Run a beta
This guide walks through how to run a beta and assumes you have a feature already created.
Feature flags enable you to run a beta, so you can show a feature to a specific subset of users before releasing it to your general audience instead of rolling your feature out to a random percentage of your user-base.
1. Decide on an attribute
An attribute is a property in your data that can be used to determine whether to target a feature to a given customer or user. Decide which attribute to use for your beta based on the data you have available in your application:
-
If you have a consumer application with multiple users, and you'd like to run your beta user by user, consider making the unique identifier that is associated with an individual user in your application an attribute in Optimizely (ex:
userId
). -
If you have a business application that has multiple customers or accounts and want to run a beta customer by customer, consider making the unique identifier that is associated with an individual customer in your application an attribute in Optimizely (ex:
customerId
,accountId
,projectId
, etc.).
2. Implement the attribute
The next step is to create an attribute in Optimizely for the attribute selected above. For example, if you were running a beta by enabling features for a particular customerId
, create an attribute in Optimizely with the key customerId
.
In your code, pass the value from the data available in your application to the Is Feature Enabled
API as an attribute. The below example shows how you would do this if you were running a beta based on customerId
:
// Implement the customerId attribute
int customerId = 123;
Map<String, Object> attributes = new HashMap<>();
attributes.put("customerId", customerId);
boolean enabled = optimizelyClient.isFeatureEnabled('new_feature', 'user123', attributes);
// Implement the customerId attribute
int customerId = 123;
UserAttributes attributes = new UserAttributes
{
{ "customerId", customerId },
};
bool enabled = OptimizelyClient.IsFeatureEnabled("new_feature", "user123", attributes);
// Implement the customerId attribute
int customerId = 123;
Map<String, Object> attributes = new HashMap<>();
attributes.put("customerId", customerId);
boolean enabled = optimizelyClient.isFeatureEnabled('new_feature', 'user123', attributes);
<OptimizelyProvider
optimizely={optimizely}
user={{
id: 'user123',
attributes: {
customerId: 123,
}
}}
>
</OptimizelyProvider>
// Implement the customerId attribute
const customerId = 123;
const attributes = {
customerId: customerId,
};
const enabled = optimizelyClientInstance.isFeatureEnabled('new_feature', 'user123', attributes);
// Implement the customerId attribute
const customerId = 123;
const attributes = {
customerId: customerId,
};
const enabled = optimizelyClientInstance.isFeatureEnabled('new_feature', 'user123', attributes);
// Implement the customerId attribute
int customerId = 123;
NSDictionary *attributes = @{
@"customerId": customerId
};
bool enabled = [client isFeatureEnabledWithFeatureKey:@"new_feature" userId:@"user123" attributes: attributes];
// Implement the customerId attribute
$customerId = 123;
$attributes = [
'customerId' => $customerId,
];
$enabled = $optimizelyClient->isFeatureEnabled('new_feature', 'user123', $attributes);
# Implement the customerId attribute
customer_id = 123;
attributes = {
'customerId': customer_id,
}
enabled = optimizely_client.is_feature_enabled('new_feature', 'user123', attributes)
# Change forceEnabled so the value is controlled by the test cookie
customerId = true
attributes = {
'customerId' => customerId,
}
enabled = optimizely_client.is_feature_enabled('new_feature', 'user123', attributes)
// Change forceEnabled so the value is controlled by the test cookie
let customerId = 123
let attributes = [
"customerId": customerId,
]
let enabled = optimizely.isFeatureEnabled(featureKey: "new_feature", userId: "user123", attributes: attributes)
Important
The same attribute may appear twice in the API call
isFeatureEnabled( 'new_feature', 'userId123', // UserId used for random percentage rollout { userId: 'userId123', // Attribute used for non-random audience rollout isVIP: true, }, )
3. Create a beta audience
Once you have implemented the attribute you will use for the beta, create an audience for the beta users of your feature.
-
Name: Include the feature key in the audience name, so you can easily determine which feature this audience is for. For example, if your feature key was
chat_window
, we recommend naming the audience "[chat_window] Beta Users" -
Audience conditions: Drag and drop the attribute that determines who gets access to the beta. For the value of the condition, use an internal or test identifier to include yourself into the beta first to make sure everything is correctly setup.
Important
Be sure to match the value with the attribute's appropriate type.
- If your attribute is an integer, be sure to select "Number equals".
- If your attribute is a string, be sure to select "String equals".
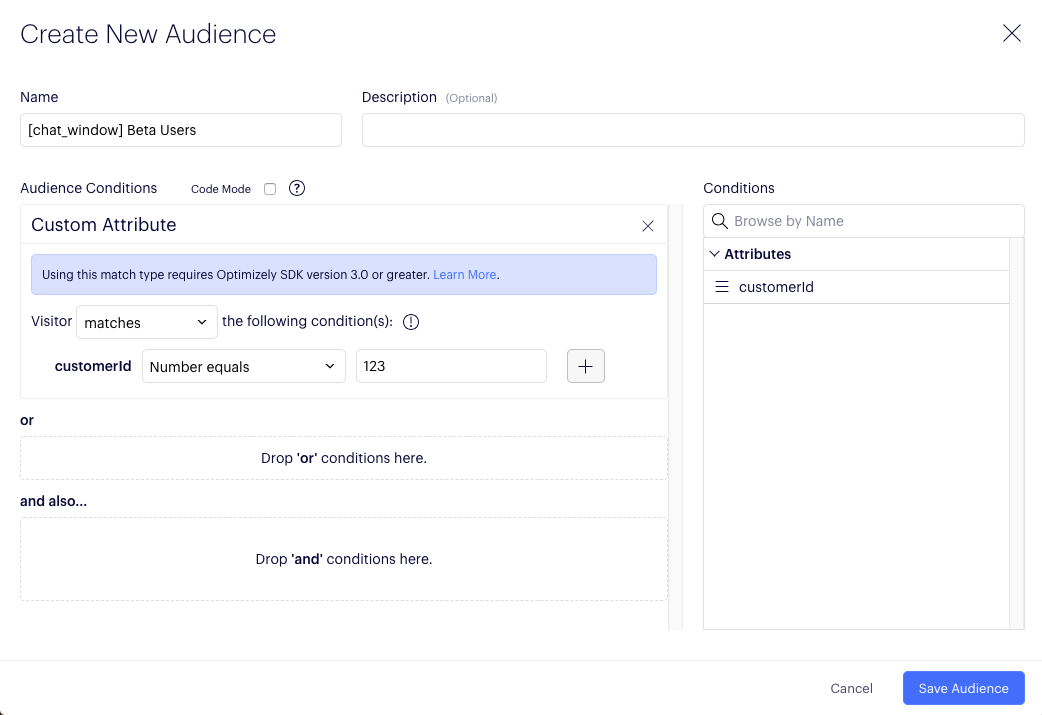
Add the audience you created above as a targeting condition to your feature and save, ensuring that the rollout is configured to 100%:
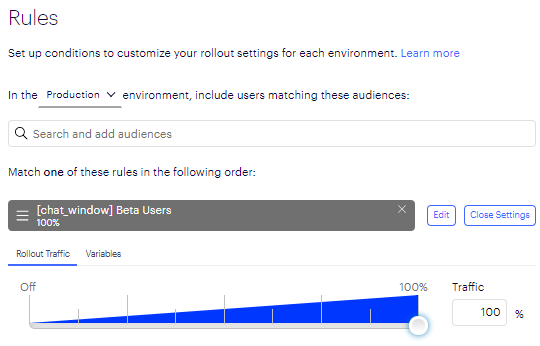
4. Add users to the beta
Now that you have added the audience to your feature, your beta is running. Edit the audience definition to add or remove users from the beta:
- Click on the "+" sign and save to add beta users
- Click on the "x" sign and save to remove beta users
In this example, we've added three customers to the beta. Customers with ids: 123, 456, and 789.
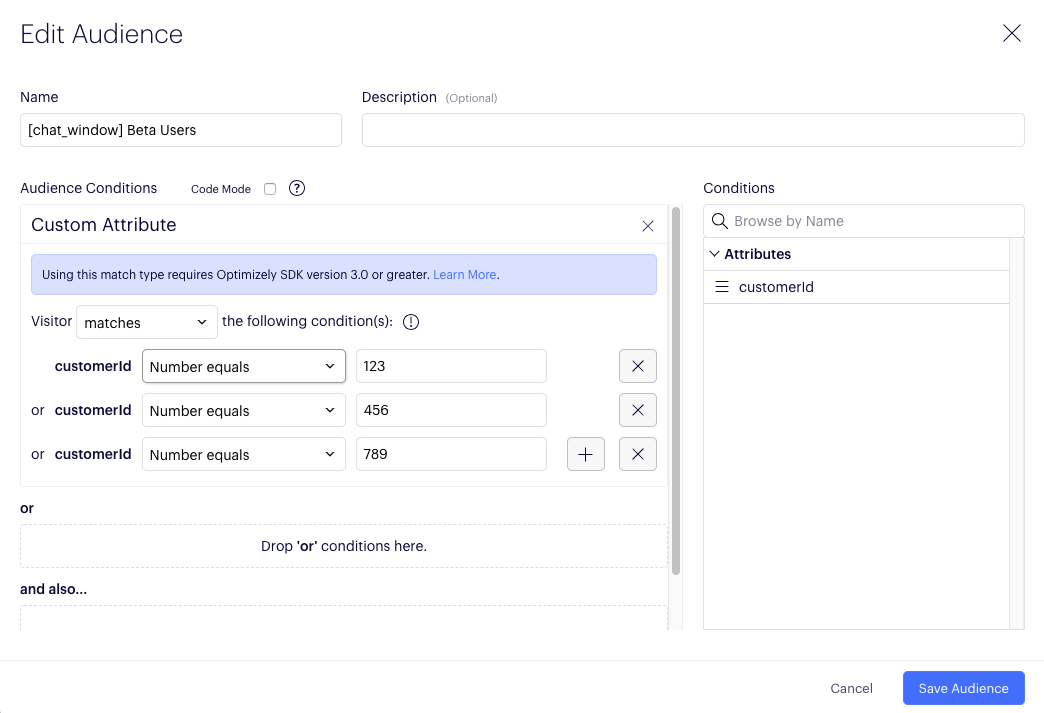
5. Add further audiences
After your first beta audience, you can retain them at 100% and strategically roll out to a sequence of audiences. For example, you could define audiences to roll out to such as:
- other beta audiences
- lowest value customers
- difficult migration customers
- all customers signing up in the last 20 days
- highest value customers
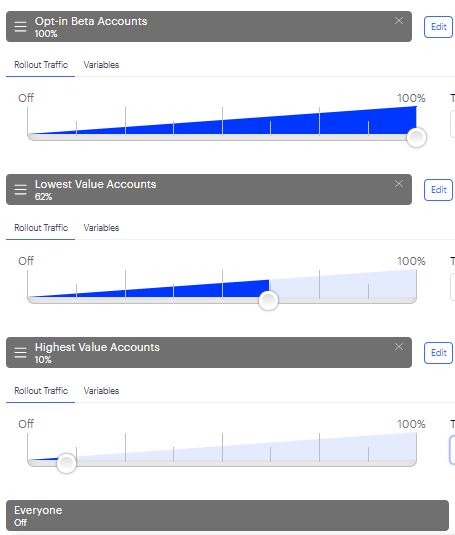
Launch
When all your audiences are at 100% traffic, you are launched! Congratulations!
Updated about 1 year ago
To optimize your feature flag during or after a rollout, test variations of your flag.