Define feature variables
A feature configuration is a set of variables associated with a feature that you can set as part of a feature test. Defining feature variables allows you to iterate on your feature without redeploying the code. Run feature tests to determine the optimal combination of variable values, then set those values as your default feature configuration and launch using a rollout.
Add a feature configuration
To add feature configuration variables:
- On the Features dashboard, click the name of the feature you want to configure.
- Click Add Variable.
- Type a unique variable key, select a type, and enter a default value for each variation in the configuration.
- Click Add Variable to add another variable.
- Click Save when you are finished adding variables for your feature.
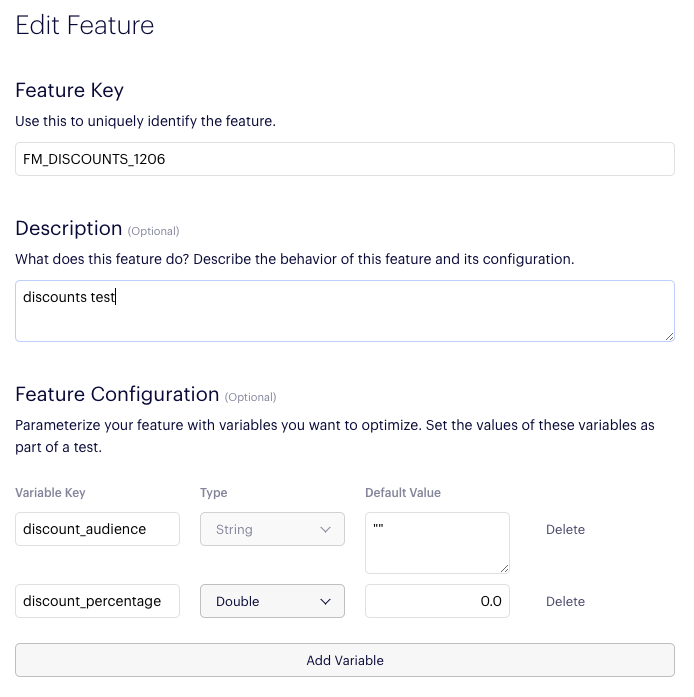
- Deploy the feature configuration.
When you add a feature configuration, Optimizely updates the feature’s example code to include feature variable accessors. The image shows how variable values are dynamically assigned if the feature flag is active.
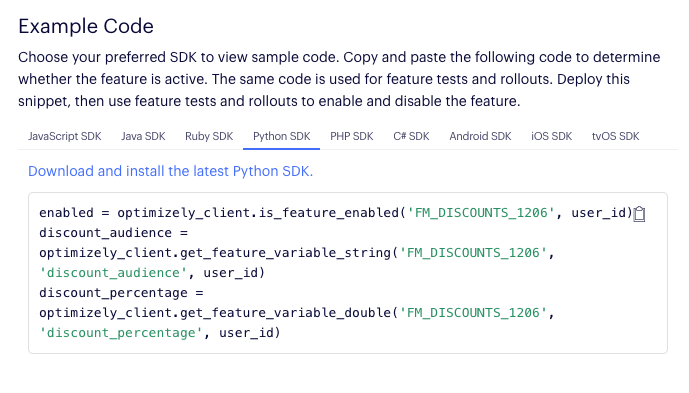
The SDKs offer different Get Feature Variable methods based on the variable's datatype (boolean, double, integer, or string). The maximum length of a string variable is 1500 characters.
The example shows how variable values are dynamically assigned if the feature flag is active.
/**
* Look for getFeatureVariableString() and getFeatureVariableInteger()
* in the following code.
*
* Fetch feature flag value for the "sorting_algorithm" feature.
* Then specify default value for feature config variable.
* Here we use two different variable values, essentially creating
* a multi-variate test so we can test out the combinatorial effects
* of the various configurations
*/
Boolean isSortingAlgoEnabled = optimizelyClient.isFeatureEnabled(
"sorting_algorithm",
"user-1"
);
String sortingProperty = "name";
Integer pageLimit = 10;
if (isSortingAlgoEnabled) {
/**
* If feature is enabled, fetch dynamic feature config value to toggle
* the property used to sort items. The value of the variable is determined
* by the value specified by the Feature Test or Feature Rollout that
* "user-1" was assigned.
*/
sortingProperty = optimizelyClient.getFeatureVariableString(
"sorting_algorithm",
"sorting_propery",
"user-1"
);
pageLimit = optimizelyClient.getFeatureVariableInteger(
"sorting_algorithm",
"page_limit",
"user-1"
);
}
/**
* Fetch products and pass the sorting property as well as the number
* of products to fetch
*/
ArrayList<Product> products = productProvider.get(sortingProperty, pageLimit);
/**
* Look for GetFeatureVariableString() and GetFeatureVariableInteger()
* in the following code.
*
* Fetch feature flag value for the "sorting_algorithm" feature.
* Then specify default value for feature config variable.
* Here we use two different variable values, essentially
* creating a multi-variate test so we can test out the
* combinatorial effects of the various configurations
*/
var optimizelyClient = new Optimizely(datafile);
bool isSortingAlgoEnabled = optimizelyClient.IsFeatureEnabled(
"sorting_algorithm",
"user-1"
);
string sortingProperty = "name";
int? pageLimit = 10;
if (isSortingAlgoEnabled)
{
/**
* If feature is enabled, fetch dynamic feature config value to toggle
* the property used to sort items. The value of the variable is determined
* by the value specified by the Feature Test or Feature Rollout that
* "user-1" was assigned.
*/
sortingProperty = optimizelyClient.GetFeatureVariableString(
"sorting_algorithm",
"sorting_propery",
"user-1"
);
pageLimit = optimizelyClient.GetFeatureVariableInteger(
"sorting_algorithm",
"page_limit",
"user-1"
);
}
/**
* Fetch products and pass the sorting property as well as the number of
* products to fetch
*/
ArrayList<Product> products = productProvider.Get(sortingProperty, pageLimit);
/**
* Look for getFeatureVariableString() and getFeatureVariableInteger()
* in the following code.
*
* Fetch feature flag value for the "sorting_algorithm" feature.
* Then specify default value for feature config variable.
* Here we use two different variable values, essentially creating
* a multi-variate test so we can test out the combinatorial effects
* of the various configurations
*/
Boolean isSortingAlgoEnabled = optimizelyClient.isFeatureEnabled(
"sorting_algorithm",
"user-1"
);
String sortingProperty = "name";
Integer pageLimit = 10;
if (isSortingAlgoEnabled) {
/**
* If feature is enabled, fetch dynamic feature config value to toggle
* the property used to sort items. The value of the variable is determined
* by the value specified by the Feature Test or Feature Rollout that
* "user-1" was assigned.
*/
sortingProperty = optimizelyClient.getFeatureVariableString(
"sorting_algorithm",
"sorting_propery",
"user-1"
);
pageLimit = optimizelyClient.getFeatureVariableInteger(
"sorting_algorithm",
"page_limit",
"user-1"
);
}
/**
* Fetch products and pass the sorting property as well as the number
* of products to fetch
*/
ArrayList<Product> products = productProvider.get(sortingProperty, pageLimit);
/**
* Look for getFeatureVariableString() and getFeatureVariableInteger()
* in the following code.
*
* Fetch feature flag value for the 'sorting_algorithm' feature.
* Then specify default value for feature config variable.
* Here we use two different variable values, essentially creating
* a multi-variate test so
* we can test out the combinatorial effects of the various
* configurations.
*/
const isSortingAlgoEnabled = optimizelyClient.isFeatureEnabled(
'sorting_algorithm',
'user-1'
)
let sortingProperty = 'name'
let pageLimit = 10
if (isSortingAlgoEnabled) {
/**
* If feature is enabled, fetch dynamic feature config value to toggle
* the property used to sort items. The value of the variable is determined
* by the value specified by the Feature Test or Feature Rollout that
* 'user-1' was assigned.
*/
sortingProperty = optimizelyClient.getFeatureVariableString(
'sorting_algorithm',
'sorting_propery',
'user-1'
)
pageLimit = optimizelyClient.getFeatureVariableInteger(
'sorting_algorithm',
'page_limit',
'user-1'
)
}
/**
* Fetch products and pass the sorting property as well as the number of
* products to fetch.
*/
const products = productProvider.get(sortingProperty, pageLimit)
/**
* Look for getFeatureVariableString() and getFeatureVariableInteger()
* in the following code.
*
* Fetch feature flag value for the 'sorting_algorithm' feature.
* Then specify default value for feature config variable.
* Here we use two different variable values, essentially creating
* a multi-variate test so
* we can test out the combinatorial effects of the various
* configurations.
*/
const isSortingAlgoEnabled = optimizelyClient.isFeatureEnabled(
"sorting_algorithm",
userId
);
let sortingProperty = "name";
let pageLimit = 10;
if (isSortingAlgoEnabled) {
/**
* If feature is enabled, fetch dynamic feature config value to toggle
* the property used to sort items. The value of the variable is determined
* by the value specified by the Feature Test or Feature Rollout that
* 'user-1' was assigned.
*/
sortingProperty = optimizelyClient.getFeatureVariableString(
"sorting_algorithm",
"sorting_propery",
userId
);
pageLimit = optimizelyClient.getFeatureVariableInteger(
"sorting_algorithm",
"page_limit",
userId
);
}
/**
* Fetch products and pass the sorting property as well as the number of
* products to fetch.
*/
// TODO: implement me
// const products = productProvider.get(sortingProperty, pageLimit);
- (DiscountAudience *)getDiscountAudience:(OPTLYManager *)manager {
DiscountAudience *discountAudience = [[DiscountAudience alloc] init:@"general"];
if ([manager getOptimizely]) {
NSString *domain = @"";
NSRange range = [self.userId rangeOfString:@"@"];
if ( range.length > 0 ) {
domain = [self.userId substringFromIndex:(range.location + range.length)];
}
NSString * audienceType = [[manager getOptimizely] getFeatureVariableString:@"discountAudienceFeatureKey" variableKey:@"discountAudienceFeatureVariableKey" userId:self.userId attributes:@{@"domainAttr": domain}];
discountAudience = [[DiscountAudience alloc] init:audienceType];
}
return discountAudience;
}
/**
* Look for get_feature_variable_string() and
* get_feature_variable_integer() in the following code.
*
* Fetch feature flag value for the 'sorting_algorithm' feature.
* Then specify default value for feature config variable.
* Here we use two different variable values, essentially creating
* a multi-variate test so we can test out the combinatorial effects
* of the various configurations.
*/
$isSortingAlgoEnabled = $optimizelyClient->isFeatureEnabled(
'sorting_algorithm',
'user-1'
);
$sortingProperty = 'name';
$pageLimit = 10;
if ($isSortingAlgoEnabled) {
/**
* If feature is enabled, fetch dynamic feature config value to toggle
* the property used to sort items. The value of the variable is determined
* by the value specified by the Feature Test or Feature Rollout that 'user-1'
* was assigned.
*/
$sortingProperty = $optimizelyClient->get_feature_variable_string(
'sorting_algorithm',
'sorting_propery',
'user-1'
);
$pageLimit = $optimizelyClient->get_feature_variable_integer(
'sorting_algorithm',
'page_limit',
'user-1'
);
}
/**
* Fetch products and pass the sorting property as well as the number of
* products to fetch.
*/
$products = $productProvider->get($sortingProperty, $pageLimit);
# Look for get_feature_variable_string() and
# get_feature_variable_integer() in the following code.
# Fetch feature flag value for the 'sorting_algorithm' feature.
# Then specify default value for feature config variable.
# Here we use two different variable values, essentially creating
# a multi-variate test so we can test out the combinatorial effects
# of the various configurations.
is_sorting_algo_enabled = optimizely_client.is_feature_enabled(
'sorting_algorithm',
'user-1'
)
sorting_property = 'name'
page_limit = 10
if is_sorting_algo_enabled:
# If feature is enabled, fetch dynamic feature config value to toggle
# the property used to sort items. The value of the variable is determined
# by the value specified by the Feature Test or Feature Rollout that
# 'user-1' was assigned.
sorting_property = optimizely_client.get_feature_variable_string(
'sorting_algorithm',
'sorting_propery',
'user-1'
)
page_limit = optimizely_client.get_feature_variable_integer(
'sorting_algorithm',
'page_limit',
'user-1'
)
# Fetch products and pass the sorting property as well as the number of
# products to fetch.
products = product_provider.get(sorting_property, page_limit)
# Look for get_feature_variable_string() and get_feature_variable_integer()
# in the following code.
# Fetch feature flag value for the 'sorting_algorithm' feature.
# Then specify default value for feature config variable.
# Here we use two different variable values, essentially creating a
# multi-variate test so we can test out the combinatorial effects of
# the various configurations.
is_sorting_algo_enabled = optimizely_client.is_feature_enabled(
'sorting_algorithm',
'user-1'
)
sorting_property = 'name'
page_limit = 10
if is_sorting_algo_enabled
# If feature is enabled, fetch dynamic feature config value to toggle
# the property used to sort items. The value of the variable is determined
# by the value specified by the Feature Test or Feature Rollout that
# 'user-1' was assigned.
sorting_property = optimizely_client.get_feature_variable_string(
'sorting_algorithm',
'sorting_propery',
'user-1'
)
page_limit = optimizely_client.get_feature_variable_integer(
'sorting_algorithm',
'page_limit',
'user-1'
)
end
# Fetch products and pass the sorting property as well as the number of
# products to fetch.
products = product_provider.get(sorting_property, page_limit)
// Look for getFeatureVariableString() in the following code.
// Sample usage
func discountAudience() -> DiscountAudience {
var audience = DiscountAudience.general
guard let userId = UserDefaults.user?.email,
let _optimizelyClient = self.optimizelyClient
else { return audience }
let domain = userId.components(separatedBy: "@").last ?? ""
let audienceType = _optimizelyClient.getFeatureVariableString(self.discountAudienceFeaturekey,
variableKey: self.discountAudienceFeatureVariableKey,
userId: userId,
attributes: [domainAttributeKey: domain])
audience = DiscountAudience.init(fromString: audienceType ?? "")
return audience
}
Updated over 1 year ago