Exclude products from promotions
Describes how to configure filters to exclude a product from the promotion engine in Optimizely Customized Commerce. Two APIs are available for doing this.
You can exclude catalog items from all promotions, or from one promotion, using the APIs described in the following.
Components mentioned here are available in the EPiServer.Commerce.Marketing namespace.
Exclude catalog items on all promotions
Use this code to ignore catalog items when evaluating promotions and applying discounts. For example, while promotions on sneakers may be enacted from time to time, you never want the Faded Glory Mens Canvas Slip-On Shoe to receive a discount or to be included in discount-related events (such as Buy at least X items...Get the following discount).
To exclude a product from the promotion engine, implement the IEntryFilter. In most cases, you can use the EntryFilterSettings class to configure the default implementation. If that does not meet your needs, create your own IEntryFilter implementation.
The default implementation of IEntryFilter uses the EntryFilterSettings class to implement the Filter method. If using that implementation, you only need to add filters to the instance of EntryFilterSettings. The following example illustrates various ways to configure the settings.
Example
Add filters when a site is initialized.
Note
If you want the filters to work on the front end and the back end site, you must configure them on both sites.
namespace EPiServer.Reference.Commerce.Site.Infrastructure
{
[ModuleDependency(typeof(EPiServer.Commerce.Initialization.InitializationModule))]
public class SiteInitialization : IConfigurableModule
{
public void Initialize(InitializationEngine context)
{
SetupExcludedPromotionEntries(context);
}
public void ConfigureContainer(ServiceConfigurationContext context)
{
}
public void Uninitialize(InitializationEngine context)
{
}
private void SetupExcludedPromotionEntries(InitializationEngine context)
{
var filterSettings = context.Locate.Advanced.GetInstance<EntryFilterSettings>();
filterSettings.ClearFilters();
//Add filter predicates for a whole content type.
filterSettings.AddFilter<TypeThatShouldNeverBeIncluded>(x => false);
//Add filter predicates based on any property of the content type, including implemented interfaces.
filterSettings.AddFilter<IInterfaceThatCanBeImplementedToDetermineExclusion>(x => !x.ShouldBeExcluded);
//Add filter predicates based on meta fields that are not part of the content type models, e.g. if the field is dynamically added to entries in an import or integration.
filterSettings.AddFilter<EntryContentBase>(x => !(bool)(x["ShouldBeExcludedPromotionMetaField"] ?? false));
//Add filter predicates base on codes like below.
var ExcludingCodes = new string[] { "SKU-36127195", "SKU-39850363", "SKU-39101253" };
filterSettings.AddFilter<EntryContentBase>(x => !ExcludingCodes.Contains(x.Code));
}
}
}
Exclude catalog items from a promotion [New in 13.0.0]
Excluding catalog items from a promotion (discount) can be done from the Marketing user interface.
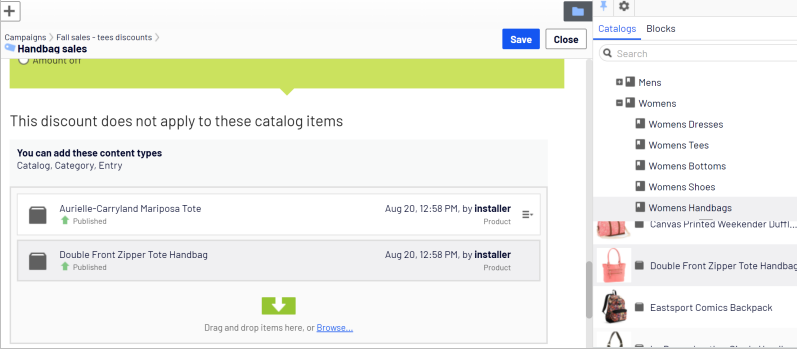
You can also set it through the API. To exclude catalog entries from a single promotion, set the promotion's ExcludedCatalogItems property to a list of ContentReference.
Example
promotion.ExcludedCatalogItems = new List<ContentReference>() { catalogLink, categoryLink, productLink };
Use ContentReferences to specify catalogs, categories, and entries.
Note
The exclusion is always recursive. If you add a category to ExcludedCatalogItems, all subcategories and their subcategories will also be excluded.
To change the default way to exclude catalog items, implement the IPromotionExcludedCatalogItemService interface, then register it in one of your initialization modules. See Initialization.
Example
public interface IPromotionExcludedCatalogItemService
{
/// <summary>
/// Gets the line item codes that are excluded by the promotion setting.
/// </summary>
/// <param name="promotionData">The promotion.</param>
/// <param name="codes">Codes of line items in the order.</param>
IEnumerable<string> GetExcludedLineItemCodes(PromotionData promotionData, IEnumerable<string> codes);
}
namespace EPiServer.Reference.Commerce.Site
{[ServiceConfiguration(typeof(IPromotionExcludedCatalogItemService), Lifecycle = ServiceInstanceScope.Singleton)]public class CustomPromotionExcludedCatalogItemService : IPromotionExcludedCatalogItemService{ IEnumerable<string> IPromotionExcludedCatalogItemService.GetExcludedLineItemCodes(PromotionData promotionData, IEnumerable<string> codes) { return Enumerable.Empty<string>(); }}
}
public void ConfigurationContainer(ServiceConfigurationContext context)
{var services = context.Services;services.RemoveAll<IPromotion.ExcludedCatalogItemsService>();services.AddSingleton<IPromotion.ExcludedCatalogItemsService, CustomPromotionExcludedCatalogItemService>();
}
Related blog post: New feature in Customized Commerce 13: Exclude catalog items per promotion
Updated about 1 year ago