Use feature flags
Feature flags, also known as feature toggles, are a software development technique that lets you turn certain functionality on and off without deploying new code. This allows for better control and more experimentation over the full lifecycle of features. You can toggle a feature off to release code quickly without exposing it to users. You can also roll it out to a fraction of your user base to minimize the impact of the launch, allowing you to validate functionality and measure performance prior to rolling out broadly.
Full Stack supports advanced feature flagging through the core concept of a feature identified by a unique key like shopping_cart
or price_filter
. You can create a new feature and manage its rollout under the Features tab, or build a feature test in the Experiments tab.
Create a feature
To create a feature:
- Click Features > Create New Feature….
- Give the feature a unique key (and optional description). Valid keys contain alphanumeric characters, hyphens, and underscores, are limited to 64 characters, and can't contain spaces. You’ll use the feature key to determine whether the feature is on or off in your code.
- Click Create Feature.
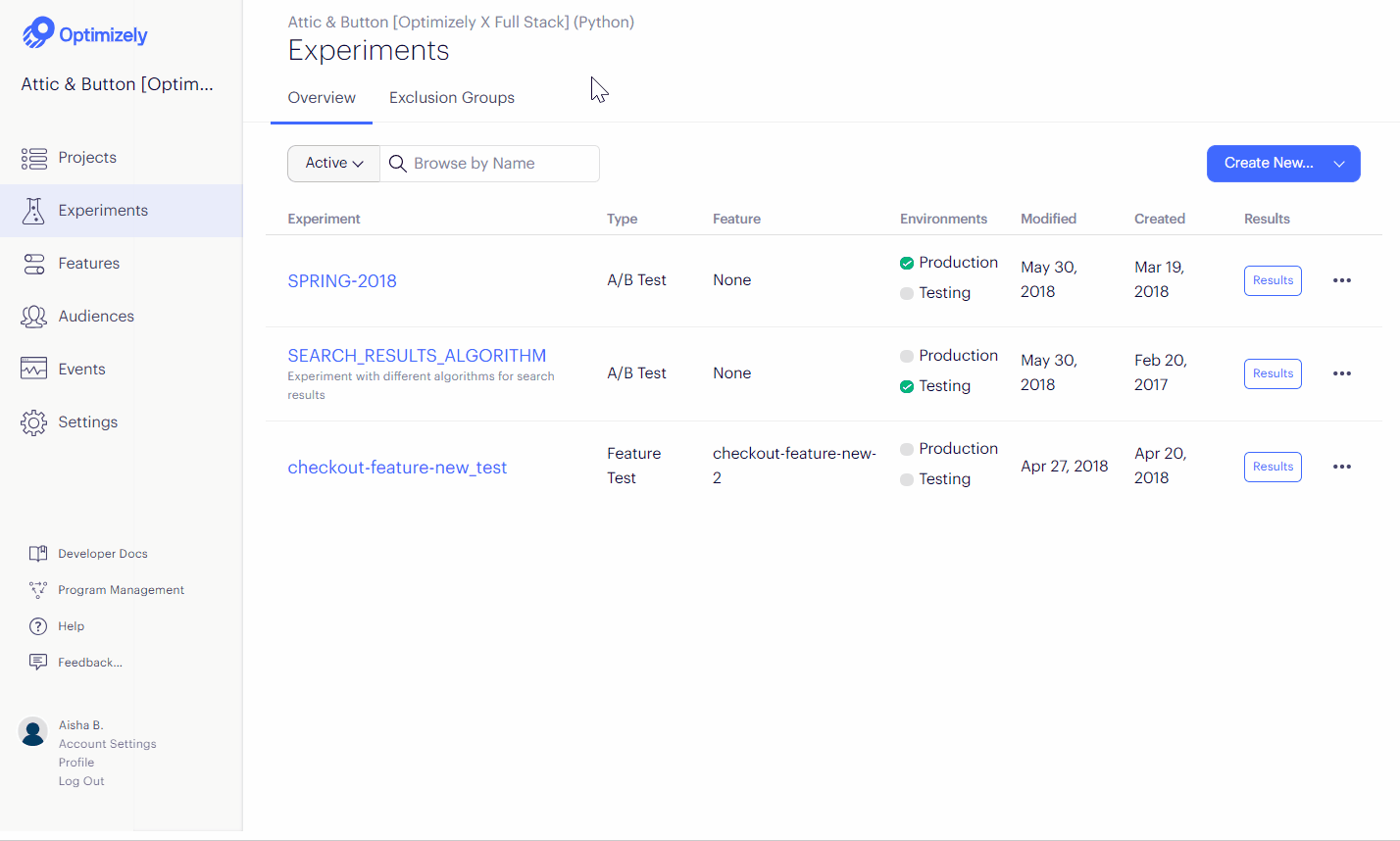
- Implement the feature flag. At the bottom of the Create New Feature modal, Optimizely automatically populates example code for an API call with your feature key:
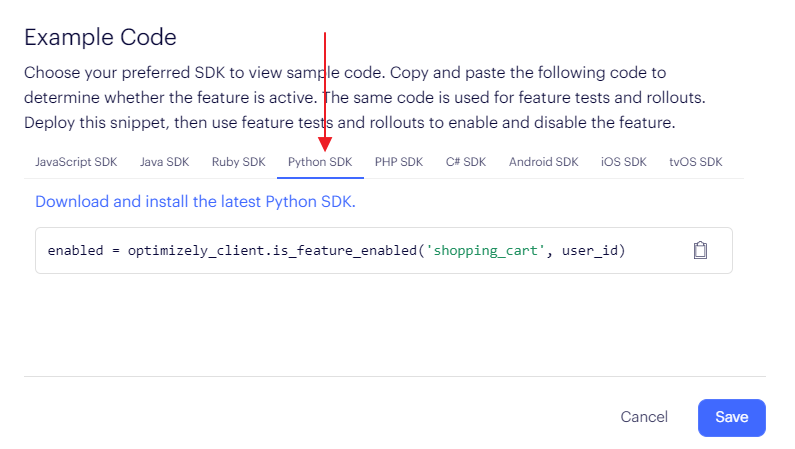
The example below shows how you can evaluate the feature flag with the Is Feature Enabled
method. This lets you build conditional branches in your code, showing the feature if enabled is true
and hiding it otherwise. You can also get a list of all the features that are enabled for a user using the Get Enabled Features
method.
The goal of these methods is to separate the process of developing and releasing code from the decision to turn a feature on. Build your feature and deploy it to your application behind this flag, then turn the feature on or off for specific users by running tests and rollouts. The value this method returns is determined by your test(s) and rollout associated with the feature. For example, if the current user is assigned to a variation in a feature test for which the feature is on, the method returns true
. Otherwise, it returns false
.
import com.optimizely.ab.android.sdk.OptimizelyClient;
// Evaluate a feature flag and a variable
Boolean enabled = optimizelyClient.isFeatureEnabled("price_filter", userId);
Integer minPrice = optimizelyClient.getFeatureVariableInteger("price_filter", "min_price", userId);
using OptimizelySDK;
var optimizelyClient = new Optimizely(datafile);
string userId = "";
// Evaluate a feature flag and a variable
bool enabled = optimizelyClient.IsFeatureEnabled("price_filter", userId);
int? min_price = optimizelyClient.GetFeatureVariableInteger("price_filter", variableKey: "min_price", userId: userId);
import com.optimizely.ab.Optimizely;
// Evaluate a feature flag and a variable
Boolean enabled = optimizelyClient.isFeatureEnabled("price_filter", userId);
Integer minPrice = optimizelyClient.getFeatureVariableInteger("price_filter", "min_price", userId);
// Evaluate a feature flag and a variable
var enabled = optimizelyClientInstance.isFeatureEnabled('price_filter', userId);
var min_price = optimizelyClientInstance.getFeatureVariableInteger('price_filter', 'min_price', userId);
// Evaluate a feature flag and a variable
var userId = `test${Date.now()}.${Math.random()}`;
const enabled = optimizelyClient.isFeatureEnabled("price_filter", userId);
console.log(`Price filter enabled: ${enabled}`);
if (enabled) {
const minPrice = optimizelyClient.getFeatureVariableInteger(
"price_filter",
"min_price",
userId
);
console.log(`Min price: ${minPrice}`);
}
// Evaluate a feature flag and a variable
bool enabled = [client isFeatureEnabled:@"price_filter" userId: userId];
int min_price = [[client getFeatureVariableInteger:@"price_filter" userId: userId] integerValue];
// Evaluate a feature flag and a variable
$enabled = $optimizelyClient->isFeatureEnabled('price_filter', $userId);
$min_price = $optimizelyClient->getFeatureVariableInteger('price_filter', 'min_price', $userId);
from optimizely import optimizely
optimizely_client = optimizely.Optimizely(datafile)
# Evaluate a feature flag and variable
enabled = optimizely_client.is_feature_enabled('price_filter', user_id)
min_price = optimizely_client.get_feature_variable_integer('price_filter', 'min_price', user_id)
# Evaluate a feature flag and a variable
enabled = optimizely_client.is_feature_enabled('price_filter', user_id)
min_price = optimizely_client.get_feature_variable_integer('price_filter', 'min_price', user_id)
// Evaluate a feature flag and a variable
let enabled = optimizelyClient?.isFeatureEnabled("price_filter", userId: userId)
let min_price = optimizelyClient?.getFeatureVariableInteger("price_filter", "min_price", userId: userId)?.intValue
Configuration variables
In addition to binary flagging, features also support variables for remote configuration. This lets you parameterize the feature and configure its settings without deploying new code. For example, you can tune the parameters of a search algorithm or the display of a user interface.
Toggles and rollouts
Feature flags support remote toggling, so you can turn a feature on or off directly from the Optimizely dashboard. This means you can deploy code silently, then toggle an individual feature on without impacting other features in your release. It also supports incremental rollouts so you can launch a feature to a targeted subset your users. For example, you can launch a new feature to a random 10% of all users, or to a specific audience of beta testers. If anything goes wrong, you can immediately roll back without redeploying your app.
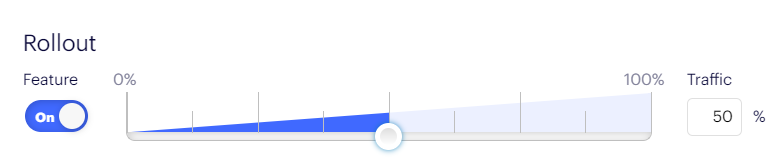
See Rollout and rollback features.
Feature tests
Full Stack enables you to experiment on the features you build. You can build a feature test to measure whether your feature raises key metrics, or if it actually hurts them. For example, did a redesigned homepage lead to higher revenue, or did it actually slow down the app? You can also test different configurations against each other to find the ideal combination of variables to show different users.
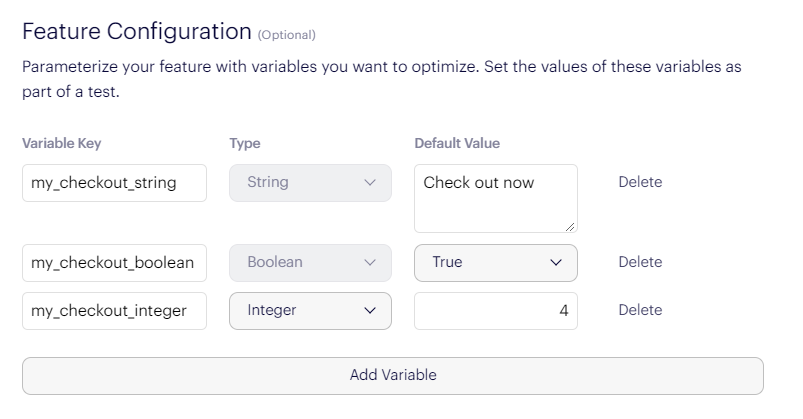
See Run feature tests.
Updated about 1 year ago