Initialize Flutter SDK
Initialize the Flutter SDK.
Use the initializeClient
method to initialize the Flutter SDK and instantiate an instance of the Optimizely SDK class. Each client corresponds to the datafile representing the state of a project for a certain environment. The datafile is accessible via OptimizelyConfig.
Version
SDK version 1.0.1 or higher
Description
The constructor accepts a configuration object to configure Optimizely.
Some parameters are optional, because the SDK provides a default implementation, but you may want to override these for your production environments. For example, you may want to set up event options to manage network calls and datafile handler parameters to set up the datafile download path or interval.
Parameters
The table below lists the required and optional parameters for the start
method:
Parameter | Type | Description |
---|---|---|
sdkKey required | String | Each environment has its own SDK key. |
eventOptions optional | EventOptions | Allows user to set batchSize , timeInterval and maxQueueSize of event processor.If a user does not set these values, the system uses the defaults of batchSize (10), timeInterval (60) and maxQueueSize (10000). |
datafilePeriodicDownloadInterval optional | int | Sets the interval (in seconds) during which DatafileHandler will attempt to update the cached datafile periodically. The minimum interval is 15 minutes (enforced by the Android JobScheduler API). |
datafileHostOptions optional | Map<ClientPlatform, DatafileHostOptions> | Adds support to provide custom datafileHost URL for different platforms. ClientPlatform is enum with two platform options iOS and android .DatafileHostOptions allows user to set datafileHostPrefix and datafileHostSuffix .For each platform, the datafileHostSuffix format is a little different. For example, Suffix for iOS should look like: /datafiles/%@.json (%@ will automatically be substituted with the sdkKey).Suffix for Android should look like: /datafiles/%s.json (%s will automatically be substituted with the sdkKey). |
Returns
Instantiates an instance of the Optimizely class.
Examples
In the Flutter SDK, you do not need to manage the datafile directly. The SDK includes a datafile manager that provides support for datafile polling to automatically update the datafile on a regular interval while the application is in the foreground.
To use it:
-
Create a
OptimizelyFlutterSdk
by supplying your SDK Key and optional configuration settings. You can find the SDK key in the Settings > Environments tab of a Full Stack project. -
To start the client synchronously and use the
initializeClient
method to instantiate a client:
// Initializing Optimizely Client
var flutterSDK = OptimizelyFlutterSdk("<Your_SDK_Key>");
var response = await flutterSDK.initializeClient();
// flag decision
var user = await flutterSDK.createUserContext("<User_ID>");
var decideResponse = await user!.decide("<Flag_Key>");
Use initialization
To instantiate the Optimizely client: synchronous. This method pings the Optimizely servers for a new datafile during initialization.
Initialization
During initialization, your app requests the newest datafile from the CDN servers. Requesting the newest datafile ensures that your app always uses the most current project settings. It also means your app cannot instantiate a client until it downloads a new datafile, discovers the datafile has not changed or the request times out.
Initializing a client synchronously causes the manager to first attempt to download the newest datafile. This network activity is what causes an initialization to take longer to complete.
If the network request returns an error, like when network connectivity is unreliable or if the manager discovers that the cached datafile is identical to the newest datafile, the Optimizely manager searches for a cached datafile. If one is available, the manager uses it to complete the client initialization, otherwise initialization fails. If the manager discovers there is an updated datafile that differs from the cached datafile, the manager downloads the new datafile and uses it to initialize the client.
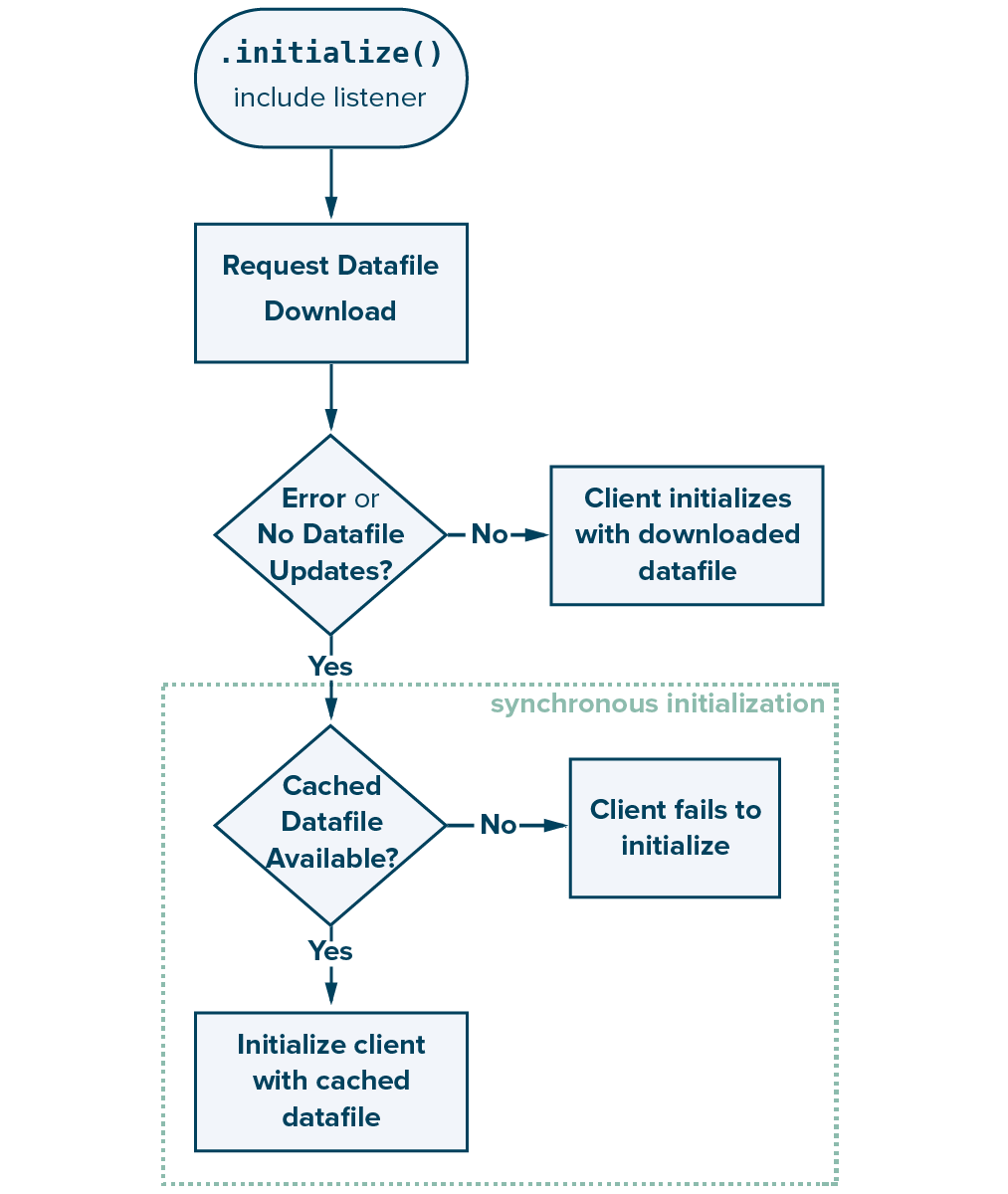
To initialize an OptimizelyClient
object, call flutterSDK.initializeClient()
.
Configure datafile polling
After the manager attempts to pull the newest datafile from the CDN servers, the Optimizely manager periodically checks for and pulls a new datafile at the time interval you set during its initialization. The process of checking for and downloading a new datafile is called datafile polling.
By default, datafile polling is enabled. You can set a non-zero interval value for increasing or decreasing the time period of polling interval. This value is the number of seconds the manager waits between datafile polling attempts.
// Datafile update interval can be configured when the SDK is configured
// Background datafile update is disabled if periodicDownloadInterval is zero
var flutterSDK = OptimizelyFlutterSdk(<Your_SDK_Key>, datafilePeriodicDownloadInterval: 15 * 60);
// every 15 minutes
Usage notes
-
The minimum polling interval is 15 minutes while the app is open. If you specify shorter polling intervals than the minimum, the SDK automatically resets the intervals to 15 minutes.
-
The Optimizely manager only checks for new datafiles when the SDK is active and the app is running on iOS/tvOS/Android.
-
The flutter SDK automatically updates when it detects a new datafile to ensure you are always working with the latest datafile. If you want to receive a notification when the project updates, register for a datafile change via the datafile change notification listener. You can also register for datafile change notifications via the UpdateConfigNotification
flutterSDK.addUpdateConfigNotificationListener((msg) {
print("got datafile change")
});
More sample code
// Initializing OptimizelyClient without optional parameters
var flutterSDK = OptimizelyFlutterSdk("<Your_SDK_Key>");
var response = await flutterSDK.initializeClient();
// flag decision
var user = await flutterSDK.createUserContext("<User_ID>");
var decideResponse = await user!.decide("<Flag_Key>");
// Initializing OptimizelyClient with optional datafilePeriodicDownloadInterval
var flutterSDK = OptimizelyFlutterSdk(sdkKey, datafilePeriodicDownloadInterval: 16*60);
var response = await flutterSDK.initializeClient();
// flag decision
var user = await flutterSDK.createUserContext("<User_ID>");
var decideResponse = await user!.decide("<Flag_Key>");
Source files
The language/platform source files containing the implementation for Flutter are available on GitHub.
Updated over 1 year ago