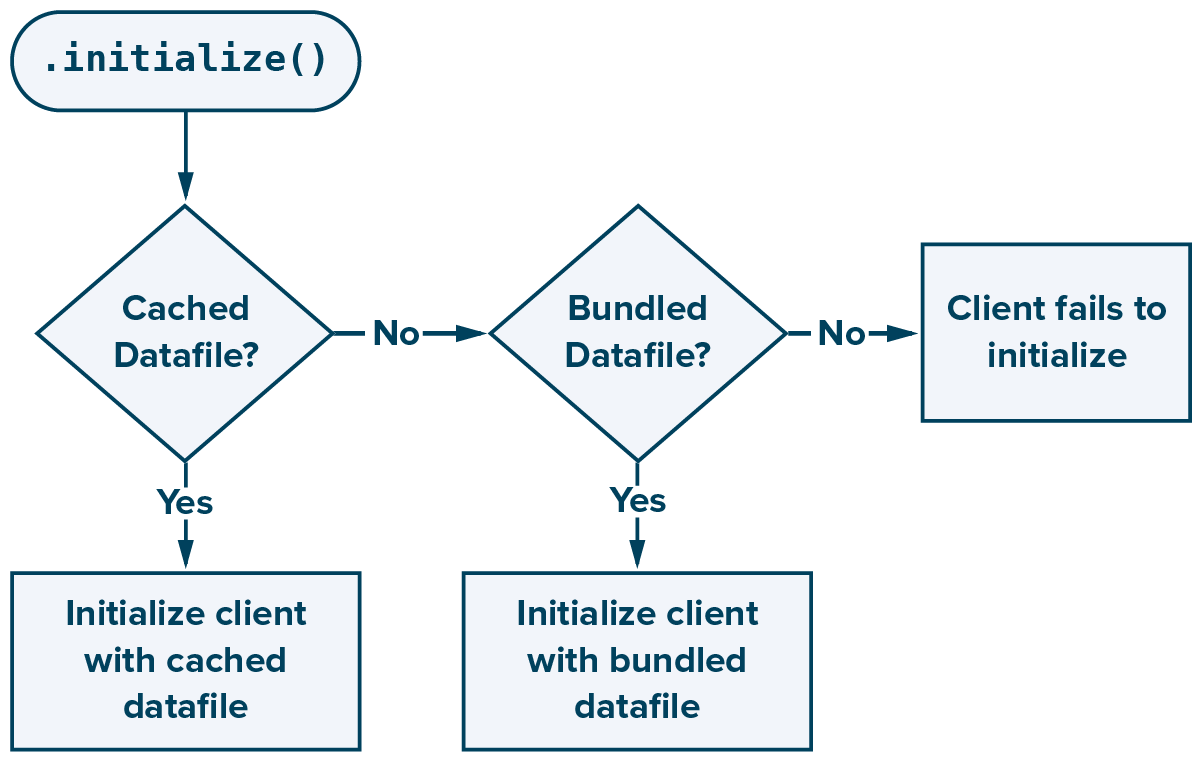
To initialize an OptimizelyClient
object synchronously, call OptimizelyManager#initialize()
and provide two arguments:
- The Application instance (from
android.app.Application
). - An
Integer
pointer to the application resource datafile.
For more details on the specific requirements, view OptimizelyManager.java
from the publicly available Android SDK.
Asynchronous initialization
The asynchronous method prioritizes accuracy over speed.
During initialization, your app requests the newest datafile from the CDN servers. Requesting the newest datafile ensures that your app always uses the most current project settings, but it also means your app cannot instantiate a client until it downloads a new datafile, discovers the datafile has not changed, or until the request times out. This process takes time.
Initializing a client asynchronously executes like the synchronous initialization, except the manager will first attempt to download the newest datafile. This network activity is what causes an asynchronous initialization to take longer to complete.
If the network request returns an error (such as when network connectivity is unreliable) or if the manager discovers that the cached datafile is identical to the newest datafile, the manager then uses the synchronous approach. If the manager discovers that the datafile was updated and now differs from the cached datafile, the manager downloads the new datafile and uses it to initialize the client.
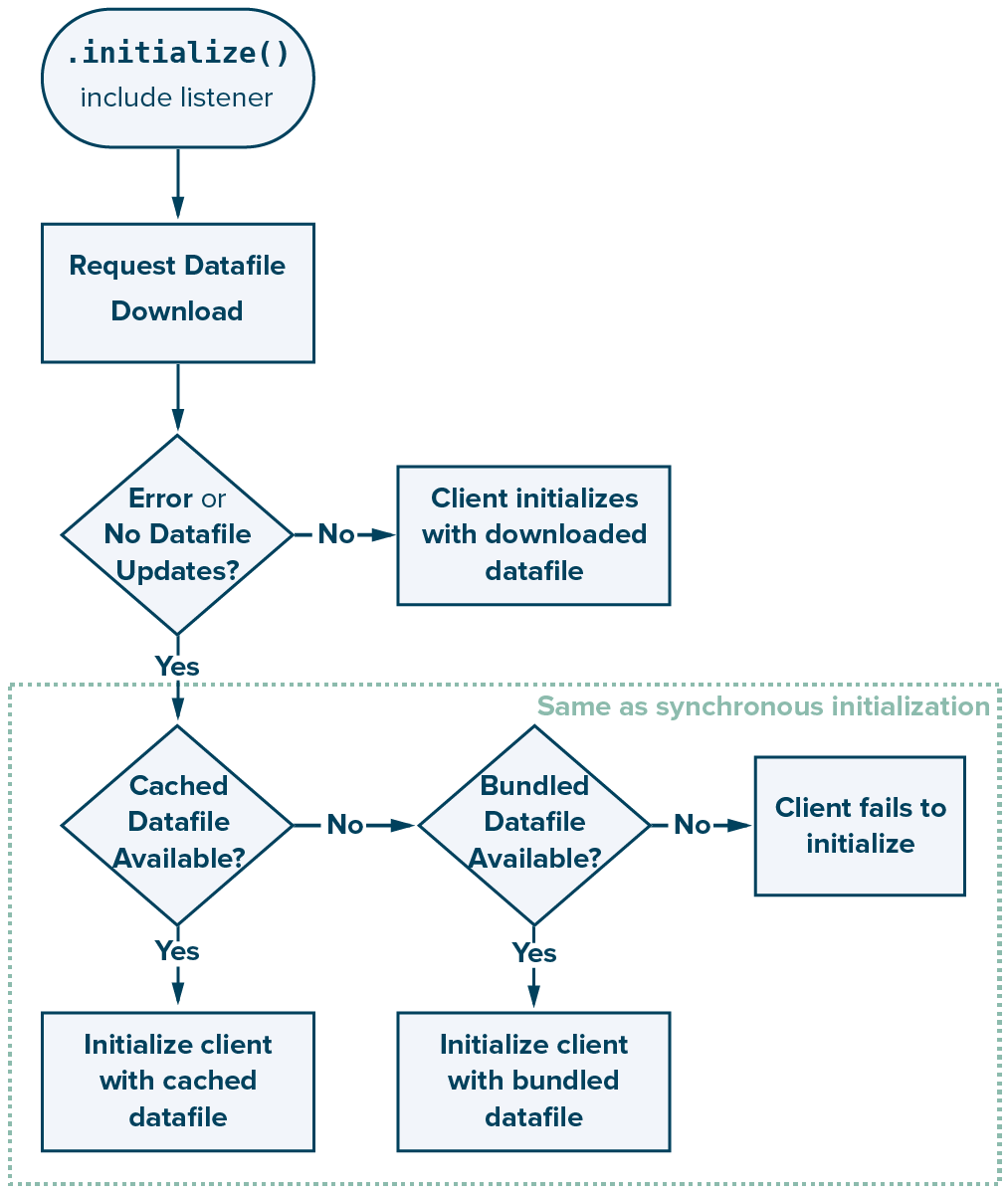
To initialize an OptimizelyClient
object asynchronously, call OptimizelyManager#initialize()
and three arguments:
- The Application instance (from
android.app.Application
). - An
Integer
pointer to the application resource datafile. - A listener object.
For more details on the specific requirements and to see how to build each object, see OptimizelyManager.java
from the publicly available Android SDK.
Configure datafile polling
The Optimizely manager attempts to pull the newest datafile from the CDN servers during its initialization. After this, the Optimizely manager can periodically check for and pull a new datafile at the time interval you set during its initialization. The process of checking for and downloading a new datafile is called datafile polling.
Datafile polling is disabled by default, so the manager only checks for a new datafile during initialization. To enable polling, set it to a non-zero interval value. This value is the number of seconds the manager waits between datafile polling attempts.
// Poll every 15 minutes
val optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.build(context)
// Poll every 15 minutes
OptimizelyManager optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.build(context);
Usage notes
- The minimum polling interval is 900 seconds (which is 15 minutes, enforced by the Android JobScheduler API).
- Updated datafiles are automatically picked up because the default datafile handler now supports the ProjectConfigManager API.
- You can register for datafile change notifications as well with the UpdateConfigNotification on Github:
optimizelyClient.addUpdateConfigNotificationHandler { notification -> println("got datafile change") }
optimizelyClient.addUpdateConfigNotificationHandler(notification -> { System.out.println("got datafile change"); });
To override the default datafile handler and use your own, see DatafileHandler on GitHub.
Enable bundled datafiles
When your customer opens your app for the first time after installing or updating it, the manager attempts to pull the newest datafile from Optimizely's CDN. If your app cannot contact the servers, the manager can substitute a datafile that you included (bundled) when you created your app.
By bundling a datafile within your app, you ensure that the Optimizely manager can initialize without waiting for a response from the CDN. This prevents poor network connectivity from causing your app to hang or crash while it loads.
Datafile bundling works with synchronous and asynchronous initialization because reverting to a bundled datafile happens during the Optimizely manager's initialization before a client is instantiated.
// Initialize Optimizely asynchronously with a datafile.
// If it is not able to download a new datafile, it
// initializes an OptimizelyClient with the one provided.
optimizelyManager.initialize(context, R.raw.datafile) { optimizelyClient: OptimizelyClient ->
val user = optimizelyClient.createUserContext("<User_ID>")!!
val decision = user.decide("<Flag_Key>")
}
// Initialize Optimizely synchronously
// This immediately instantiates and returns an
// OptimizelyClient with the datafile that was passed in.
// It also downloads a new datafile from the Optimizely CDN and
// persist it to local storage.
// The newly downloaded datafile is used the next
// time the SDK is initialized.
optimizelyManager.initialize(context, R.raw.datafile)
// Initialize Optimizely asynchronously with a datafile.
// If it is not able to download a new datafile, it will
// initialize an OptimizelyClient with the one provided.
optimizelyManager.initialize(context, R.raw.datafile, (OptimizelyClient optimizelyClient) -> {
OptimizelyUserContext user = optimizelyClient.createUserContext("<User_ID>");
OptimizelyDecision decision = user.decide("<Flag_Key>");
});
// Initialize Optimizely synchronously
// This will immediately instantiate and return an
// OptimizelyClient with the datafile that was passed in.
// It'll also download a new datafile from the CDN and
// persist it to local storage.
// The newly downloaded datafile will be used the next
// time the SDK is initialized.
optimizelyManager.initialize(context, R.raw.datafile);
Dispose of the client
For effective resource management with the Optimizely Android SDK, you must properly close the Optimizely client instance when it is no longer needed. This is done by callingoptimizelyClient.close()
.
The .close()
method ensures that the processes and queues associated with the instance are properly released. This is essential for preventing memory leaks and ensuring that the application runs efficiently, especially in environments where resources are limited or in applications that create and dispose of many instances over their lifecycle.
See Close Optimizely Feature Experimentation Android SDK on application exit.
More sample code
// Here are more sample codes for synchronous and asynchronous SDK initializations with multiple options
// [Synchronous]
// [S1] Synchronous initialization
// 1. SDK is initialized instantly with a cached (or bundled) datafile
// 2. A new datafile can be downloaded in background and cached after the SDK is initialized.
// The cached datafile will be used only when the SDK re-starts in the next session.
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.build(context)
optimizelyClient = optimizelyManager.initialize(context, R.raw.datafile)
user = optimizelyClient.createUserContext("<User_ID>")!!
decision = user.decide("<Flag_Key>")
// [S2] Synchronous initialization
// 1. SDK is initialized instantly with a cached (or bundled) datafile
// 2. A new datafile can be downloaded in background and cached after the SDK is initialized.
// The cached datafile is used immediately to update the SDK project config.
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.build(context)
optimizelyClient = optimizelyManager.initialize(context, R.raw.datafile, true, true)
user = optimizelyClient.createUserContext("<User_ID>")!!
decision = user.decide("<Flag_Key>")
// [S3] Synchronous initialization
// 1. SDK is initialized instantly with a cached (or bundled) datafile
// 2. A new datafile can be downloaded in background and cached after the SDK is initialized.
// The cached datafile is used immediately to update the SDK project config.
// 3. Polling datafile periodically.
// The cached datafile is used immediately to update the SDK project config.
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.build(context)
optimizelyClient = optimizelyManager.initialize(context, R.raw.datafile)
user = optimizelyClient.createUserContext("<User_ID>")!!
decision = user.decide("<Flag_Key>")
// [Asynchronous]
// [A1] Asynchronous initialization
// 1. A datafile is downloaded from the server and the SDK is initialized with the datafile
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.build(context)
optimizelyManager.initialize(context, null) { client: OptimizelyClient ->
val user = client.createUserContext("<User_ID>")!!
val decision = user.decide("<Flag_Key>")
}
// [A2] Asynchronous initialization
// 1. A datafile is downloaded from the server and the SDK is initialized with the datafile
// 2. Polling datafile periodically.
// The cached datafile is used immediately to update the SDK project config.
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.build(context)
optimizelyManager.initialize(context, null) { client: OptimizelyClient ->
val user = client.createUserContext("<User_ID>")!!
val decision = user.decide("<Flag_Key>")
}
// Here are more sample codes for synchronous and asynchronous SDK initializations with multiple options
// [Synchronous]
// [S1] Synchronous initialization
// 1. SDK is initialized instantly with a cached (or bundled) datafile
// 2. A new datafile can be downloaded in background and cached after the SDK is initialized.
// The cached datafile will be used only when the SDK re-starts in the next session.
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.build(context);
optimizelyClient = optimizelyManager.initialize(context, R.raw.datafile);
user = optimizelyClient.createUserContext("<User_ID>");
decision = user.decide("<Flag_Key>");
// [S2] Synchronous initialization
// 1. SDK is initialized instantly with a cached (or bundled) datafile
// 2. A new datafile can be downloaded in background and cached after the SDK is initialized.
// The cached datafile is used immediately to update the SDK project config.
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.build(context);
optimizelyClient = optimizelyManager.initialize(context, R.raw.datafile, true, true);
user = optimizelyClient.createUserContext("<User_ID>");
decision = user.decide("<Flag_Key>");
// [S3] Synchronous initialization
// 1. SDK is initialized instantly with a cached (or bundled) datafile
// 2. A new datafile can be downloaded in background and cached after the SDK is initialized.
// The cached datafile is used immediately to update the SDK project config.
// 3. Polling datafile periodically.
// The cached datafile is used immediately to update the SDK project config.
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.build(context);
optimizelyClient = optimizelyManager.initialize(context, R.raw.datafile, true, true);
user = optimizelyClient.createUserContext("<User_ID>");
decision = user.decide("<Flag_Key>");
// [Asynchronous]
// [A1] Asynchronous initialization
// 1. A datafile is downloaded from the server and the SDK is initialized with the datafile
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.build(context);
optimizelyManager.initialize(context, null, (OptimizelyClient client) -> {
OptimizelyUserContext userContext = client.createUserContext("<User_ID>");
OptimizelyDecision optDecision = userContext.decide("<Flag_Key>");
});
// [A2] Asynchronous initialization
// 1. A datafile is downloaded from the server and the SDK is initialized with the datafile
// 2. Polling datafile periodically.
// The cached datafile is used immediately to update the SDK project config.
optimizelyManager = OptimizelyManager.builder()
.withSDKKey("<Your_SDK_Key>")
.withDatafileDownloadInterval(15, TimeUnit.MINUTES)
.build(context);
optimizelyManager.initialize(context, null, (OptimizelyClient client) -> {
OptimizelyUserContext userContext = client.createUserContext("<User_ID>");
OptimizelyDecision optDecision = userContext.decide("<Flag_Key>");
});
Source files
The language and platform source files containing the implementation for Android are available on GitHub.
Updated 3 months ago