Get the datafile
The datafile is a JSON representation of your Full Stack project. It contains all the instructions needed to run your experiments.
The datafile is updated immediately after changes to your experiments, but it may take a few minutes to upload to the content delivery network (CDN). If you’re experiencing delays longer than 5 minutes, please contact Optimizely support.
In general, you don’t need to access the datafile, but it can be handy if your experiments don’t update as expected. The datafile can help you debug a Full Stack experiment and confirm expected updates.
See also Datafile versioning and management.
Note
If you're using the iOS or Android SDKs, skip straight to Initialize a mobile SDK, which encompasses getting the datafile and instantiating a client.
Important
Be careful changing the datafile's environment key as it will impact the datafile URL your code loads. The environment key displays on the Settings > Datafile page; see the image in Access the datafile via the app.
Get the datafile
Before you instantiate a client, get a local copy of the datafile from our servers. Synchronizing this local copy lets the SDK make immediate decisions on experiments, without waiting for a blocking network request.
string datafile = string.Empty;
var url = "https://cdn.optimizely.com/json/12345.json";
using(var webClient = new System.Net.WebClient()){
// To refresh on every request
webClient.CachePolicy = new System.Net.Cache.RequestCachePolicy(System.Net.Cache.RequestCacheLevel.NoCacheNoStore);
datafile = webClient.DownloadString(url);
}
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
try {
String url = "https://cdn.optimizely.com/datafiles/QMVJcUKEJZFg8pQ2jhAybK.json";
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpGet httpget = new HttpGet(url);
String datafile = EntityUtils.toString(
httpclient.execute(httpget).getEntity());
}
catch (Exception e) {
System.out.print(e);
return;
}
const url = 'https://cdn.optimizely.com/json/12345.json';
window
.fetch(url, { mode: 'cors' })
.then(response => response.json())
.then(datafile => {
const optimizelyClientInstance = optimizelySdk.createInstance({
datafile,
});
});
// Minimal client
const optimizely = require("@optimizely/optimizely-sdk");
const rp = require("request-promise");
// TODO: replace with your datafile URL
const DATAFILE_URL =
"https://cdn.optimizely.com/datafiles/QMVJcUKEJZFg8pQ2jhAybK.json";
const datafile = await rp({ uri: DATAFILE_URL, json: true });
console.log("Datafile:", datafile);
var optimizelyClient = optimizely.createInstance({
datafile
});
// Use optimizelyClient to run experiments
use Optimizely\Optimizely;
$url = 'https://cdn.optimizely.com/datafiles/abcdEFGH123.json';
$datafile = file_get_contents($url);
// Instantiate an Optimizely client
$optimizelyClient = new Optimizely($datafile);
from optimizely import optimizely
import requests
url = 'https://cdn.optimizely.com/datafiles/abcdEFGH123.json'
datafile = requests.get(url).text
optimizely_client = optimizely.Optimizely(datafile)
require 'optimizely'
require 'httparty'
url = 'https://cdn.optimizely.com/datafiles/QMVJcUKEJZFg8pQ2jhAybK.json'
datafile = HTTParty.get(url).body
optimizely_client = Optimizely::Project.new(datafile)
How you synchronize and how often you synchronize depends on your application:
- Recommended method: Use webhooks to notify your server when the datafile changes so it can retrieve the latest version.
- Alternative method: Poll the CDN or REST API at a regular interval (such as every minute) for an updated datafile. The length of this interval will vary according to your application requirements.
Note
Be sure to synchronize the datafile often enough to stay current with changes in the Optimizely project environment without generating unacceptable network traffic and latency. For more information on how to balance network latency with datafile freshness, see Datafile versioning and management.
Fetch the datafile via REST API
You can access the datafile via Optimizely's authenticated REST API. You need to authenticate with an API token. For more information, see Read the datafile of an environment.
Access the datafile via the app
To access the datafile for your Full Stack project:
- Navigate to Settings > Environments.
- Find the SDK Key/Primary URL for the project and environment whose datafile you want to access.
- Click the SDK Key/Primary URL to open the CDN link in a new browser tab or window.
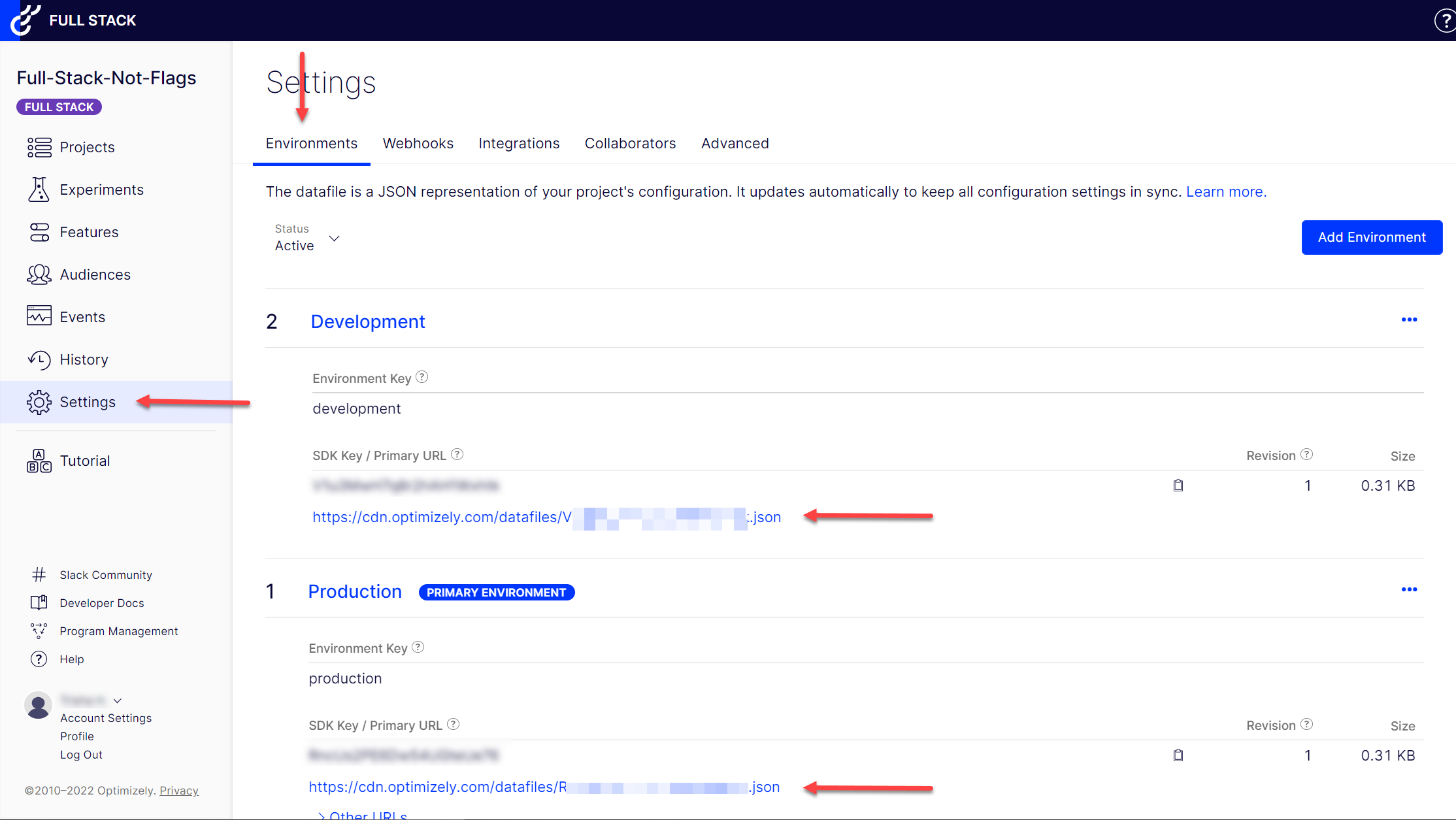
View the datafile via the CDN link
This image shows how the datafile appears when accessed from the CDN link.
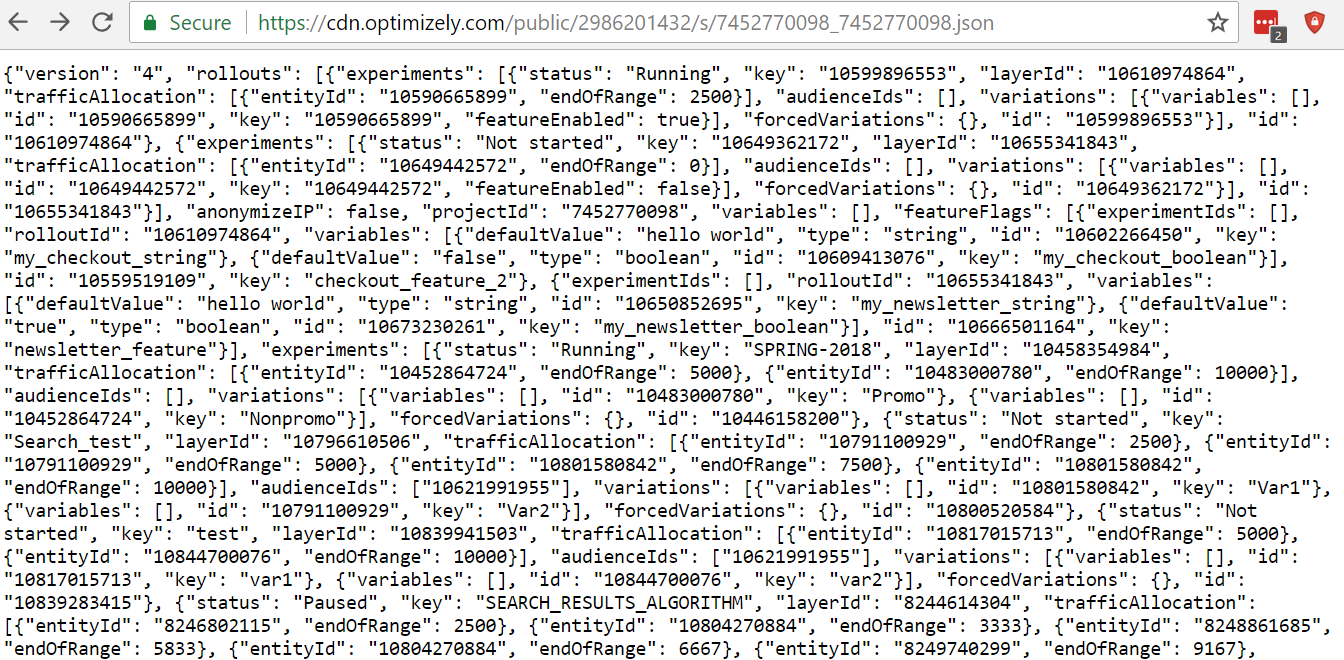
See Example datafile to see an example in properly formatted JSON.
Updated about 1 year ago