Build an app
Begin building a custom app using the Optimizely Connect Platform (OCP) developer platform.
Beta
Optimizely Connect Platform (OCP) for Optimizely is in beta and currently only works with Optimizely Graph in Optimizely Content Management System (CMS) (SaaS) and CMS (PaaS).
The Optimizely Connect Platform (OCP) app development lifecycle begins in the OCP command-line interface (CLI), where you register and scaffold the app and then prepare your development environment.
From there, you define the app's data schema in your development environment, which involves creating and defining any custom objects, fields, and relationships necessary to store the data your app will handle.
After you do that, you can use the OCP CLI to validate your app configuration, prepare your app for publishing, then finally publish and install the app to your OCP account. At this point, your app displays in the OCP App Directory, and you can view the data schema it created in the Objects & Fields page of the OCP user interface (UI).
Now you must write the code for your app, which involves defining the app settings form that anyone who installs the app will use to connect and configure the app per their needs.
You can iterate on your app build and continue to publish versions of your app to the OCP App Directory so you can see how the settings form displays and functions, and you can test the app to ensure it works as expected.
Register and scaffold the app
- Register your app and complete the prompts to give your app a unique app ID and name, define OCP as the target product, and mark the app as shareable with other developers in your organization.
Important
Ensure you choose
HUB
as the target product for the app. Your app ID must be in snake case, where each word is lowercase and all spaces are replaced with an underscore.
This command returns the following prompts that you must complete:ocp app register
The app id to reserve: YOUR_APP_ID The display name of the app: YOUR APP DISPLAY NAME Target product for the app: HUB Keep this app private and not share it with other developers in your organization? No
- Scaffold the app and initialize the App Directory. The
Basic Sample
template contains example code to get you started (including functions and jobs), and theEmpty Project
template is a basic app without example code. If you use theBasic Sample
template, you must replace the event examples with objects you intend to update in your OCP account. It also includessrc/schema/customers.yml
which you must replace with the schema definition of the object you want to update, likesrc/schema/commerce_products.yml
. See App versioning for help with how to version your app.Important
Do not select any of the following categories:
- Loyalty & Rewards
- Reviews & Ratings
- Commerce Platform
- Content Management
- Offers
This command returns the following prompts that you must complete:ocp app init
Name of your app (e.g., My App): YOUR APP DISPLAY NAME ID of your app [ocp_quickstart]: YOUR_APP_ID Version [1.0.0-dev.1]: 1.0.0-dev.1 App Summary (brief): YOUR APP SUMMARY Support URL: YOUR SUPPORT URL Contact email address: YOUR SUPPORT EMAIL Select the category for the app: YOUR APP CATEGORY Select a template project: Empty Project
- Open it in your preferred editor and run
git init
to prepare the environment.
Define the schema
In the src/schema
folder, you can define the data tables, fields, and relationships needed for your app. All .yml
files within refer to objects in OCP. These represent the data that users can select and sync to their Optimizely products in the OCP user interface (UI). When a user installs your app, what you define here is added to their OCP data schema.
Important
You must prefix object names with your app ID, and prefix the object display names with your app display name. For example, if your app ID is
my_app
and the display name isMy App
, prefix object names withmy_app
, likemy_app_customer_service_tickets
, and prefix object display names withMy App
, likeMy App Customer Service Tickets
.You do not have to do this for fields within an object that your app creates.
-
Define any custom objects your app needs. For example, if you want to create a Commerce Products object, you need to create an
src/schema/commerce_products.yml
file, which might look like the following:name: my_app_commerce_products # internal name for the data table aka ‘object’ display_name: My App Commerce Products # the name that will show in the OCP UI for the object fields: - name: commerce_product_id # internal name of field. This is an example of creating the primary key for the object type: string # field type, options are: number, boolean, string, timestamp display_name: CS Ticket ID # name that will show in the OCP UI for the field description: Unique identifier of the ticket # description will appear on hover of the field in the OCP UI primary: true # denotes the field is the primary key for the object - name: name # internal name of field type: string # field type display_name: Name # name that will show in the OCP UI for the field description: The name of the product # description will appear on hover of the field in the OCP UI - name: price type: number display_name: Price description: The price of the product
-
Define a relationship between objects. You can define relationships between objects should your app need to do so based on the data model of the external system you are connecting to. Here is an example of a
product_category
object containing a relation to the previously definedmy_app_commerce_products
object. Define theproduct_category
object in ansrc/schema/my_app_commerce_product_categories.yml
file, which might look like the following:name: my_app_commerce_product_categories display_name: My App Commerce Product Categories fields: - name: commerce_product_category_id display_name: Commerce Product Category ID type: string primary: true - name: commerce_product_id display_name: Commerce Product ID type: string - name: category_name display_name: Category Name type: string relations: - name: product display_name: Product child_object: my_app_commerce_products join_fields: - parent: commerce_product_id child: commerce_product_id
Validate, prepare, publish, and install the app
-
Validate your app configuration by running
ocp app validate
in the CLI. -
Run
ocp app prepare
to prepare your app for publishing. -
Publish the app to your OCP App Directory by running
ocp directory publish [email protected]
. See App versioning for help with how to version your app. -
Run
ocp directory install [email protected] TRACKER_ID
to install the app to your OCP account. ReplaceTRACKER_ID
with your OCP account Tracker ID, which you can find in Data Setup > Integrations in the OCP user interface (UI). For example,ocp directory install [email protected] Z6jCndly_E2WzpvDkcKBKA
.At this point, you can view your app listing in your OCP app directory (only you can view it). The app does not run any code yet, but you can view the app schema in Data Setup > Objects & Fields in the OCP UI.
Define the app settings form
OCP apps include a settings tab which is accessible after a user installs the app. The settings tab is where you define how you want users to connect and use your app.
OCP apps support several types of authentication, including OAuth, tokens, username and password, and API keys.
The following image shows an example settings tab for an OCP app.
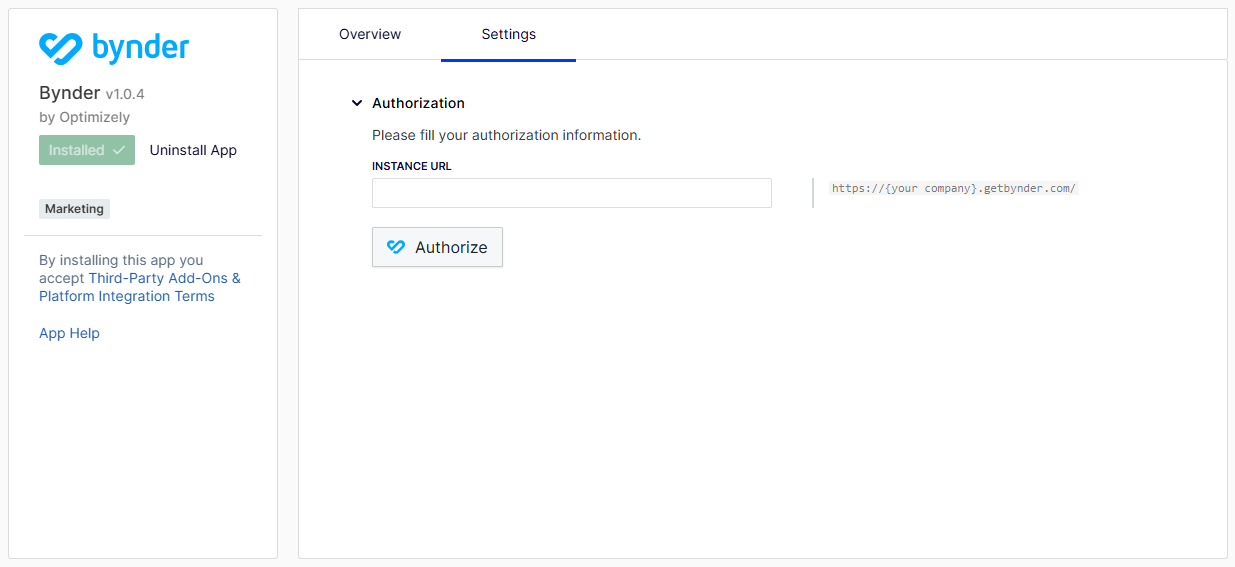
Manage your app settings in the src/forms/settings.yml
file, which is already created as a placeholder form after you complete the previous steps. Your settings form consists of the following:
-
Sections – You can have multiple sections in your form where the first section is expanded by default, and users can expand subsequent sections. You can organize sections in the
forms/
folder. The following is an example of a settings form with two sections, where the second section will only show after a successful login in the first section.sections: - key: login label: Login properties: - successful elements: - ... - key: lists label: Import Lists visible: key: login.successful equals: true elements: - ...
-
Form elements – The different UI elements that users interact with in your settings form. See Form elements for a list of form elements that you can add to your settings area.
Updated 16 days ago