Multi-market examples
Describes how to use the Optimizely Customized Commerce ECF API to work with multi-market and warehouse features to change markets, get prices and discounts for markets, list warehouses and get inventories per warehouse.
Change market to display
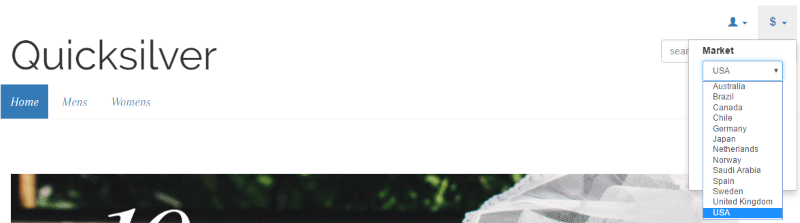
Example: Get all available markets.
public IEnumerable GetAvailableMarkets()
{
var marketService = ServiceLocator.Current.GetInstance<IMarketService>();
// Get all available markets.
return marketService.GetAllMarkets();
}
Example: Get current markets.
public IMarket GetCurrentMarket()
{
var currentMarketService = ServiceLocator.Current.GetInstance<ICurrentMarket>();
// Get current markets.
return currentMarketService.GetCurrentMarket();
}
Example: Set current markets.
public void SetCurrentMarket(MarketId marketId)
{
var currentMarketService = ServiceLocator.Current.GetInstance<ICurrentMarket>();
// Get current markets.
currentMarketService.SetCurrentMarket(marketId);
}
Display entry listing, prices, and discounts per market
Examples of displaying the entries listed with pricing and discounts for a selected market.
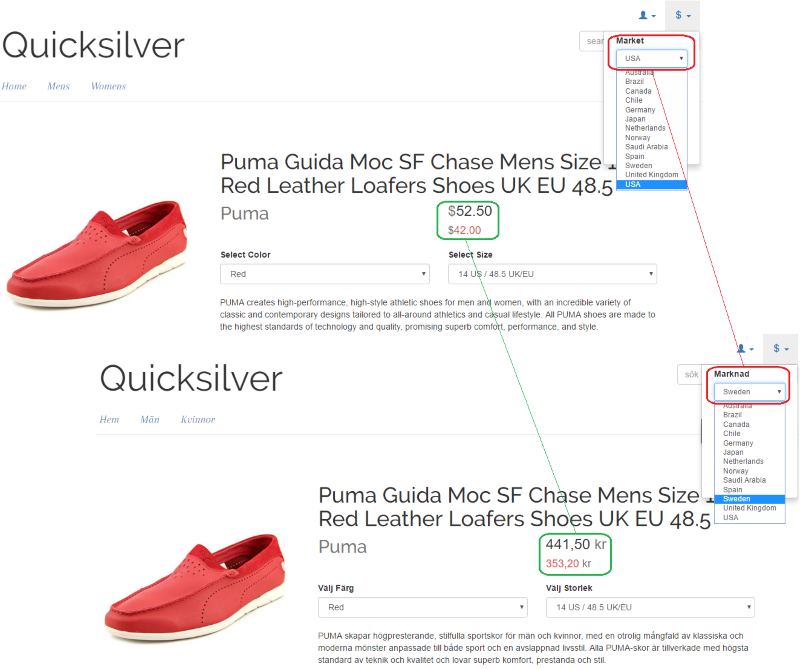
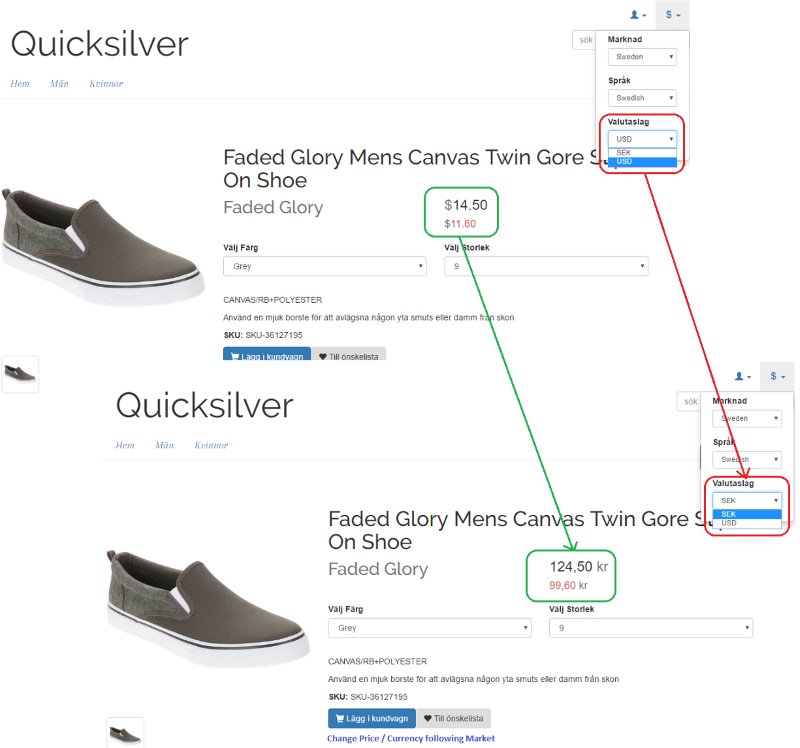
Example: Get the price for an entry per market.
Customized Commerce Version 12.8 and higher.
public Price GetSalePrice(Entry entry, decimal quantity)
{
var currentMarketService = ServiceLocator.Current.GetInstance<ICurrentMarket>();
var currentMarket = currentMarketService.GetCurrentMarket();
var currency = currentMarket.DefaultCurrency;
List<CustomerPricing> customerPricing = new List<CustomerPricing>();
customerPricing.Add(CustomerPricing.AllCustomers);
var principal = PrincipalInfo.CurrentPrincipal;
if (principal != null)
{
if (!string.IsNullOrEmpty(principal.Identity.Name))
{
customerPricing.Add(new CustomerPricing(CustomerPricing.PriceType.UserName, principal.Identity.Name));
}
CustomerContact currentUserContact = principal.GetCustomerContact();
if (currentUserContact != null && !string.IsNullOrEmpty(currentUserContact.EffectiveCustomerGroup))
{
customerPricing.Add(new CustomerPricing(CustomerPricing.PriceType.PriceGroup, currentUserContact.EffectiveCustomerGroup));
}
}
IPriceService priceService = ServiceLocator.Current.GetInstance<IPriceService>();
PriceFilter filter = new PriceFilter()
{
Quantity = quantity,
Currencies = new Currency[] { currency },
CustomerPricing = customerPricing
};
// return less price value
IPriceValue priceValue = priceService.GetPrices(currentMarket.MarketId, DateTime.UtcNow, new CatalogKey(entry.ID), filter)
.OrderBy(pv => pv.UnitPrice)
.FirstOrDefault();
if (priceValue != null)
{
return new Mediachase.Commerce.Catalog.Objects.Price(priceValue.UnitPrice);
}
return null;
}
Customized Commerce Versions 10-12.7
public Price GetSalePrice(Entry entry, decimal quantity)
{
var currentMarketService = ServiceLocator.Current.GetInstance<ICurrentMarket>();
var currentMarket = currentMarketService.GetCurrentMarket();
var currency = currentMarket.DefaultCurrency;
List<CustomerPricing> customerPricing = new List<CustomerPricing>();
customerPricing.Add(CustomerPricing.AllCustomers);
var principal = PrincipalInfo.CurrentPrincipal;
if (principal != null)
{
if (!string.IsNullOrEmpty(principal.Identity.Name))
{
customerPricing.Add(new CustomerPricing(CustomerPricing.PriceType.UserName, principal.Identity.Name));
}
CustomerContact currentUserContact = principal.GetCustomerContact();
if (currentUserContact != null && !string.IsNullOrEmpty(currentUserContact.EffectiveCustomerGroup))
{
customerPricing.Add(new CustomerPricing(CustomerPricing.PriceType.PriceGroup, currentUserContact.EffectiveCustomerGroup));
}
}
IPriceService priceService = ServiceLocator.Current.GetInstance<IPriceService>();
PriceFilter filter = new PriceFilter()
{
Quantity = quantity,
Currencies = new Currency[] { currency },
CustomerPricing = customerPricing
};
// return less price value
IPriceValue priceValue = priceService.GetPrices(currentMarket.MarketId, FrameworkContext.Current.CurrentDateTime, new CatalogKey(entry.ID), filter)
.OrderBy(pv => pv.UnitPrice)
.FirstOrDefault();
if (priceValue != null)
{
return new Mediachase.Commerce.Catalog.Objects.Price(priceValue.UnitPrice);
}
return null;
}
Example: Get discounts per market.
public Price GetDiscountPrice(ContentReference contentLink, Curreny currency)
{
var currentMarketService = ServiceLocator.Current.GetInstance<ICurrentMarket>();
var promotionEngine = ServiceLocator.Current.GetInstance<IPromotionEngine>();
var market = currentMarketService.GetCurrentMarket();
return promotionEngine.GetDiscountPrices(contentLink, market, currency).First().DiscountPrices.OrderBy(p=>p.Price).First().Price;
}
Updated about 1 year ago
Next