Command Manager
Describes how to work with Command Manager to handle actions in Commerce Manager in Optimizely Commerce Connect 13.
Note
This content does not apply to Commerce Connect versions 14+.
CommandManager is a class for handling actions in Commerce Manager. The following command types are available:
- Navigate – Navigation to another page.Â
- OpenWindow – Open popup in new window.
- OpenFrameModalPopup – Open modal popup (IFrame) in current window.
- ServerAction – Server action (POST) with calling of special server handler.
- ClientAction – Client action with calling of predefined JavaScript.
Commands defined in XML files
Commands are defined in the View/Commands section of an XML file and look like this:
<Command id="Command_Name1">
<CommandType>Navigate</CommandType>
...
</Command>
The following example shows how to bind a command to a toolbar button simply by adding the commandName attribute with the command identifier to Button.Â
<Button id="Button1" text="button text" commandName="Command_Name1"></Button>
Navigate
The navigate-type command can contain the following tags:
- Url (string) – URL to page for navigation.
- Target (string) – Navigate to named frame.
- EnableHandler – Server handler in the format "className, assemblyName" defining accessibility of the command.
The server handler must implement the ICommandEnableHandler interface.
ICommandEnableHandler interface
public class CanAdminHandler : ICommandEnableHandler
{
public bool IsEnable(object Sender, object Element)
{
bool retval = false;
return retval;
}
}
Example:
<Command id="MC_ListApp_ManageList">
<CommandType>Navigate</CommandType>
<Url>~/Apps/ListApp/ListInfoView.aspx?class={QueryString:ViewName}</Url>
</Command>
Dynamic parameters for Url parameter
You can use the following templates as dynamic parameters for the Url parameter.
- {QueryString:KeyName} – Get value for KeyName parameter from QueryString for current page.
- {HttpContext:KeyName} – Get value for KeyName from HttpContext.
- {Session:KeyName} – Get value from KeyName from ASP.NET Session.
- {DataContext:KeyName} – Get value from KeyName from DataContext.
- {Security:KeyName} – Get value from security context. KeyName can get the following values:
- CurrentUser (CurrentUserId) – ID of current user.
- {DateTime:KeyName} – Get date value. Keyname can get following values for date:
- Today (TodayStart) – Starting of current day.
- ThisWeek (ThisWeekyStart) – Starting of current week.
- ThisMonth (ThisMonthStart) – Starting of current month.
- ThisYear (ThisYearStart) – Starting of current year.
- Yesterday (YesterdayStart) – Starting of previous day.
- LastWeek (LastWeekStart) – Starting of previous week.
- LastMonth (LastMonthStart) – Starting of previous month.
- LastYear (LastYearStart) – Starting of previous year.
- TodayEnd – End for current day.
- ThisWeekEnd – End of current week.
- ThisMonthEnd – End of current month.
- ThisYearEnd – End of current year.
- YesterdayEnd – End of previous day.
- LastWeekEnd – End of previous week.
- LastMonthEnd – End of previous month.
- LastYearEnd – End of previous year.
- [ClientParam:ParameterName] – Gets parameter name from CommandParameters collection, for example [ClientParam:PrimaryKeyId].
OpenWindow
The OpenWindow-type command can contain the following tags:
- Url (string) – Url to page for opening in pop-up window. You can use the same templates as in the Navigate-type command.
- Width (int) – Width in pixels for window(default, 640).
- Height (int) – Height in pixels for window(default, 480).
- Left (int) – Left position for window (show in center by default).
- Top (int) – Top position for window(show in center by default).
- Scroll (True/False) – Allow scroll bar for window or not.
- Resize (True/False) – Allow resize for window or not.
- RefreshMethod (string) – JavaScript function name with one parameter, which will be called after window close.
- UpdatePanelIds (string) – List of UpdatePanels IDs, which necessary to update after window close.
- EnableHandler (string) – Server handler in format "className, assemblyName" which define accessibility of the command.
In WEB, you do not have a standard use-case for monitoring closed pop-up windows, so you need to take additional actions. To update the parent window, run the following code:
CommandManager.RegisterRefreshParentWindowScript(this.Page, param);
The param string parameter is sent to the JavaScript function; if you do not need any parameters for the client side, send String.Empty.
Use the following code to close the current pop-up windows and refresh parent windows:
CommandManager.RegisterCloseOpenedWindowScript(this.Page, param);
For updating UpdatePanels in the parent window (which are defined in the XML description), run the following code.
Update UpdatePanels
CommandParameters cp = new CommandParameters(commandId);
CommandManager.RegisterRefreshParentWindowScript(this.Page, cp.ToString());
or
string command = String.Empty;
if (!String.IsNullOrEmpty(CommandName))
{
CommandParameters cp = new CommandParameters(CommandName);
command = cp.ToString();
}
The commandId command name is mapped from <Commandid=""> in the XML.
commandId command name
<Command id="MC_TimeTracking_MultipleAdd">
<CommandType>OpenWindow</CommandType>
<Url>~/Apps/MultipleAdd.aspx?ViewName={QueryString:ViewName}</Url>
<Width>500</Width>
<Height>375</Height>
<Resize>False</Resize>
<Scroll>False</Scroll>
<UpdatePanelIds>UpdatePanel1,UpdatePanel2</UpdatePanelIds>
</Command>
CommandParameters cp = new CommandParameters("MC_TimeTracking_MultipleAdd");
CommandManager.RegisterRefreshParentWindowScript(this.Page, cp.ToString());
OpenFrameModalPopup
The OpenFrameModalPopup-type of command can contain these tags:
- Url (string) – URL to page for opening in pop-up window. You can use the same templates as in the Navigate-type command.
- Width (int) – Width in pixels for window (default, 640).
- Height (int) – Height in pixels for window (default, 480).
- Left (int) – Left position for window (displayed in center by default).
- Top (int) – Top position for window (displayed in center by default).
- PopupTitle (string) – Pop-up window title.
- Drag (True/False) – Allow to drag window or not (default False).
- UpdatePanelIds (string) – List of UpdatePanels IDs, which is necessary to update after the window closes.
- EnableHandler (string) – Server handler in format "className, assemblyName", which defines accessibility of the command.
- AutoHeightResize (True/False) – When True, if the pop-up dialog cannot be displayed in the browser, its height is adjusted.Â
OpenFrameModalPopup example
<Command id="MC_ListApp_AddMetaField">
<CommandType>OpenModalPopup</CommandType>
<Url>~/Apps/MetaFieldListEdit.ascx</Url>
<Width>600</Width>
<Height>440</Height>
<Left>20</Left>
<Top>20</Top>
<PopupTitle>{IbnFramework.ListInfo:tAddField}</PopupTitle>
</Command>
The .ascx-control, which should be displayed in dialog, must implement the IModalPopupControl interface:
Implement the IModalPopupControl interface
public partial class QuickAddControl : System.Web.UI.UserControl, IModalPopupControl
{
#region IModalPopupControl Members
public void BindData(string p)
{
}
public string ContainerId
{
get
{
if (ViewState["ContainerId"] != null)
return ViewState["ContainerId"].ToString();
else
return String.Empty;
}
set
{
ViewState["ContainerId"] = value;
}
}
public string ScriptHidePopup
{
get
{
if (ViewState["ScriptHidePopup"] != null)
return ViewState["ScriptHidePopup"].ToString();
else
return String.Empty;
}
set
{
ViewState["ScriptHidePopup"] = value;
}
}
#endregion
}
To update the parent window and close the ModalPopup, run the following code:
CommandParameters cp = new CommandParameters(commandId);
CommandManager.RegisterCloseOpenedFrameScript(this.Page, cp.ToString());
If the parent window contains UpdatePanels, and you need to run a full update for the window when the button id="Btn1" is pressed, run the following code in Page_Load for the .ascx control:
ScriptManager.GetCurrent(this.Page).RegisterPostBackControl(Btn1);
For closingModalPopup:
CancelButton.OnClientClick = CommandManager.GetCloseOpenedFrameScript(this.Page, String.Empty, false, true);
If you need to run another command from ModalPopup:
CommandManager cm = CommandManager.GetCurrent(this.Page);
Dictionary<string, string> prms = new Dictionary<string, string>();
string command = cm.AddCommand(className, viewName, placeName, "MC_TimeTracking_MultipleAdd", prms);
MultipleAddLink.NavigateUrl = String.Format(CultureInfo.InvariantCulture, "javascript:{0};{1}", ScriptHidePopup, command);
Example: UI for IFrame ModalPopup:
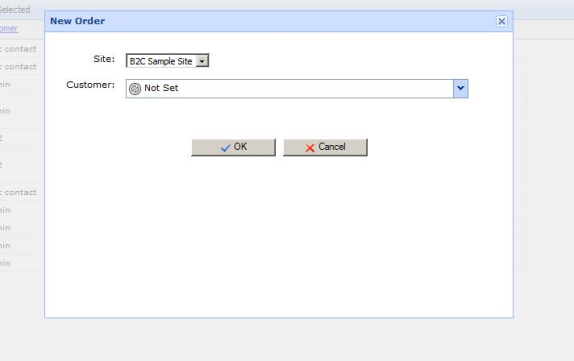
The following steps create a dialog box for a MyPage.aspx custom page.
- Create an .ascx control implementing the IModalPopupControl interface, and name it MyControl.ascx.
- Create an XML file with the name MyPage.xml.
<?xml version="1.0" encoding="utf-8" ?> <View xmlns="http://schemas.mediachase.com/ecf/view"> <ListViewUI> <Commands> <add> <Command id="MyCommand"> <CommandType>OpenModalPopup</CommandType> <Url>~/Apps/MyControl.ascx</Url> <Width>300</Width> <Height>200</Height> <Left>100</Left> <Top>100</Top> <Drag>True</Drag> <PopupTitle>Some Title</PopupTitle> </Command> </add> </Commands> </ListViewUI> </View>
- Run the following code for MyPage to display the dialog box:
CommandManager cm = CommandManager.GetCurrent(this.Page); CommandParameters cp = new CommandParameters("MyCommand"); string command = cm.AddCommand("MyPage ", "", "", cp);
4. After you run the AddCommand method, the variable command contains JavaScript code. If you add the javascript: prefix, you can use the NavigateUrl property for the HyperLink control.
ServerAction
The ServerAction-type command can contain the following tags:
- ConfirmationText (string) – Text in the confirmation dialog box. If this parameter is not defined, it does not display text.
- Handler (string) – Server handler for command in format "className, assemblyName".
- UpdatePanelIds (string) – List of UpdatePanels IDs, which are necessary to update after window close.
- EnableHandler (string) – Server handler in format "className, assemblyName" which defines the accessibility of the command.
Example: ServerAction-type command
<Command id="MC_ListApp_Selected_Delete">
<CommandType>ServerAction</CommandType>
<ConfirmationText>{IbnFramework.Common:DeleteConfirm}</ConfirmationText>
<Handler>Mediachase.Ibn.DeleteSelectedItemsHandler, Mediachase.UI.Web</Handler>
</Command>
The server handler must implement the ICommand interface. The following is an example of the server handler, with actions for selected elements in the grid:
Server handler implementing ICommand interface
public class DeleteHandler : ICommand
{
public void Invoke(object Sender, object Element)
{
if (Element is CommandParameters)
{
CommandParameters cp = (CommandParameters)Element;
string[] selectedElements = MetaGrid.GetCheckedCollection(((CommandManager)Sender).Page,
cp.CommandArguments["GridId"]);
foreach (string elem in selectedElements)
{
string id = elem.Split(new string[] { "::" },
StringSplitOptions.RemoveEmptyEntries)[0];
// Here some actions for elements
// ...
}
}
}
}
The following example shows how to get the primaryKeyId for a grid record:
Example: get primaryKeyId for grid record
CommandParameters cp = (CommandParameters)Element;
if (cp.CommandArguments["primaryKeyId"] == null) throw new ArgumentException();
PrimaryKeyId pk = PrimaryKeyId.Parse(cp.CommandArguments["primaryKeyId"]);
ClientAction
The ClientAction-type command can contain the following tags:
- ClientScript – String containing JavaScript, use a template like {TemplateType:KeyName} (see the Navigate section for the description).
- EnableHandler (string) – Server handler in the format "className, assemblyName" defining accessibility of the command.
Updated 3 months ago