Real-Time Segments for Feature Experimentation segment qualification methods for the React Native SDK
Use the fetchQualifiedSegments
method to retrieve the external audience mapping for the user from the Optimizely Data Platform (ODP) server. Use the isQualifiedFor
method to check if the user qualifies for the specified segment.
Prerequisites
You must enable and configure Real-Time Segments for Feature Experimentation before fetching qualified segments and checking if the user is qualified for the given audience segment.
fetchQualifiedSegments
Minimum SDK Version
v3.0.0 or higher
Description
You can use the fetchQualifiedSegments
method to retrieve the external audience mapping for a specific user from the ODP server. The Optimizely Feature Experimentation React Native SDK provides an asynchronous version of the Optimizely Data Platform (ODP) fetchQualifiedSegments
method.
fetchQualfiedSegments
is a method of the ReactSDKClient
object.
Parameters
The following table describes the parameters for the fetchQualifiedSegments
method:
Parameter | Type | Description |
---|---|---|
options (optional) | String | A set of options for fetching qualified segments from ODP. |
Returns
This method returns a Promise, which will resolve with true if segments are fetched successfully, and false otherwise.
If the React Native SDK does not find an ODP audience in the datafile, it returns an empty qualified segments array without sending a request to the ODP server.
Note
You can read and write directly to the qualified segments array. This lets you bypass the remote fetching process from ODP or utilize your own fetching service. This can be helpful when testing or debugging.
Example fetchQualifiedSegments
call
fetchQualifiedSegments
callThe following is an example of calling the fetchQualifiedSegments method and accessing the returned completion object
import { createInstance } from "@optimizely/react-sdk";
const optimizelyClient = createInstance({
sdkKey: '<Your_SDK_Key>'
});
export default function App() {
optimizelyClient.onReady().then(() => {
optimizelyClient.fetchQualifiedSegments();
});
return (
<YourComponent />
);
}
The following diagram shows the network calls between your application, the React Native SDK, and the ODP server when calling fetchQualifiedSegments
:
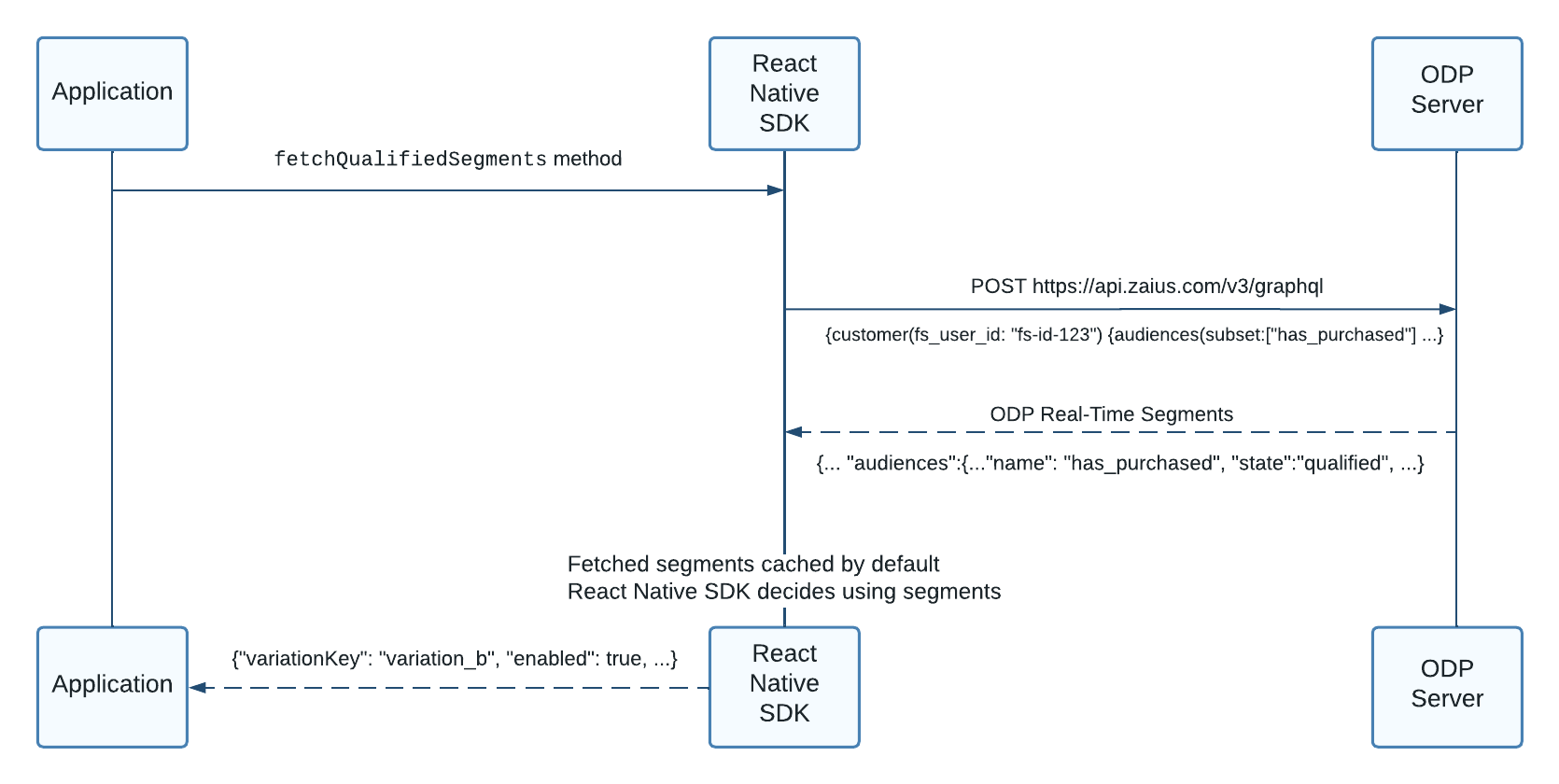
- Call the fetchQualifiedSegments` method.
- The React Native SDK makes a GraphQL call to ODP to fetch segments.
- ODP responds with segments.
- Fetched segments mapping user IDs to segments are cached.
- Appropriate variations are returned for the user.
After the segments are fetched, they are cached. This means that if the same user is requesting the segments again (when new user contexts are created), the audience segment information can be retrieved from the cache instead of being fetched again from the remote ODP server.
The cache is used for the fetchQualifiedSegments
call. This method is called on the user context (the user context is fixed, including the real-time segments the user qualifies for).
The cache only applies when calling the fetchQualifiedSegments
call. If you set the cache timeout to 0, the cache is disabled. Optimizely uses the LRU algorithm, so the oldest record is bumped out when the maximum size is reached. If there is a cache miss upon the method call, Optimizely makes a network request.
If you would like to bypass caching, you can add the following options to your options
array:
OptimizelySegmentOption.IGNORE_CACHE
– Bypass segments cache for lookup and save.OptimizelySegmentOption.RESET_CACHE
– Reset all segments cache.
isQualifiedFor
Minimum SDK version
3.0.0 or higher
Description
Check if the user is qualified for the given audience segment.
Parameters
The following table describes the parameters for the isQualifiedFor
method:
Parameter | Type | Description |
---|---|---|
segment | string | The ODP audience segment name to check if the user is qualified for. |
Returns
true
if the user is qualified otherwise, false
Examples
The following is an example of whether or not the user is qualified for an ODP segment:
import { createInstance } from "@optimizely/react-sdk";
const optimizelyClient = createInstance({
sdkKey: '<Your_SDK_Key>'
});
export default function App() {
optimizelyClient.onReady().then(() => {
optimizelyClient.fetchQualifiedSegments();
const isQualifiedForSegment1 = optimizelyClient.getUserContext()?.isQualifiedFor("segment1");
console.log(isQualifiedForSegment1); // true
});
return (
<YourComponent />
);
}
Source files
The language and platform source files containing the implementation for React Native are available on Github.
Updated 3 months ago