C#
Quickstart guide for Optimizely Full Stack
Welcome to the quickstart guide for Optimizely's Full Stack C# SDK. Follow the steps in the guide below to roll out a feature and start your first experiment. You will need an account to follow this guide, if you do not have one yet, you can register for a free account at Optimizely.com.
Steps:
- Install the SDK
- Instantiate Optimizely in your app
- Create a feature flag (with variable) in Optimizely
- Implement the feature in your app
- Create an event to track in Optimizely
- Implement event tracking in your app
- Launch the experiment for your feature!
1. Install the SDK
The C# SDK is distributed through NuGet.
For Windows, to install, run the command Install-Package Optimizely.SDK in the Package Manager Console:
Install-Package Optimizely.SDK
or with .net cli:
dotnet add package Optimizely.SDK
The package is on NuGet. The full source code is available on GitHub.
2. Instantiate Optimizely
For most customer uses we recommend implementing the OptimizelySDK as a singleton. This means that you will be using a single instance of the SDK and datafile. You have control on how often to query for datafile updates.
Next import OptimizelySDK package that installed earlier and create Optimizely instance using OptimizelyFactory.
using OptimizelySDK;
/// inside method body
// datafile will be downloaded async mode
// for sync mode, define ConfigManager explicitly, see the example below
var optimizelyInstance = OptimizelyFactory.NewDefaultInstance("<Your_SDK_Key>");
Or ConfigManager can be defined explicitly.
using OptimizelySDK;
using OptimizelySDK.Config;
/// inside method body
var configManager = new HttpProjectConfigManager
.Builder()
.WithSdkKey("<SDK_KEY>")
.Build(false); // sync mode
var optimizelyInstance = OptimizelyFactory.NewDefaultInstance(configManager);
Remember to replace <Your_SDK_Key> with your own.
To find your SDK Key in your Optimizely project:
- Navigate to Settings > Primary Environment.
- Copy the SDK Key.
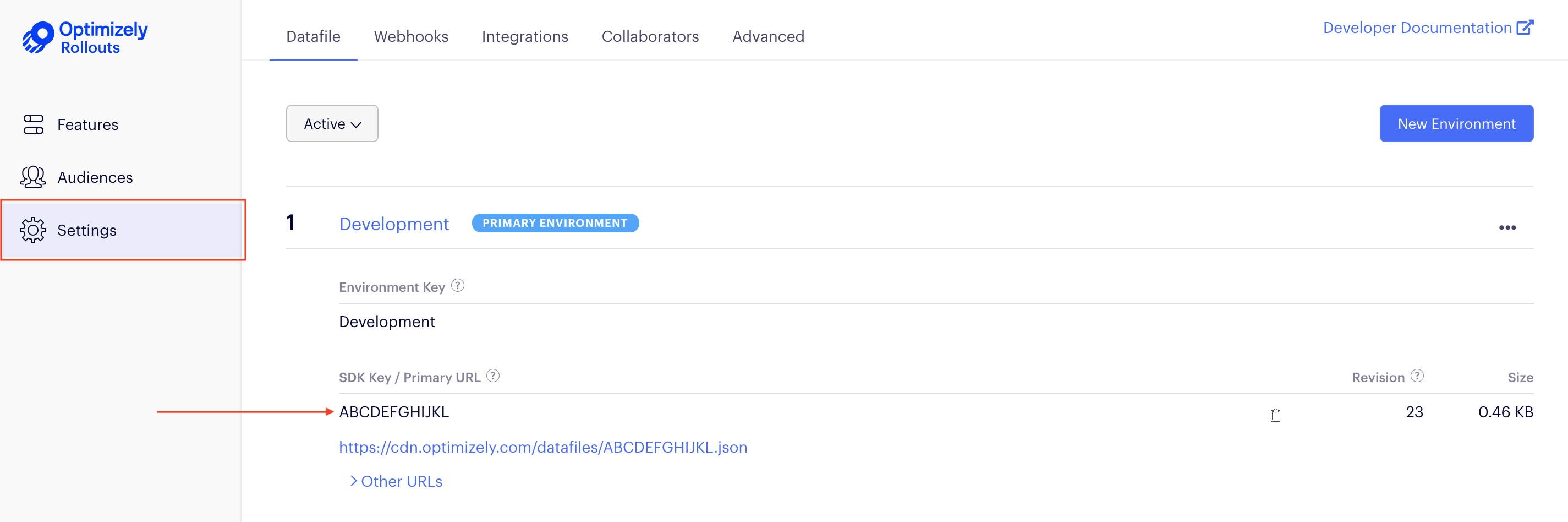
Click image to enlarge
Note
Notice that each environment has its own SDK key.
3. Create a feature flag
A feature flag lets you control which users are exposed to a new feature in your app. For this quickstart, imagine that you run an online store and you’re rolling out a new ‘discount’ feature that shows different discounts to try to increase sales. Create a feature in Optimizely named ‘discount’ and give it a variable named ‘amount’.
Create a feature flag in your Optimizely project:
- Navigate to Features > New Feature.
- Name the feature key discount
- Click Add Variable
- Name the variable amount
- Set the variable type to "Integer"
- Set the variable default value to 5 (this represents $5)
- Skip the rest of the dialog and click Create Feature.
Here is a visual for how to set it up:
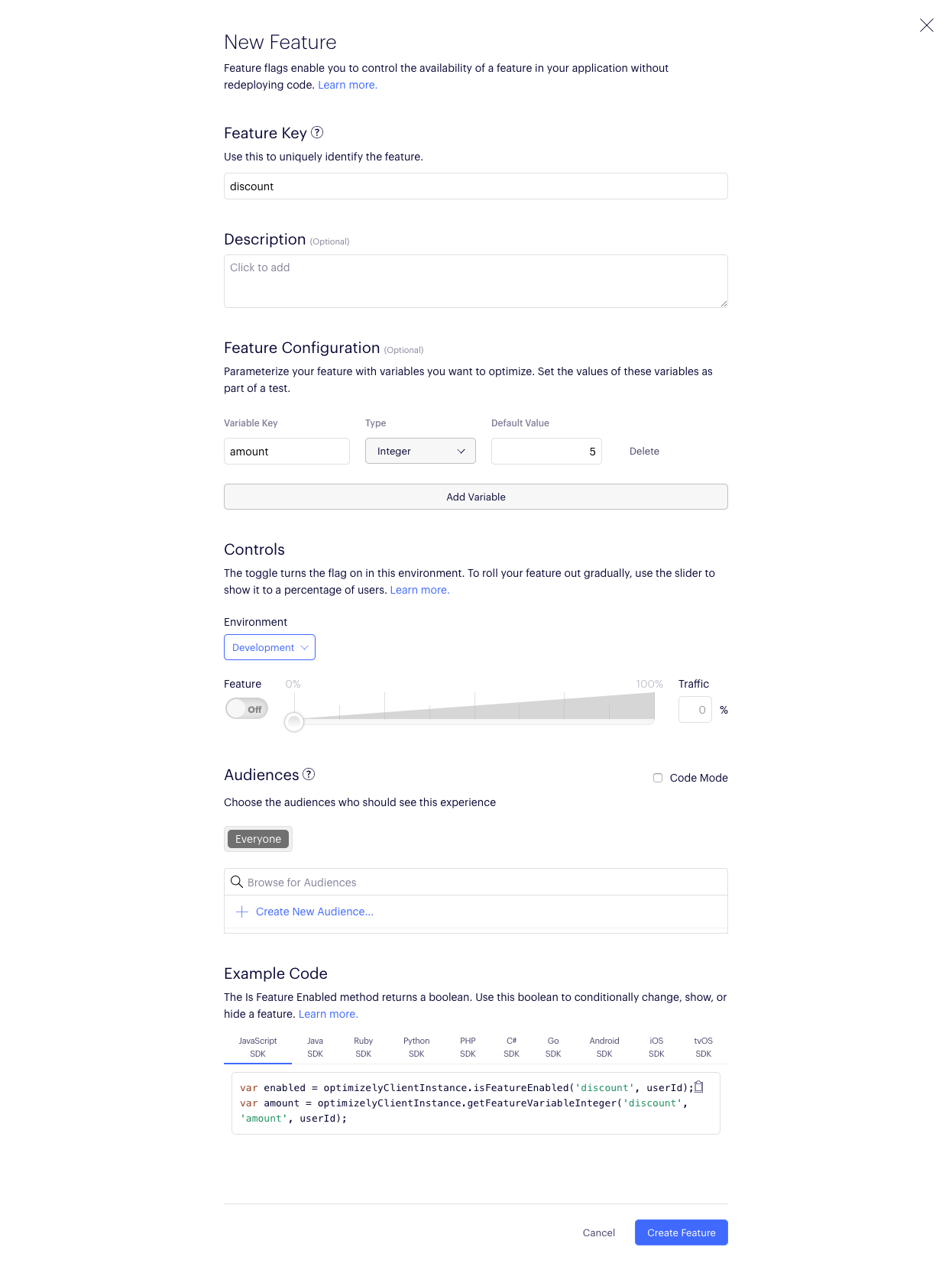
Click image to enlarge
4. Implement the feature
Next, use the SDK’s Is Feature Enabled method to determine whether to show or hide the discount feature for a specific user. You can roll out the discount feature to a percentage of traffic and run an experiment to show different discounts to just a portion of users (this makes use of the dynamic serving capability of Optimizely). Once you learn which discount value works best to increase sales, roll out the discount feature to all traffic with the amount set to the optimum value.
/// After initializing Optimizely Instance
var userId = "user_123";
var featureEnabled = optimizelyInstance.IsFeatureEnabled("discount", userId);
Even before you decide which discount amounts to test, you can code logic for exposing them. You can set the different discount values at any time in Optimizely (we’ll set them in the last step). For now, use the Get Feature Variable method to return the discount amount for a specific user.
// Continued from above example
if (featureEnabled)
{
var discountAmount = optimizelyInstance.GetFeatureVariableInteger("discount", "amount", userId);
Console.WriteLine($"{userId} got a discount of $$ {discountAmount}");
}
else
{
Console.WriteLine($"{userId} did not get the discount feature");
}
5. Create an event
In Optimizely, you will use event tracking to measure the performance of your experiments. Exposing users to new features influences them to behave in different ways. Tracking the users’ relevant actions is how we capture the impact those features had. This means we need to define which actions we want to track.
Since we are experimenting with a new discount feature it makes sense to track if the user goes on to make a purchase after they have been shown a discount.
Create an event named ‘purchase’ in your Optimizely project:
- Navigate to Events > New Event.
- Set the event key to purchase
- Click Create Event
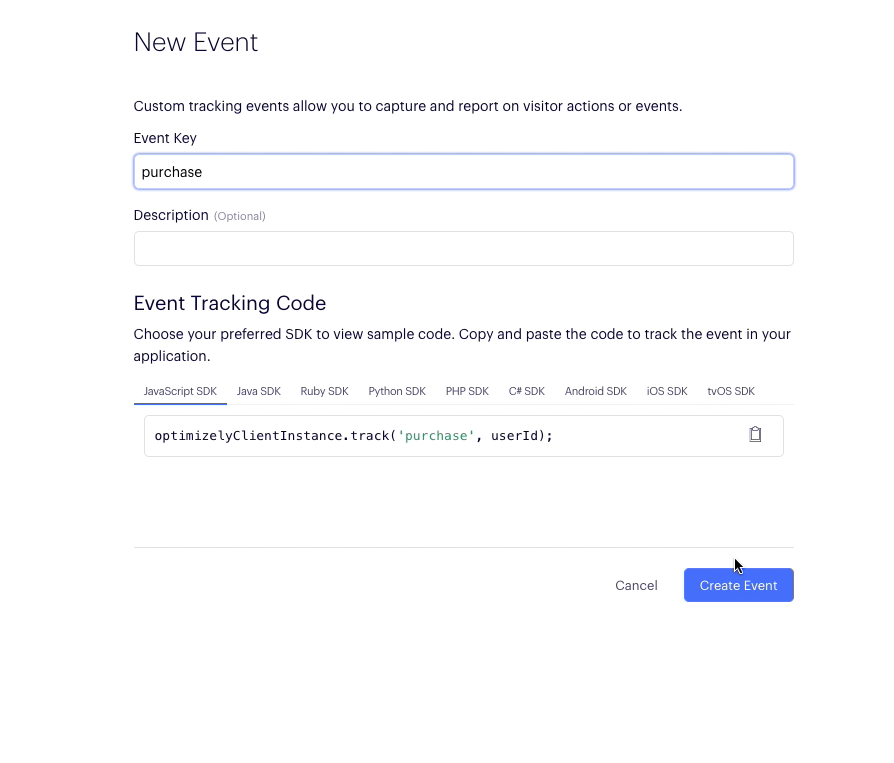
Click image to enlarge
6. Implement event tracking
You need a way to tell Optimizely when a user has made a purchase in your app and map this event in your app code to the specific event you created in Optimizely. Luckily the SDK has a method for that! Simply use the ‘track’ method on the SDK client and pass in the key for the event you created (‘purchase’) and the userId.
//after we have confirmed purchase completed
optimizelyInstance.Track("purchase", userId);
7. Launch the experiment
At this point, you have set up almost everything we need to run your Full Stack experiment. You have:
- Set up feature flag (with variable)
- Implemented dynamic serving of the feature & variable in the app
- Set up event to track the impact of the feature
- Implemented tracking of purchase event in the app
The only thing left to set up is the experiment itself! To create an experiment in your Optimizely project:
- Navigate to Experiments.
- Click Create New > Feature Test.
- In the Feature box, select the discount feature
- Click Create Feature Test.
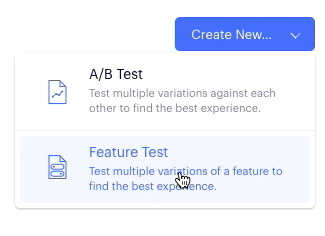
Click image to enlarge
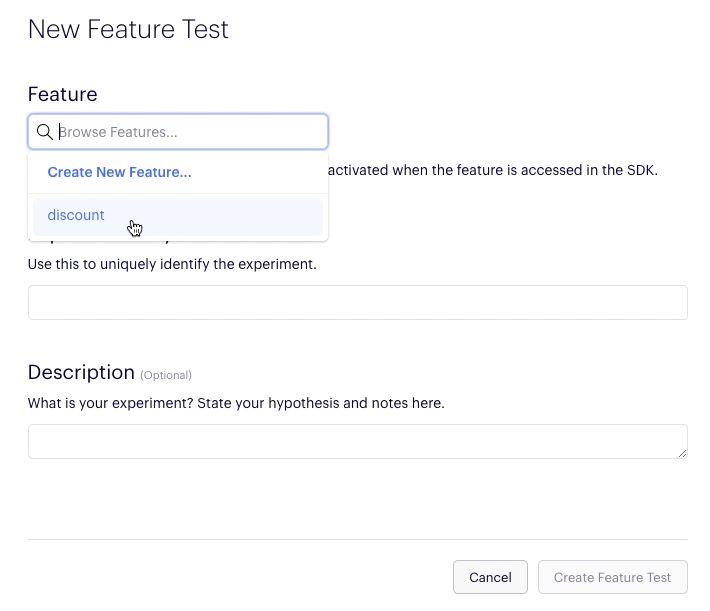
Click image to enlarge
By default, Optimizely creates an experiment with two variations: one with the feature on and one with the feature off. Toggling the feature on for both variations will cause Optimizely to show the discounts to all users who are part of the experiment (a single user only ever sees one variation).
- Toggle the feature on for both variations
- For variation_1 set the discount value to 5
- For variation_2 set the discount value to 10
- Click Save to save your changes.
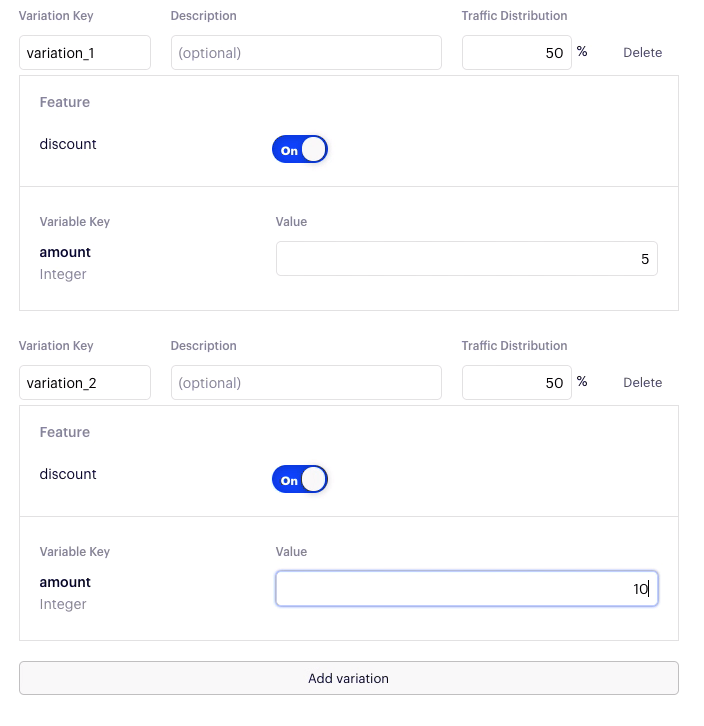
Click image to enlarge
Next, click on Metrics in the left side-panel and add your new purchase event as a metric for your experiment and save to your experiment.
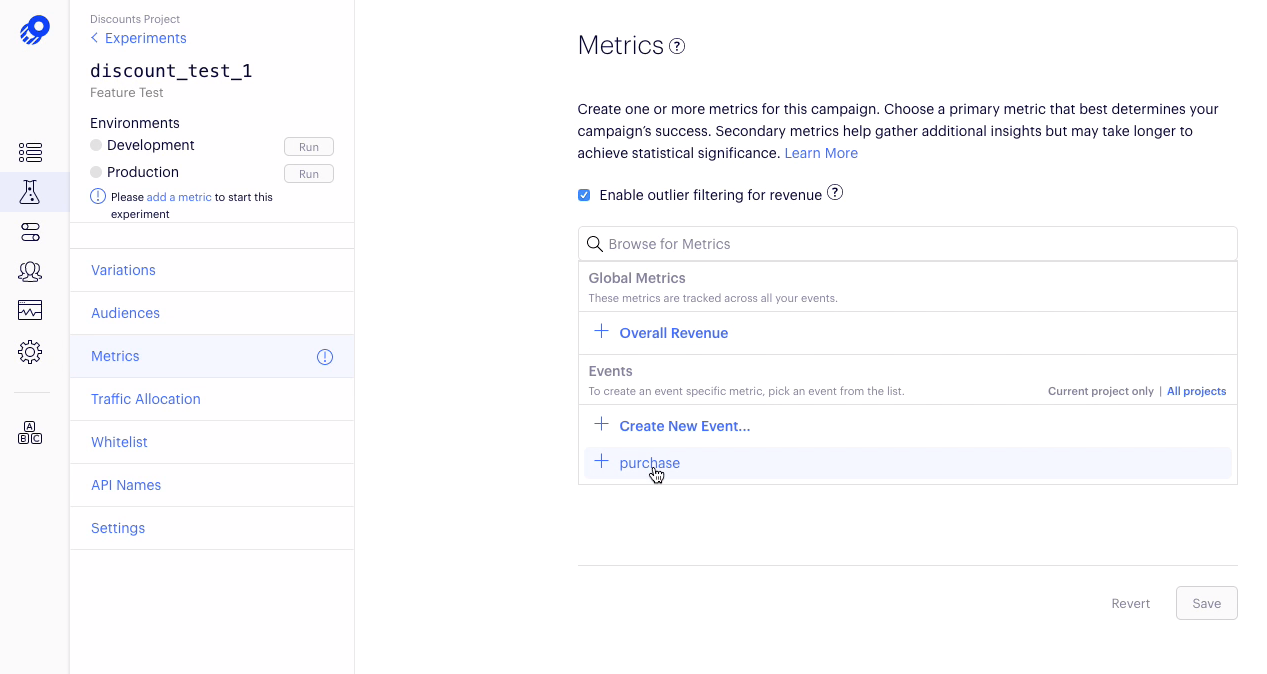
Click image to enlarge
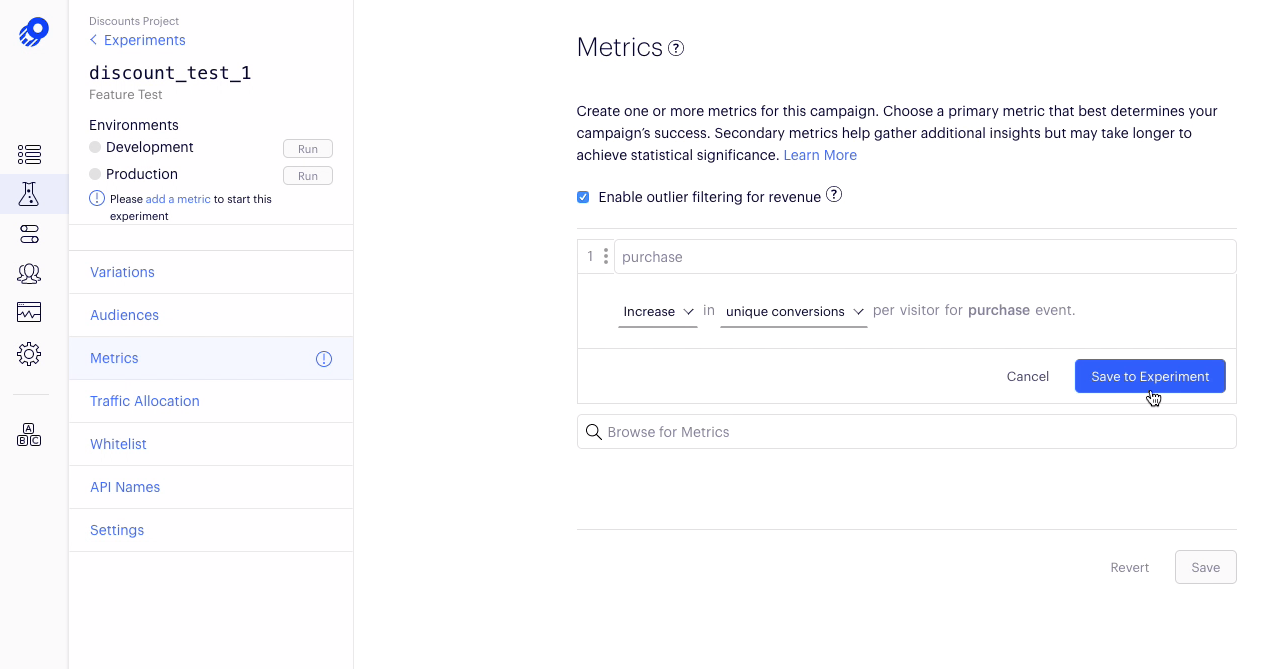
Click image to enlarge
Now click Run in the top left of the experiment page in your chosen environment and your experiment will start.
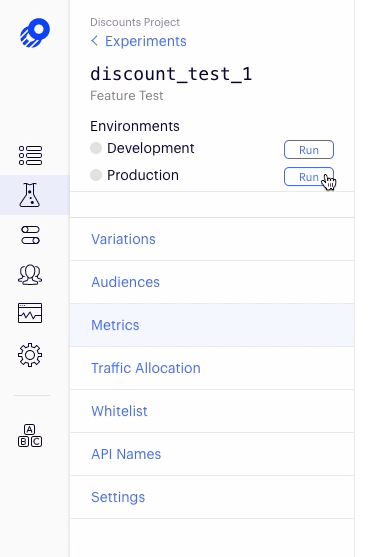
Click image to enlarge
Note
To test the different variations of the experiment, you will need to pass in unique
userIds
to the SDK methods. One way to do this is to use a random number generator to pass in randomuserIds
.
Congratulations! You have successfully set up and launched your first Optimizely Full Stack experiment. While this example focused on optimizing sales, Optimizely’s experimentation platform can support an open-ended set of experimentation use cases.
Review our documentation for more info and to learn more ways to optimize your software using experimentation.
Updated about 2 years ago