Remote select options
Describes how to populate a select list with available options using a function in your Optimizely Connect Platform (OCP) app.
You can populate select
and multi-select
form elements with a hard-coded list of values or an external data source. For example, to allow the user to select one or more Mailchimp lists that the app retrieves from Mailchimp using the customer's API credentials, specify a dataSource
on the select
element:
sections:
- key: config
label: Configuration
elements:
- type: select
key: list_id
label: List Name
help: Name of your list in Mailchimp with contacts to sync OCP Insights to. If this does not populate, your API key is likely not entered correctly.
dataSource:
type: app
function: get_lists
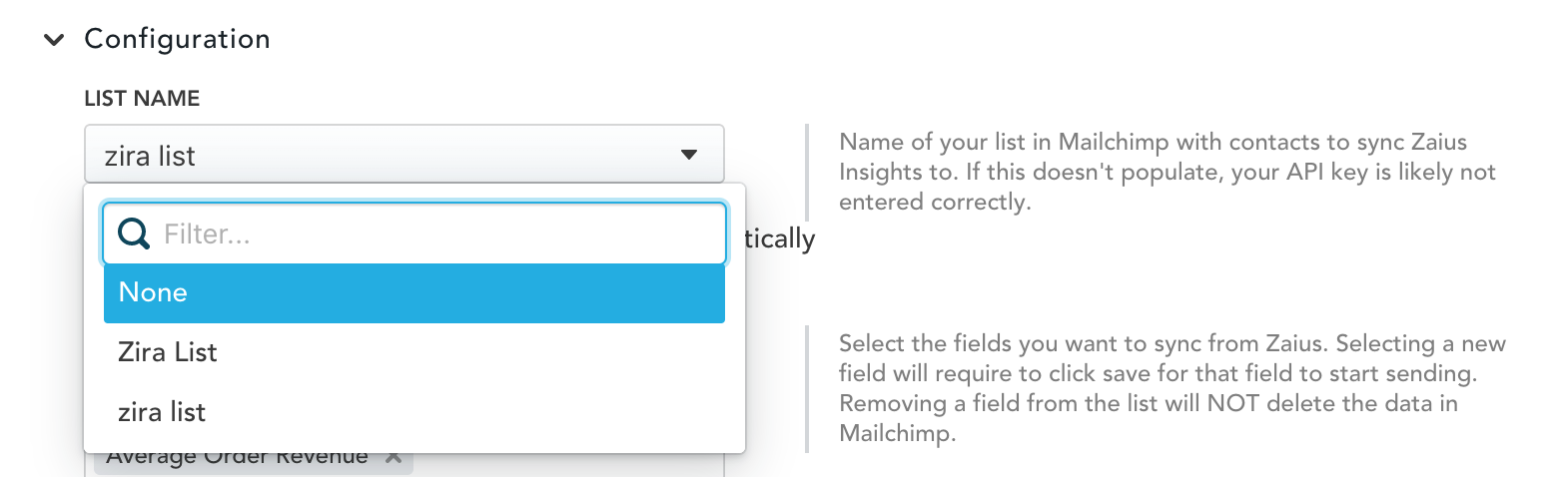
Specify app
as the dataSource
app
as the dataSource
By specifying app
as the dataSource
type, you can have the form call a function
defined in your app to get the list of options available. In the example above, the Mailchimp app calls the get_lists
function any time you need to populate or show the list to the user. The app now has access to all the other form data, including the user's Mailchimp API key. The function is responsible for returning a JSON list of options in the following format:
[
{
"text": "Text to show the user",
"value": "internal value"
},
...
]
Below is an example of how you can implement this:
import {Schema} from '@zaiusinc/app-forms-schema';
import * as App from '@zaiusinc/app-sdk';
import {logger} from '@zaiusinc/app-sdk';
import {MailChimp} from '../lib/MailChimp';
export class GetLists extends App.Function {
public async perform(): Promise<App.Response> {
logger.info(this.request.body);
const mc = new MailChimp();
const lists = await mc.getLists();
const options: Schema.SelectOption[] = [{text: 'None', value: null}];
lists.forEach((l) => {
options.push({text: l.name, value: l.id});
});
return new App.Response(200, options);
}
}
Specify http
as the dataSource
http
as the dataSource
If your select options are available publicly over HTTP, you can alternatively use an HTTP data source by specifying http
as the dataSource
type in the form. For example:
...
dataSource:
type: http
function: https://example-site.io/data/options.json
The options must still be in the same JSON format that app functions are expected to return. If they are not, you can use app
as the dataSource
to fetch the options from your app's function and format them correctly instead of going directly to the source.
Updated about 1 year ago